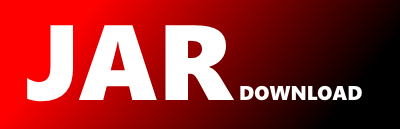
com.urbanairship.api.channel.parse.ChannelViewReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
/*
* Copyright (c) 2013-2016. Urban Airship and Contributors
*/
package com.urbanairship.api.channel.parse;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.urbanairship.api.channel.Constants;
import com.urbanairship.api.channel.model.ChannelView;
import com.urbanairship.api.channel.model.ios.IosSettings;
import com.urbanairship.api.channel.model.open.OpenChannel;
import com.urbanairship.api.channel.model.web.WebSettings;
import com.urbanairship.api.common.parse.APIParsingException;
import com.urbanairship.api.common.parse.BooleanFieldDeserializer;
import com.urbanairship.api.common.parse.JsonObjectReader;
import com.urbanairship.api.common.parse.ListOfStringsDeserializer;
import com.urbanairship.api.common.parse.StringFieldDeserializer;
import org.joda.time.DateTime;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public final class ChannelViewReader implements JsonObjectReader {
private static final ObjectMapper MAPPER = ChannelObjectMapper.getInstance();
private final ChannelView.Builder builder;
public ChannelViewReader() {
builder = ChannelView.newBuilder();
}
public void readChannelId(JsonParser jsonParser) throws IOException {
builder.setChannelId(StringFieldDeserializer.INSTANCE.deserialize(jsonParser, Constants.CHANNEL_ID));
}
public void readDeviceType(JsonParser jsonParser) throws IOException {
builder.setChannelType(StringFieldDeserializer.INSTANCE.deserialize(jsonParser, Constants.DEVICE_TYPE));
}
public void readInstalled(JsonParser jsonParser) throws IOException {
builder.setInstalled(BooleanFieldDeserializer.INSTANCE.deserialize(jsonParser, Constants.INSTALLED));
}
public void readOptIn(JsonParser jsonParser) throws IOException {
builder.setOptIn(BooleanFieldDeserializer.INSTANCE.deserialize(jsonParser, Constants.OPT_IN));
}
public void readBackground(JsonParser jsonParser) throws IOException {
builder.setBackground(BooleanFieldDeserializer.INSTANCE.deserialize(jsonParser, Constants.BACKGROUND));
}
public void readPushAddress(JsonParser jsonParser) throws IOException {
builder.setPushAddress(jsonParser.readValueAs(String.class));
}
public void readCreated(JsonParser jsonParser) throws IOException {
builder.setCreated(jsonParser.readValueAs(DateTime.class));
}
public void readLastRegistration(JsonParser jsonParser) throws IOException {
builder.setLastRegistration(jsonParser.readValueAs(DateTime.class));
}
public void readAlias(JsonParser jsonParser) throws IOException {
builder.setAlias(jsonParser.readValueAs(String.class));
}
public void readTags(JsonParser jsonParser) throws IOException {
builder.addAllTags(ListOfStringsDeserializer.INSTANCE.deserialize(jsonParser, Constants.TAGS));
}
public void readTagGroups(JsonParser jsonParser) throws IOException {
Map> mutableTagGroups = jsonParser.readValueAs(new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy