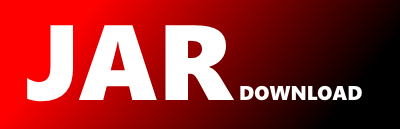
com.univocity.api.data.DatasetProducer Maven / Gradle / Ivy
Show all versions of univocity-api Show documentation
/*******************************************************************************
* Copyright (c) 2014 uniVocity Software Pty Ltd. All rights reserved.
* This file is subject to the terms and conditions defined in file
* 'LICENSE.txt', which is part of this source code package.
******************************************************************************/
package com.univocity.api.data;
import java.util.*;
import com.univocity.api.common.*;
import com.univocity.api.config.builders.*;
import com.univocity.api.engine.*;
/**
* A producer of datasets. Used to process values of a data entity and store the results in one or more datasets.
* Use {@link DataIntegrationEngine#addDatasetProducer(EngineScope, DatasetProducer)} to configure your dataset producer.
* The datasets generated can be used by different entity mappings and their values are bound to a scope.
* The datasets will only be regenerated by the producer if their scope was destroyed.
*
* @see DataIntegrationEngine
* @see DatasetProducerSetup
*
* @author uniVocity Software Pty Ltd - [email protected]
*
*/
public abstract class DatasetProducer {
private final String[] datasetNames;
private String[] inputFields;
private final Map inputFieldPositions = new HashMap();
public DatasetProducer(String... datasetNames) {
Args.notEmpty(datasetNames, "Names of the datasets produced by this DatasetProducer");
for (String name : datasetNames) {
Args.notBlank(name, "Dataset name");
}
this.datasetNames = datasetNames;
}
/**
* used by uniVocity to inform this DatasetProducer
a reading process has been started on the data entity it is bound to.
* @param fieldNames the field names of the records in an entity being loaded by uniVocity
*/
public final void processStarted(String[] fieldNames) {
inputFields = fieldNames;
int position = 0;
for (String field : fieldNames) {
inputFieldPositions.put(field.trim().toLowerCase(), position++);
}
processStarted();
}
/**
* Returns the position of a given field in each record passed by uniVocity into {@link #processNext(Object[])}.
* @param fieldName the name of the field to whose position in the input should be returned
* @return the position of the given field name in the input rows sent to this dataset producer.
*/
protected final int getFieldPosition(String fieldName) {
Args.notBlank(fieldName, "Field name");
Integer position = inputFieldPositions.get(fieldName.trim().toLowerCase());
if (position == null) {
throw new IllegalArgumentException("Invalid field name '" + fieldName + "'. Available field names are: " + Arrays.toString(inputFields));
}
return position;
}
protected final String[] getInputFields() {
return inputFields.clone();
}
/**
* invoked after {@link #processStarted(String[])} is called by uniVocity and the positions of each field name in the input were populated.
* Override this method to get the indexes of fields that will be read in each input row sent by uniVocity to {@link #processNext(Object[])}.
*/
public void processStarted() {
}
/**
* used by uniVocity to notify this DatasetProducer
that all records from the data entity have bean read.
*/
public void processEnded() {
}
/**
* used by uniVocity to provide the next record extracted from the entity
* @param row the values of the entity record
*/
public abstract void processNext(Object[] row);
/**
* Returns one of the datasets produced by this DatasetProducer
as a result of processing records of a data entity.
* @param name the dataset name declared when configuring this DatasetProducer
* @return the dataset generated after processing records of a data entity.
*/
public abstract Dataset getDataset(String name);
/**
* Returns the names of the datasets produced by this object.
* @return the names of produced datasets
*/
public final String[] getDatasetNames() {
return datasetNames.clone();
}
}