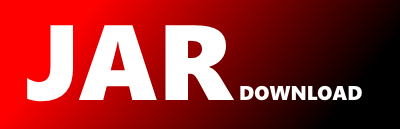
com.univocity.api.entity.jdbc.IdentifierEscaper Maven / Gradle / Ivy
Show all versions of univocity-api Show documentation
/*******************************************************************************
* Copyright (c) 2013 uniVocity Software Pty Ltd. All rights reserved.
* This file is subject to the terms and conditions defined in file
* 'LICENSE.txt', which is part of this source code package.
******************************************************************************/
package com.univocity.api.entity.jdbc;
/**
* A class responsible for escaping table and column names that can be reserved words in SQL statements.
*
* For example, a column named max must be escaped so it doesn't conflict with the max function provided by most (if not all) databases.
*
Additionally a table might have columns whose names contain white spaces such as "purchase date", or even repeated column names in different case: "Max", "mAx".
*
For these cases, most databases require enclosing the column name within quotes. A valid select statement will then be:
*
SELECT "Max", "mAx, "purchase date" FROM table
*
*
Before producing an SQL statement, uniVocity will identify whether a table or column name requires escaping.
* If it is required, the {@link #escape(String)} method will be called to obtain a column name.
*
*
uniVocity will escape most common reserved words used by different database vendors, but you might want to provide additional identifiers.
*
Use {@link JdbcDataStoreConfiguration#addReservedWordsToEscape(String...)} to provide any additional reserved words to be escaped in your JDBC data store.
*
*
If {@link #alwaysEscape()} returns {@code true}, then the SQL statements produced by uniVocity for this JDBC data store will always escape column and table names.
*
* @see JdbcDataStoreConfiguration
* @see SqlProducer
*
* @author uniVocity Software Pty Ltd - [email protected]
*
*/
public abstract class IdentifierEscaper {
/**
* Escapes identifier names to avoid producing SQL statements with conflicting names.
* @param identifier The table or column name that must be escaped
* @return the escaped version of the identifier
*/
public abstract String escape(String identifier);
/**
* Should uniVocity always escape all identifiers in SQL statements?
*
* @return {@code true} if all identifiers must be escaped by default, otherwise {@code false}.
*/
public abstract boolean alwaysEscape();
}