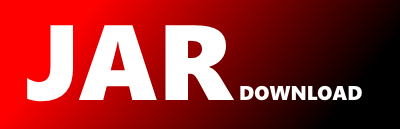
com.undefinedlabs.scope.rules.proto.HelloServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scope-rules-testing Show documentation
Show all versions of scope-rules-testing Show documentation
Scope is a APM for tests to give engineering teams unprecedented visibility into their CI process to quickly identify, troubleshoot and fix failed builds.
This artifact contains classes that supports Testing in the other Scope Rules modules.
package com.undefinedlabs.scope.rules.proto;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.*;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.*;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.4.0)",
comments = "Source: HelloService.proto")
public final class HelloServiceGrpc {
private HelloServiceGrpc() {}
public static final String SERVICE_NAME = "com.undefinedlabs.scope.rules.proto.HelloService";
// Static method descriptors that strictly reflect the proto.
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_SUCCESS_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitarySuccessSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitarySuccessFutureDirect"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitarySuccessFutureCallback"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_SUCCESS_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitarySuccessAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_FAILURE_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitaryFailureSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_FAILURE_FUTURE_DIRECT =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitaryFailureFutureDirect"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitaryFailureFutureCallback"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_UNITARY_FAILURE_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloUnitaryFailureAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_CLIENT_STREAMING_SUCCESS_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloClientStreamingSuccessSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloClientStreamingSuccessAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_CLIENT_STREAMING_FAILURE_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloClientStreamingFailureSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_CLIENT_STREAMING_FAILURE_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloClientStreamingFailureAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_SERVER_STREAMING_SUCCESS_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloServerStreamingSuccessSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_SERVER_STREAMING_SUCCESS_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloServerStreamingSuccessAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_SERVER_STREAM_FAILURE_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloServerStreamFailureSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_SERVER_STREAM_FAILURE_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloServerStreamFailureAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloBiDirStreamingSuccessSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloBiDirStreamingSuccessAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_BI_DIR_STREAMING_FAILURE_SYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloBiDirStreamingFailureSync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(
"com.undefinedlabs.scope.rules.proto.HelloService", "helloBiDirStreamingFailureAsync"))
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.undefinedlabs.scope.rules.proto.HelloResponse.getDefaultInstance()))
.build();
/**
* Creates a new async stub that supports all call types for the service
*/
public static HelloServiceStub newStub(io.grpc.Channel channel) {
return new HelloServiceStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static HelloServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new HelloServiceBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static HelloServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
return new HelloServiceFutureStub(channel);
}
/**
*/
public static abstract class HelloServiceImplBase implements io.grpc.BindableService {
/**
*/
public void helloUnitarySuccessSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_SUCCESS_SYNC, responseObserver);
}
/**
*/
public void helloUnitarySuccessFutureDirect(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT, responseObserver);
}
/**
*/
public void helloUnitarySuccessFutureCallback(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK, responseObserver);
}
/**
*/
public void helloUnitarySuccessAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_SUCCESS_ASYNC, responseObserver);
}
/**
*/
public void helloUnitaryFailureSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_FAILURE_SYNC, responseObserver);
}
/**
*/
public void helloUnitaryFailureFutureDirect(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_FAILURE_FUTURE_DIRECT, responseObserver);
}
/**
*/
public void helloUnitaryFailureFutureCallback(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK, responseObserver);
}
/**
*/
public void helloUnitaryFailureAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_UNITARY_FAILURE_ASYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingSuccessSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_CLIENT_STREAMING_SUCCESS_SYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingSuccessAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingFailureSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_CLIENT_STREAMING_FAILURE_SYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingFailureAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_CLIENT_STREAMING_FAILURE_ASYNC, responseObserver);
}
/**
*/
public void helloServerStreamingSuccessSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_SERVER_STREAMING_SUCCESS_SYNC, responseObserver);
}
/**
*/
public void helloServerStreamingSuccessAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_SERVER_STREAMING_SUCCESS_ASYNC, responseObserver);
}
/**
*/
public void helloServerStreamFailureSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_SERVER_STREAM_FAILURE_SYNC, responseObserver);
}
/**
*/
public void helloServerStreamFailureAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_HELLO_SERVER_STREAM_FAILURE_ASYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingSuccessSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingSuccessAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingFailureSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_BI_DIR_STREAMING_FAILURE_SYNC, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingFailureAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(METHOD_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC, responseObserver);
}
@Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
METHOD_HELLO_UNITARY_SUCCESS_SYNC,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_SUCCESS_SYNC)))
.addMethod(
METHOD_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT)))
.addMethod(
METHOD_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK)))
.addMethod(
METHOD_HELLO_UNITARY_SUCCESS_ASYNC,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_SUCCESS_ASYNC)))
.addMethod(
METHOD_HELLO_UNITARY_FAILURE_SYNC,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_FAILURE_SYNC)))
.addMethod(
METHOD_HELLO_UNITARY_FAILURE_FUTURE_DIRECT,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_FAILURE_FUTURE_DIRECT)))
.addMethod(
METHOD_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK)))
.addMethod(
METHOD_HELLO_UNITARY_FAILURE_ASYNC,
asyncUnaryCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_UNITARY_FAILURE_ASYNC)))
.addMethod(
METHOD_HELLO_CLIENT_STREAMING_SUCCESS_SYNC,
asyncClientStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_CLIENT_STREAMING_SUCCESS_SYNC)))
.addMethod(
METHOD_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC,
asyncClientStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC)))
.addMethod(
METHOD_HELLO_CLIENT_STREAMING_FAILURE_SYNC,
asyncClientStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_CLIENT_STREAMING_FAILURE_SYNC)))
.addMethod(
METHOD_HELLO_CLIENT_STREAMING_FAILURE_ASYNC,
asyncClientStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_CLIENT_STREAMING_FAILURE_ASYNC)))
.addMethod(
METHOD_HELLO_SERVER_STREAMING_SUCCESS_SYNC,
asyncServerStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_SERVER_STREAMING_SUCCESS_SYNC)))
.addMethod(
METHOD_HELLO_SERVER_STREAMING_SUCCESS_ASYNC,
asyncServerStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_SERVER_STREAMING_SUCCESS_ASYNC)))
.addMethod(
METHOD_HELLO_SERVER_STREAM_FAILURE_SYNC,
asyncServerStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_SERVER_STREAM_FAILURE_SYNC)))
.addMethod(
METHOD_HELLO_SERVER_STREAM_FAILURE_ASYNC,
asyncServerStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_SERVER_STREAM_FAILURE_ASYNC)))
.addMethod(
METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC,
asyncBidiStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC)))
.addMethod(
METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC,
asyncBidiStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC)))
.addMethod(
METHOD_HELLO_BI_DIR_STREAMING_FAILURE_SYNC,
asyncBidiStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_BI_DIR_STREAMING_FAILURE_SYNC)))
.addMethod(
METHOD_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC,
asyncBidiStreamingCall(
new MethodHandlers<
com.undefinedlabs.scope.rules.proto.HelloRequest,
com.undefinedlabs.scope.rules.proto.HelloResponse>(
this, METHODID_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC)))
.build();
}
}
/**
*/
public static final class HelloServiceStub extends io.grpc.stub.AbstractStub {
private HelloServiceStub(io.grpc.Channel channel) {
super(channel);
}
private HelloServiceStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@Override
protected HelloServiceStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new HelloServiceStub(channel, callOptions);
}
/**
*/
public void helloUnitarySuccessSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_SYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloUnitarySuccessFutureDirect(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloUnitarySuccessFutureCallback(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloUnitarySuccessAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_ASYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloUnitaryFailureSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_SYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloUnitaryFailureFutureDirect(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_FUTURE_DIRECT, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloUnitaryFailureFutureCallback(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloUnitaryFailureAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_ASYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingSuccessSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncClientStreamingCall(
getChannel().newCall(METHOD_HELLO_CLIENT_STREAMING_SUCCESS_SYNC, getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingSuccessAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncClientStreamingCall(
getChannel().newCall(METHOD_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC, getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingFailureSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncClientStreamingCall(
getChannel().newCall(METHOD_HELLO_CLIENT_STREAMING_FAILURE_SYNC, getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloClientStreamingFailureAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncClientStreamingCall(
getChannel().newCall(METHOD_HELLO_CLIENT_STREAMING_FAILURE_ASYNC, getCallOptions()), responseObserver);
}
/**
*/
public void helloServerStreamingSuccessSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_HELLO_SERVER_STREAMING_SUCCESS_SYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloServerStreamingSuccessAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_HELLO_SERVER_STREAMING_SUCCESS_ASYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloServerStreamFailureSync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_HELLO_SERVER_STREAM_FAILURE_SYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public void helloServerStreamFailureAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_HELLO_SERVER_STREAM_FAILURE_ASYNC, getCallOptions()), request, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingSuccessSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncBidiStreamingCall(
getChannel().newCall(METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC, getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingSuccessAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncBidiStreamingCall(
getChannel().newCall(METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC, getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingFailureSync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncBidiStreamingCall(
getChannel().newCall(METHOD_HELLO_BI_DIR_STREAMING_FAILURE_SYNC, getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver helloBiDirStreamingFailureAsync(
io.grpc.stub.StreamObserver responseObserver) {
return asyncBidiStreamingCall(
getChannel().newCall(METHOD_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC, getCallOptions()), responseObserver);
}
}
/**
*/
public static final class HelloServiceBlockingStub extends io.grpc.stub.AbstractStub {
private HelloServiceBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private HelloServiceBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@Override
protected HelloServiceBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new HelloServiceBlockingStub(channel, callOptions);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitarySuccessSync(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_SUCCESS_SYNC, getCallOptions(), request);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitarySuccessFutureDirect(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT, getCallOptions(), request);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitarySuccessFutureCallback(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK, getCallOptions(), request);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitarySuccessAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_SUCCESS_ASYNC, getCallOptions(), request);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitaryFailureSync(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_FAILURE_SYNC, getCallOptions(), request);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitaryFailureFutureDirect(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_FAILURE_FUTURE_DIRECT, getCallOptions(), request);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitaryFailureFutureCallback(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK, getCallOptions(), request);
}
/**
*/
public com.undefinedlabs.scope.rules.proto.HelloResponse helloUnitaryFailureAsync(com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_HELLO_UNITARY_FAILURE_ASYNC, getCallOptions(), request);
}
/**
*/
public java.util.Iterator helloServerStreamingSuccessSync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_HELLO_SERVER_STREAMING_SUCCESS_SYNC, getCallOptions(), request);
}
/**
*/
public java.util.Iterator helloServerStreamingSuccessAsync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_HELLO_SERVER_STREAMING_SUCCESS_ASYNC, getCallOptions(), request);
}
/**
*/
public java.util.Iterator helloServerStreamFailureSync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_HELLO_SERVER_STREAM_FAILURE_SYNC, getCallOptions(), request);
}
/**
*/
public java.util.Iterator helloServerStreamFailureAsync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_HELLO_SERVER_STREAM_FAILURE_ASYNC, getCallOptions(), request);
}
}
/**
*/
public static final class HelloServiceFutureStub extends io.grpc.stub.AbstractStub {
private HelloServiceFutureStub(io.grpc.Channel channel) {
super(channel);
}
private HelloServiceFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@Override
protected HelloServiceFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new HelloServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitarySuccessSync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_SYNC, getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitarySuccessFutureDirect(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT, getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitarySuccessFutureCallback(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK, getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitarySuccessAsync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_SUCCESS_ASYNC, getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitaryFailureSync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_SYNC, getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitaryFailureFutureDirect(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_FUTURE_DIRECT, getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitaryFailureFutureCallback(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK, getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture helloUnitaryFailureAsync(
com.undefinedlabs.scope.rules.proto.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_HELLO_UNITARY_FAILURE_ASYNC, getCallOptions()), request);
}
}
private static final int METHODID_HELLO_UNITARY_SUCCESS_SYNC = 0;
private static final int METHODID_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT = 1;
private static final int METHODID_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK = 2;
private static final int METHODID_HELLO_UNITARY_SUCCESS_ASYNC = 3;
private static final int METHODID_HELLO_UNITARY_FAILURE_SYNC = 4;
private static final int METHODID_HELLO_UNITARY_FAILURE_FUTURE_DIRECT = 5;
private static final int METHODID_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK = 6;
private static final int METHODID_HELLO_UNITARY_FAILURE_ASYNC = 7;
private static final int METHODID_HELLO_SERVER_STREAMING_SUCCESS_SYNC = 8;
private static final int METHODID_HELLO_SERVER_STREAMING_SUCCESS_ASYNC = 9;
private static final int METHODID_HELLO_SERVER_STREAM_FAILURE_SYNC = 10;
private static final int METHODID_HELLO_SERVER_STREAM_FAILURE_ASYNC = 11;
private static final int METHODID_HELLO_CLIENT_STREAMING_SUCCESS_SYNC = 12;
private static final int METHODID_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC = 13;
private static final int METHODID_HELLO_CLIENT_STREAMING_FAILURE_SYNC = 14;
private static final int METHODID_HELLO_CLIENT_STREAMING_FAILURE_ASYNC = 15;
private static final int METHODID_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC = 16;
private static final int METHODID_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC = 17;
private static final int METHODID_HELLO_BI_DIR_STREAMING_FAILURE_SYNC = 18;
private static final int METHODID_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC = 19;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final HelloServiceImplBase serviceImpl;
private final int methodId;
MethodHandlers(HelloServiceImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@Override
@SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_HELLO_UNITARY_SUCCESS_SYNC:
serviceImpl.helloUnitarySuccessSync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT:
serviceImpl.helloUnitarySuccessFutureDirect((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK:
serviceImpl.helloUnitarySuccessFutureCallback((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_UNITARY_SUCCESS_ASYNC:
serviceImpl.helloUnitarySuccessAsync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_UNITARY_FAILURE_SYNC:
serviceImpl.helloUnitaryFailureSync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_UNITARY_FAILURE_FUTURE_DIRECT:
serviceImpl.helloUnitaryFailureFutureDirect((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK:
serviceImpl.helloUnitaryFailureFutureCallback((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_UNITARY_FAILURE_ASYNC:
serviceImpl.helloUnitaryFailureAsync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_SERVER_STREAMING_SUCCESS_SYNC:
serviceImpl.helloServerStreamingSuccessSync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_SERVER_STREAMING_SUCCESS_ASYNC:
serviceImpl.helloServerStreamingSuccessAsync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_SERVER_STREAM_FAILURE_SYNC:
serviceImpl.helloServerStreamFailureSync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_HELLO_SERVER_STREAM_FAILURE_ASYNC:
serviceImpl.helloServerStreamFailureAsync((com.undefinedlabs.scope.rules.proto.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@Override
@SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_HELLO_CLIENT_STREAMING_SUCCESS_SYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloClientStreamingSuccessSync(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloClientStreamingSuccessAsync(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_HELLO_CLIENT_STREAMING_FAILURE_SYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloClientStreamingFailureSync(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_HELLO_CLIENT_STREAMING_FAILURE_ASYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloClientStreamingFailureAsync(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloBiDirStreamingSuccessSync(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloBiDirStreamingSuccessAsync(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_HELLO_BI_DIR_STREAMING_FAILURE_SYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloBiDirStreamingFailureSync(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC:
return (io.grpc.stub.StreamObserver) serviceImpl.helloBiDirStreamingFailureAsync(
(io.grpc.stub.StreamObserver) responseObserver);
default:
throw new AssertionError();
}
}
}
private static final class HelloServiceDescriptorSupplier implements io.grpc.protobuf.ProtoFileDescriptorSupplier {
@Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.undefinedlabs.scope.rules.proto.HelloServiceOuterClass.getDescriptor();
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (HelloServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new HelloServiceDescriptorSupplier())
.addMethod(METHOD_HELLO_UNITARY_SUCCESS_SYNC)
.addMethod(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_DIRECT)
.addMethod(METHOD_HELLO_UNITARY_SUCCESS_FUTURE_CALLBACK)
.addMethod(METHOD_HELLO_UNITARY_SUCCESS_ASYNC)
.addMethod(METHOD_HELLO_UNITARY_FAILURE_SYNC)
.addMethod(METHOD_HELLO_UNITARY_FAILURE_FUTURE_DIRECT)
.addMethod(METHOD_HELLO_UNITARY_FAILURE_FUTURE_CALLBACK)
.addMethod(METHOD_HELLO_UNITARY_FAILURE_ASYNC)
.addMethod(METHOD_HELLO_CLIENT_STREAMING_SUCCESS_SYNC)
.addMethod(METHOD_HELLO_CLIENT_STREAMING_SUCCESS_ASYNC)
.addMethod(METHOD_HELLO_CLIENT_STREAMING_FAILURE_SYNC)
.addMethod(METHOD_HELLO_CLIENT_STREAMING_FAILURE_ASYNC)
.addMethod(METHOD_HELLO_SERVER_STREAMING_SUCCESS_SYNC)
.addMethod(METHOD_HELLO_SERVER_STREAMING_SUCCESS_ASYNC)
.addMethod(METHOD_HELLO_SERVER_STREAM_FAILURE_SYNC)
.addMethod(METHOD_HELLO_SERVER_STREAM_FAILURE_ASYNC)
.addMethod(METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_SYNC)
.addMethod(METHOD_HELLO_BI_DIR_STREAMING_SUCCESS_ASYNC)
.addMethod(METHOD_HELLO_BI_DIR_STREAMING_FAILURE_SYNC)
.addMethod(METHOD_HELLO_BI_DIR_STREAMING_FAILURE_ASYNC)
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy