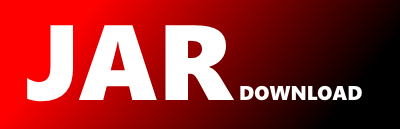
com.univapay.sdk.utils.functions.UnivapayFunctions Maven / Gradle / Ivy
package com.univapay.sdk.utils.functions;
import com.univapay.sdk.builders.Cancelable;
import com.univapay.sdk.builders.Request;
import com.univapay.sdk.models.errors.TooManyRequestsException;
import com.univapay.sdk.models.errors.UnivapayException;
import com.univapay.sdk.utils.Sleeper;
import com.univapay.sdk.utils.UnivapayCallback;
import java.io.IOException;
public class UnivapayFunctions {
public static EndoFunction identity() {
return new EndoFunction() {
@Override
public T apply(T t) throws Exception {
return t;
}
};
}
private static boolean shouldHandle(ErrorHandler handler, Throwable t) {
return handler != null && handler.getShouldHandleError().test(t);
}
public static , M2, T2 extends Request> Request compose(
final T1 firstRequest,
final Function onSuccess,
final ErrorHandler errorHandler) {
return new Request() {
@Override
public Cancelable dispatch(final UnivapayCallback callback) {
return firstRequest.dispatch(
new UnivapayCallback() {
@Override
public void getResponse(M1 response) {
onSuccess.apply(response).dispatch(callback);
}
@Override
public void getFailure(Throwable error) {
if (shouldHandle(errorHandler, error)) {
errorHandler.getHandler().apply(error).dispatch(callback);
} else {
callback.getFailure(error);
}
}
});
}
@Override
public M2 dispatch() throws IOException, UnivapayException, TooManyRequestsException {
try {
M1 response = firstRequest.dispatch();
return onSuccess.apply(response).dispatch();
} catch (IOException | UnivapayException error) {
if (shouldHandle(errorHandler, error)) {
return errorHandler.getHandler().apply(error).dispatch();
} else {
throw error;
}
}
}
@Override
public M2 dispatch(int maxRetry, Sleeper sleeper)
throws IOException, UnivapayException, TooManyRequestsException, InterruptedException {
try {
M1 response = firstRequest.dispatch(maxRetry, sleeper);
return onSuccess.apply(response).dispatch(maxRetry, sleeper);
} catch (IOException | UnivapayException error) {
if (shouldHandle(errorHandler, error)) {
return errorHandler.getHandler().apply(error).dispatch(maxRetry, sleeper);
} else {
throw error;
}
}
}
};
}
public static > Request retry(
final T1 request, final ErrorHandler errorHandler) {
return new Request() {
@Override
public Cancelable dispatch(final UnivapayCallback callback) {
return request.dispatch(
new UnivapayCallback() {
@Override
public void getResponse(M1 response) {
callback.getResponse(response);
}
@Override
public void getFailure(Throwable error) {
if (shouldHandle(errorHandler, error)) {
errorHandler.getHandler().apply(error).dispatch(callback);
} else {
callback.getFailure(error);
}
}
});
}
@Override
public M1 dispatch() throws IOException, UnivapayException, TooManyRequestsException {
try {
return request.dispatch();
} catch (IOException | UnivapayException error) {
if (shouldHandle(errorHandler, error)) {
return errorHandler.getHandler().apply(error).dispatch();
} else {
throw error;
}
}
}
@Override
public M1 dispatch(int maxRetry, Sleeper sleeper)
throws IOException, UnivapayException, TooManyRequestsException, InterruptedException {
try {
return request.dispatch(maxRetry, sleeper);
} catch (IOException | UnivapayException error) {
if (shouldHandle(errorHandler, error)) {
return errorHandler.getHandler().apply(error).dispatch(maxRetry, sleeper);
} else {
throw error;
}
}
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy