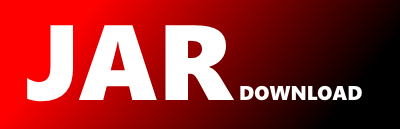
com.urbanairship.connect.client.RawEventReceiver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connect-client Show documentation
Show all versions of connect-client Show documentation
The UA Connect Java client library
/*
Copyright 2015 Urban Airship and Contributors
*/
package com.urbanairship.connect.client;
import com.google.common.base.Supplier;
import com.google.gson.Gson;
import com.google.gson.JsonParseException;
import com.google.gson.JsonSyntaxException;
import com.urbanairship.connect.client.model.GsonUtil;
import com.urbanairship.connect.client.model.responses.Event;
import com.urbanairship.connect.java8.Consumer;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
import java.util.concurrent.atomic.AtomicReference;
/**
* Class that consumes and parses the API response while tracking the stream offset.
*/
public class RawEventReceiver implements Consumer, Supplier {
private static final Logger log = LogManager.getLogger(RawEventReceiver.class);
private static final Gson gson = GsonUtil.getGson();
private AtomicReference lastOffset = new AtomicReference<>("0");
private final Consumer consumer;
/**
* Default constructor
*
* @param consumer {@code Consumer} Implemented by a library user to consume response POJOs.
*/
public RawEventReceiver(Consumer consumer) {
this.consumer = consumer;
}
/**
* Accepts an API response entry and parses it into an {@link com.urbanairship.connect.client.model.responses.Event}.
* The offset is then retrieved from the parsed response, which then gets passed into the Event consumer.
*
* @param event String a single event from the API response.
*/
@Override
public void accept(String event) {
try {
Event eventObj = gson.fromJson(event, Event.class);
log.debug("Parsing event " + eventObj.getIdentifier());
lastOffset.set(eventObj.getOffset());
consumer.accept(eventObj);
} catch (JsonSyntaxException e) {
log.warn("Error parsing " + event, e);
} catch (JsonParseException e) {
log.warn("Error parsing " + event, e);
}
}
/**
* Gets the last stream offset.
*
* @return offset
*/
@Override
public String get() {
return lastOffset.get();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy