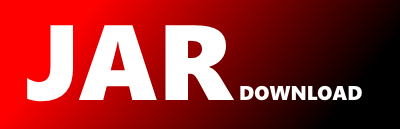
com.urbanairship.api.reports.model.CustomEventsDetailsListingResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
/*
* Copyright (c) 2013-2022. Airship and Contributors
*/
package com.urbanairship.api.reports.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.ImmutableList;
import java.util.Objects;
import java.util.Optional;
public class CustomEventsDetailsListingResponse {
private final boolean ok;
private final Float totalValue;
private final Integer totalCount;
private final String next_page;
private final String prev_page;
private final ImmutableList events;
public CustomEventsDetailsListingResponse(
@JsonProperty("ok") boolean ok,
@JsonProperty("next_page") String next_page,
@JsonProperty("prev_page") String prev_page,
@JsonProperty("total_value") Float totalValue,
@JsonProperty("total_count") Integer totalCount,
@JsonProperty("events") ImmutableList events
) {
this.ok = ok;
this.totalValue = totalValue;
this.totalCount = totalCount;
this.next_page = next_page;
this.prev_page = prev_page;
if (events == null){
this.events = ImmutableList.of();
}
else {
this.events = events;
}
}
public boolean getOk() {
return ok;
}
public Optional getTotalValue() {
return Optional.ofNullable(totalValue);
}
public Optional getTotalCount() {
return Optional.ofNullable(totalCount);
}
/**
* Get the next page attribute if present for a CustomEventsDetailsListingRequest.
*
* @return An optional string
*/
public Optional getNextPage() {
return Optional.ofNullable(next_page);
}
/**
* Get the prev page if present for a CustomEventsDetailsListingRequest.
*
* @return An optional string
*/
public Optional getPrevPage() {
return Optional.ofNullable(prev_page);
}
/**
* Get the list of event objects for a CustomEventsDetailsListingRequest
*
* @return An optional immutable list of event objects
*/
public Optional> getEvents() {
return Optional.ofNullable(events);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CustomEventsDetailsListingResponse that = (CustomEventsDetailsListingResponse) o;
return Objects.equals(next_page, that.next_page) &&
Objects.equals(prev_page, that.prev_page) &&
Objects.equals(totalValue, that.totalValue) &&
Objects.equals(totalCount, that.totalCount) &&
Objects.equals(events, that.events);
}
@Override
public int hashCode() {
return Objects.hash(next_page, prev_page, totalValue, totalCount, events);
}
@Override
public String toString() {
return "CustomEventsDetailsListingResponse{" +
"next_page=" + next_page +
"prev_page=" + prev_page +
"totalValue=" + totalValue +
"totalCount=" + totalCount +
", events=" + events +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy