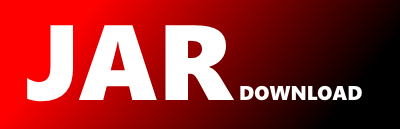
com.alibaba.dubbo.remoting.zookeeper.zkclient.ZkclientZookeeperClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dubbo2 Show documentation
Show all versions of dubbo2 Show documentation
The all in one project of dubbo2
The newest version!
package com.alibaba.dubbo.remoting.zookeeper.zkclient;
import java.util.List;
import com.alibaba.dubbo.common.Constants;
import org.I0Itec.zkclient.IZkChildListener;
import org.I0Itec.zkclient.IZkStateListener;
import org.I0Itec.zkclient.ZkClient;
import org.I0Itec.zkclient.exception.ZkNoNodeException;
import org.I0Itec.zkclient.exception.ZkNodeExistsException;
import org.apache.zookeeper.Watcher.Event.KeeperState;
import com.alibaba.dubbo.common.URL;
import com.alibaba.dubbo.remoting.zookeeper.ChildListener;
import com.alibaba.dubbo.remoting.zookeeper.StateListener;
import com.alibaba.dubbo.remoting.zookeeper.support.AbstractZookeeperClient;
public class ZkclientZookeeperClient extends AbstractZookeeperClient {
private final ZkClient client;
private volatile KeeperState state = KeeperState.SyncConnected;
public ZkclientZookeeperClient(URL url) {
super(url);
client = new ZkClient(
url.getBackupAddress(),
url.getParameter(Constants.SESSION_TIMEOUT_KEY, Constants.DEFAULT_SESSION_TIMEOUT),
url.getParameter(Constants.TIMEOUT_KEY, Constants.DEFAULT_REGISTRY_CONNECT_TIMEOUT));
client.subscribeStateChanges(new IZkStateListener() {
public void handleStateChanged(KeeperState state) throws Exception {
ZkclientZookeeperClient.this.state = state;
if (state == KeeperState.Disconnected) {
stateChanged(StateListener.DISCONNECTED);
} else if (state == KeeperState.SyncConnected) {
stateChanged(StateListener.CONNECTED);
}
}
public void handleNewSession() throws Exception {
stateChanged(StateListener.RECONNECTED);
}
});
}
public void createPersistent(String path) {
try {
client.createPersistent(path, true);
} catch (ZkNodeExistsException e) {
}
}
public void createEphemeral(String path) {
try {
client.createEphemeral(path);
} catch (ZkNodeExistsException e) {
}
}
public void delete(String path) {
try {
client.delete(path);
} catch (ZkNoNodeException e) {
}
}
public List getChildren(String path) {
try {
return client.getChildren(path);
} catch (ZkNoNodeException e) {
return null;
}
}
public boolean isConnected() {
return state == KeeperState.SyncConnected;
}
public void doClose() {
client.close();
}
public IZkChildListener createTargetChildListener(String path, final ChildListener listener) {
return new IZkChildListener() {
public void handleChildChange(String parentPath, List currentChilds)
throws Exception {
listener.childChanged(parentPath, currentChilds);
}
};
}
public List addTargetChildListener(String path, final IZkChildListener listener) {
return client.subscribeChildChanges(path, listener);
}
public void removeTargetChildListener(String path, IZkChildListener listener) {
client.unsubscribeChildChanges(path, listener);
}
}