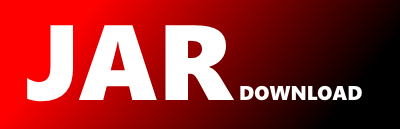
com.univocity.api.entity.text.fixed.FixedWidthFormat Maven / Gradle / Ivy
Show all versions of univocity-api Show documentation
/*******************************************************************************
* Copyright (c) 2014 uniVocity Software Pty Ltd. All rights reserved.
* This file is subject to the terms and conditions defined in file
* 'LICENSE.txt', which is part of this source code package.
******************************************************************************/
package com.univocity.api.entity.text.fixed;
import com.univocity.api.entity.*;
import com.univocity.api.entity.text.*;
/**
* The fixed-width format configuration class. Used by fixed-width data entities in {@link FixedWidthEntityConfiguration}.
* It provides the following configuration options (in addition to the ones in {@link TextFormat}):
*
*
* - padding: the character used for filling unwritten spaces in a fixed-width record.
*
e.g. if a field has a length of 5 characters, but the value is 'ZZ', the field should contain [ZZ ] (i.e. ZZ followed by 3 unwritten spaces).
*
If the padding is set to '_', then the field will be written as [ZZ___]
*
{@link #padding} defaults to ' '
*
*
*
* @see FixedWidthEntityConfiguration
* @see TextFormat
*
* @author uniVocity Software Pty Ltd - [email protected]
*
*/
public final class FixedWidthFormat extends TextFormat {
private Character padding;
/**
* Returns the character used for filling unwritten spaces in a fixed-width record.
* e.g. if a field has a length of 5 characters, but the value is 'ZZ', the field should contain [ZZ ] (i.e. ZZ followed by 3 unwritten spaces).
*
If the padding is set to '_', then the field will be written as [ZZ___]
*
Defaults to ' '
* @return the padding character
*/
public final char getPadding() {
if (padding == null) {
return ' ';
}
return padding;
}
/**
* Defines the character used for filling unwritten spaces in a fixed-width record.
*
e.g. if a field has a length of 5 characters, but the value is 'ZZ', the field should contain [ZZ ] (i.e. ZZ followed by 3 unwritten spaces).
*
If the padding is set to '_', then the field will be written as [ZZ___]
* @param padding the padding character
*/
public final void setPadding(char padding) {
this.padding = padding;
}
/**
* Identifies whether or not a given character is used for representing unwritten spaces in a fixed-width record.
*
* @param ch the character to be verified
* @return {@code true} if the given character is the padding character, otherwise {@code false}
*/
public final boolean isPadding(char ch) {
return getPadding() == ch;
}
/**
* {@inheritDoc}
*/
@Override
protected final void copyDefaultsFrom(Configuration defaultConfig) {
super.copyDefaultsFrom(defaultConfig);
FixedWidthFormat defaults = (FixedWidthFormat) defaultConfig;
if (padding == null) {
padding = defaults.getPadding();
}
}
}