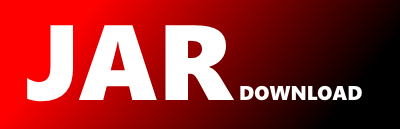
com.univocity.api.engine.RowMappingContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of univocity-api Show documentation
Show all versions of univocity-api Show documentation
uniVocity Data Integration's Public API
The newest version!
/*******************************************************************************
* Copyright (c) 2013 uniVocity Software Pty Ltd. All rights reserved.
* This file is subject to the terms and conditions defined in file
* 'LICENSE.txt', which is part of this source code package.
******************************************************************************/
package com.univocity.api.engine;
import com.univocity.api.config.builders.*;
/**
* The RowMappingContext
is available to {@link RowReader} instances used during the execution of a data mapping between two entities.
*
* It provides information specific to the mapping being executed, as well as access to the {@link DataIntegrationEngine} execution context.
*
*
* @see RowReader
* @see EntityMappingContext
* @see EngineExecutionContext
* @see MappingCycleContext
*
* @author uniVocity Software Pty Ltd - [email protected]
*
*/
public interface RowMappingContext extends EntityMappingContext, EngineExecutionContext, MappingCycleContext {
/**
* Discards the current row being processed. This behavior varies depending where the {@link RowReader} is applied:
*
*
* - while reading from the input
{@link EntityMapping#addInputRowReader(RowReader)}
:
*
discards the input row. The discarded row won't be available to RowReader
instances that manipulate output rows.
*
* - before writing to the output
{@link EntityMapping#addInputRowReader(RowReader)}
:
*
discards the output row. The discarded row won't be available to RowReader
instances that manipulate persisted rows.
*
* - after writing to the output
{@link EntityMapping#addInputRowReader(RowReader)}
:
*
does nothing.
*
*
*/
public void discardRow();
/**
* Returns the position of a field in the input row.
* @param fieldName the name of a field in the input row.
* @return the position of the given field name in the input row.
*/
public int getInputIndex(String fieldName);
/**
* Returns the position of a field in the output row (if available).
* @param fieldName the name of a field in the output row.
* @return the position of the given field name in the output row.
*/
public int getOutputIndex(String fieldName);
/**
* Return the current count of rows processed.
* @return the current count of rows processed.
*/
public int getCurrentRow();
/**
* Returns the sequence of fields read from the input data entity.
* @return the sequence of fields read from the input data entity.
*/
public String[] getInputFields();
/**
* Returns the sequence of fields read from the output data entity (if available).
* @return the sequence of fields read from the output data entity.
*/
public String[] getOutputFields();
/**
* Returns the value of a given field in the current input row.
* @param fieldName name of the input field whose value will be returned.
* @return the value of the field in the current input row.
*/
public Object getInputValue(String fieldName);
/**
* Returns the value of a given field in the current output row.
* @param fieldName name of the output field whose value will be returned.
* @return the value of the field in the current output row.
*/
public Object getOutputValue(String fieldName);
/**
* Modifies the value of a given field in the current input row.
* @param fieldName name of the input field whose value will be modified.
* @param value the new value of the field in the current input row.
*/
public void setInputValue(String fieldName, Object value);
/**
* Modifies the value of a given field in the current output row.
* @param fieldName name of the output field whose value will be modified.
* @param value the new value of the field in the current output row.
*/
public void setOutputValue(String fieldName, Object value);
/**
* Returns the value of a given field in the current input row.
* @param fieldType the type of the value stored in the input field
* @param fieldName name of the input field whose value will be returned.
* @param fieldType the class of the value stored in the input field
* @return the value of the field in the current input row.
*
*/
public T getInputValue(String fieldName, Class fieldType);
/**
* Returns the value of a given field in the current output row.
* @param fieldType the type of the value stored in the output field
* @param fieldName name of the output field whose value will be returned.
* @param fieldType the class of the value stored in the output field
* @return the value of the field in the current output row.
*/
public T getOutputValue(String fieldName, Class fieldType);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy