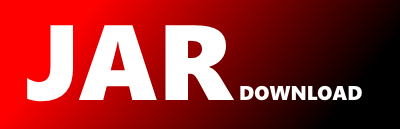
com.urbanairship.api.nameduser.model.NamedUserUpdateResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
package com.urbanairship.api.nameduser.model;
import com.google.common.base.Objects;
import com.google.common.collect.ImmutableList;
import com.urbanairship.api.common.model.ErrorDetails;
import java.util.Optional;
public class NamedUserUpdateResponse {
private final boolean ok;
private final Optional error;
private final Optional> attributeWarnings;
private final Optional> tagWarnings;
private final Optional errorDetails;
private NamedUserUpdateResponse(Builder builder) {
this.ok = builder.ok;
this.error = builder.error;
this.attributeWarnings = builder.attributeWarnings;
this.tagWarnings = builder.tagWarnings;
this.errorDetails = builder.errorDetails;
}
public static Builder newBuilder() {
return new Builder();
}
public boolean isOk() {
return ok;
}
public Optional getError() {
return error;
}
public Optional> getAttributeWarnings() {
return attributeWarnings;
}
public Optional> getTagWarnings() {
return tagWarnings;
}
public Optional getErrorDetails() {
return errorDetails;
}
@Override
public String toString() {
return "NamedUserUpdateResponse{" +
"ok=" + ok +
", error='" + error + '\'' +
", attributeWarnings=" + attributeWarnings +
", tagWarnings=" + tagWarnings +
", errorDetails=" + errorDetails +
'}';
}
@Override
public int hashCode() {
return Objects.hashCode(ok, error, attributeWarnings, tagWarnings, errorDetails);
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
final NamedUserUpdateResponse other = (NamedUserUpdateResponse) obj;
return Objects.equal(this.ok, other.ok) && Objects.equal(this.error, other.error) && Objects.equal(this.attributeWarnings, other.attributeWarnings) && Objects.equal(this.tagWarnings, other.tagWarnings) &&
Objects.equal(this.errorDetails, other.errorDetails);
}
public static class Builder {
boolean ok;
Optional error;
Optional> attributeWarnings;
Optional> tagWarnings;
Optional errorDetails;
public Builder setOk(boolean ok) {
this.ok = ok;
return this;
}
public Builder setError(Optional string) {
this.error = string;
return this;
}
public Builder setAttributeWarnings(Optional> attributeWarnings) {
this.attributeWarnings = attributeWarnings;
return this;
}
public Builder setTagWarnings(Optional> tagWarnings) {
this.tagWarnings = tagWarnings;
return this;
}
public Builder setErrorDetails(Optional errorDetails) {
this.errorDetails = errorDetails;
return this;
}
public NamedUserUpdateResponse build() {
return new NamedUserUpdateResponse(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy