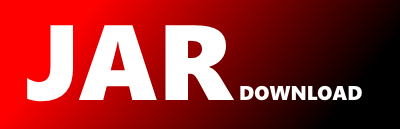
com.univocity.api.engine.FunctionCall Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2013 uniVocity Software Pty Ltd. All rights reserved.
* This file is subject to the terms and conditions defined in file
* 'LICENSE.txt', which is part of this source code package.
******************************************************************************/
package com.univocity.api.engine;
import com.univocity.api.config.annotation.*;
/**
* A custom function call that can be used from within the {@link DataIntegrationEngine} to obtain values from the current {@link EngineScope}, and to execute any external
* operation that produces values required while executing data mappings.
*
* Use {@link DataIntegrationEngine#addFunction(EngineScope, String, FunctionCall)} to associate it with a name and a scope.
* Use {@link DataIntegrationEngine#addFunctions(Object...)} to automatically create functions with the methods annotated with {@link FunctionWrapper}.
* The object instances will be used to execute the function calls and there is no restriction on their state. Keep in mind that, depending on your configuration,
* concurrent function invocations might occur. In this situation it is important either to avoid sharing an object with multiple functions or to synchronize any code that alters its state.
*
* Calls executed within uniVocity will cache the function result in the given scope and will only be executed again once data stored in that scope is lost.
*
* Functions can be used to transform mapped values. They are expressed in mappings using special expressions. For example, consider the function getLocaleId:
*
*
* engine.addFunction(EngineScope.APPLICATION, "getLocaleId", new FunctionCall<Integer, String>() {
* public Integer execute(String locale) {
* return getLocaleId(locale); // fetches the ID of a given locale from an external service.
* }
* });
*
*
* Here we use the getLocaleId function to execute against locale codes read from a source entity,
* generating locale IDs to be written into the destination:
*
* // invokes getLocaleId with the en_AU String as an argument.:
* mapping.identity().associate("locale_code").to("locale_id").readingWith("getLocaleId");
*
*
* The getLocaleId function can be used as a source field in a mapping as well. When used within a field mapping, the function must be
* enclosed within curly braces. The following example uses it to obtain the en_AU locale ID in a mapping using different constructs:
*
* // invokes getLocaleId with the "en_AU" String as an argument
* mapping.identity().associate("{getLocaleId(en_AU)}").to("locale_id");
*
* //or: invokes getLocaleId with the value of the variable currentLocale
* mapping.identity().associate("{getLocaleId($currentLocale)}").to("locale_id");
*
* //or: invokes getLocaleId with the value produced by another function call
* mapping.identity().associate("{getLocaleId(getCurrentLocale())}").to("locale_id");
*
*
* There is no limitation on the number of arguments used to invoke a function. If a function takes multiple arguments, use an array of {@code Object}s.
*
* @see FunctionWrapper
* @see EngineScope
* @see DataIntegrationEngine
*
* @author uniVocity Software Pty Ltd - [email protected]
*
* @param the output type of this function
* @param the input type expected for this function.
*/
public interface FunctionCall {
/**
* Executes the function and returns the result (if any)
*
* @param input the function arguments
* @return the function result.
*/
O execute(I input);
}