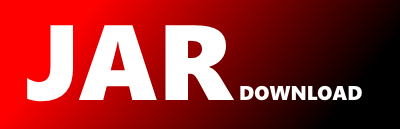
com.univocity.api.data.DatasetFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of univocity-api Show documentation
Show all versions of univocity-api Show documentation
uniVocity Data Integration's Public API
/*******************************************************************************
* Copyright (c) 2014 uniVocity Software Pty Ltd. All rights reserved.
* This file is subject to the terms and conditions defined in file
* 'LICENSE.txt', which is part of this source code package.
******************************************************************************/
package com.univocity.api.data;
import java.util.*;
import com.univocity.api.*;
import com.univocity.api.engine.*;
/**
* A factory for creating {@link Dataset} instances backed by collections.
* To get a DatasetFactory
from uniVocity, use {@link Univocity#datasetFactory()}.
*
* @author uniVocity Software Pty Ltd - [email protected]
*
*/
public interface DatasetFactory {
/**
* Creates a new modifiable dataset backed by a collection of rows.
* @param rows the rows in the dataset
* @param identifier the name of the field that identifies rows in this dataset
* @param fieldNames the sequence of field names of each record in this data set.
* @return a new modifiable dataset.
*/
public ModifiableDataset newDataset(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy