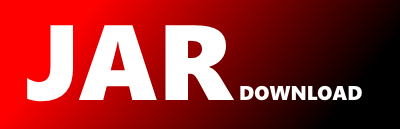
com.univocity.api.engine.RowReader Maven / Gradle / Ivy
Show all versions of univocity-api Show documentation
/*******************************************************************************
* Copyright (c) 2013 uniVocity Software Pty Ltd. All rights reserved.
* This file is subject to the terms and conditions defined in file
* 'LICENSE.txt', which is part of this source code package.
******************************************************************************/
package com.univocity.api.engine;
import com.univocity.api.config.builders.*;
/**
* A RowReader
provides access to rows being processed during the mapping between two data entities.
* Use {@link EntityMapping} to provide row readers for accessing data while reading from a source entity and before or after writing to a destination entity.
*
*
With a RowReader
, the user has easy access to the data:
*
* - loaded from an input
* - transformed and ready to be written to an output
* - written to the output
*
*
* The user can read and modify the contents of each row, and easily perform common tasks such as data monitoring, logging and statistics.
*
The {@link #initialize(RowMappingContext)} and {@link #cleanup(RowMappingContext)} methods can be overridden to allow initialization before
* and after the RowReader
receives rows for processing.
*
* A {@link RowMappingContext} object is available to allow greater control and provide information about the process in execution.
*
Functions, variables and other entities used by the {@link DataIntegrationEngine} are also available.
*
*
* @see EntityMapping
* @see RowMappingContext
*
* @author uniVocity Software Pty Ltd - [email protected]
*
*/
public abstract class RowReader {
/**
* Gives the {@code RowReader} a chance to perform any initialization it requires before the first call to {@link #processRow(Object[], Object[], RowMappingContext)} is made.
*
Note: this method is only invoked by uniVocity if there are rows in the input entity.
* @param context the contextual information and controls available to the user during the execution of a data mapping process.
*/
public void initialize(RowMappingContext context) {
}
/**
* Processes a row extracted from the input.
* @param inputRow the original data in a record read from the input data entity
* @param outputRow the transformed data. The value of this parameter varies according to where the RowReader
is applied in the {@link EntityMapping}:
*
* - while reading from input
{@link EntityMapping#addInputRowReader(RowReader)}
:
*
outputRow will be null.
*
* - before writing to the output
{@link EntityMapping#addOutputRowReader(RowReader)}
:
*
outputRow will contain all values transformed from the input, with values for references fully populated and
* ready to be written to the destination entity.
*
* - after writing to the output
{@link EntityMapping#addPersistedRowReader(RowReader)}
:
*
outputRow will contain all rows actually written to the destination entity. Generated values, if any, will be available in the outputRow.
*
*
* @param context the contextual information and controls available to the user during the execution of a data mapping process.
*/
public abstract void processRow(Object[] inputRow, Object[] outputRow, RowMappingContext context);
/**
* Cleans up the {@code RowReader} after the reading process.
* Note: this method is always invoked by uniVocity even if the input entity is empty or in case of exceptions.
* @param context the contextual information and controls available to the user after the execution of a data mapping process.
*/
public void cleanup(RowMappingContext context) {
}
}