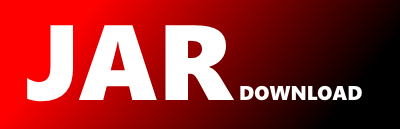
com.upplication.cordova.BuildIOsOpts Maven / Gradle / Ivy
package com.upplication.cordova;
import java.io.File;
import java.util.List;
public class BuildIOsOpts extends BuildOpts {
/**
* Type of signing identity used for code signing.
*/
private String codeSignIdentity;
/**
* Path to ResourceRules.plist.
*/
private File codeSignResourceRules;
/**
* UUID of the profile.
*/
private String provisioningProfile;
/**
* This is new for Xcode 8. The development team (Team ID) to use for code signing.
* You would use this setting and a simplified Code Sign Identity (i.e. just 'iPhone Developer') to sign your apps, you do not need to provide a Provisioning Profile.
*/
private String developmentTeam;
/**
* This will determine what type of build is generated by Xcode. Valid options are development (the default), enterprise, ad-hoc, and app-store.
*/
private String packageType;
/**
* Builds project without application signing.
*/
private boolean noSign;
/**
* TODO: https://github.com/apache/cordova-ios/blob/b8e855b87045f255c9183c4885fc62d74792f616/bin/templates/scripts/cordova/lib/build.js#44
*
* cordova build --device --buildFlag="MYSETTING=myvalue" --buildFlag="MY_OTHER_SETTING=othervalue"
* cordova run --device --buildFlag="DEVELOPMENT_TEAM=FG35JLLMXX4A" --buildFlag="-scheme TestSchemeFlag"
*
* -xcconfig
* -workspace
* -scheme
* -configuration
* -sdk
* -destination
* -archivePath
* CONFIGURATION_BUILD_DIR=
* SHARED_PRECOMPS_DIR=
*/
private String[] buildFlag;
public static BuildIOsOpts create() {
return new BuildIOsOpts();
}
public String getCodeSignIdentity() {
return codeSignIdentity;
}
public BuildIOsOpts withCodeSignIdentity(String codeSignIdentity) {
this.codeSignIdentity = codeSignIdentity;
return this;
}
public File getCodeSignResourceRules() {
return codeSignResourceRules;
}
public BuildIOsOpts withCodeSignResourceRules(File codeSignResourceRules) {
this.codeSignResourceRules = codeSignResourceRules;
return this;
}
public String getProvisioningProfile() {
return provisioningProfile;
}
public BuildIOsOpts withProvisioningProfile(String provisioningProfile) {
this.provisioningProfile = provisioningProfile;
return this;
}
public String getDevelopmentTeam() {
return developmentTeam;
}
public BuildIOsOpts withDevelopmentTeam(String developmentTeam) {
this.developmentTeam = developmentTeam;
return this;
}
public String getPackageType() {
return packageType;
}
public BuildIOsOpts withPackageType(String packageType) {
this.packageType = packageType;
return this;
}
public boolean isNoSign() {
return noSign;
}
public BuildIOsOpts withNoSign(boolean noSign) {
this.noSign = noSign;
return this;
}
public String[] getBuildFlag() {
return buildFlag;
}
public BuildIOsOpts withBuildFlag(String ... buildFlag) {
this.buildFlag = buildFlag;
return this;
}
@Override
public List toList() {
List commands = super.toList();
if (codeSignIdentity != null)
commands.add("--codeSignIdentity=" + codeSignIdentity);
if (codeSignResourceRules != null)
commands.add("--codeSignResourceRules=" + codeSignResourceRules.getAbsolutePath());
if (provisioningProfile != null)
commands.add("--provisioningProfile=" + provisioningProfile);
if (developmentTeam != null)
commands.add("--developmentTeam=" + developmentTeam);
if (packageType != null)
commands.add("--packageType=" + packageType);
if (noSign)
commands.add("--noSign");
if (buildFlag != null) {
for (String flag : buildFlag){
commands.add("--buildFlag=" + flag);
}
}
return commands;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy