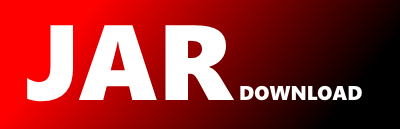
com.urbanairship.api.push.model.notification.ios.IOSAlertData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
/*
* Copyright (c) 2013-2016. Urban Airship and Contributors
*/
package com.urbanairship.api.push.model.notification.ios;
import com.urbanairship.api.push.model.PushModelObject;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
public final class IOSAlertData extends PushModelObject {
private final Optional body;
private final Optional actionLocKey;
private final Optional locKey;
private final Optional> locArgs;
private final Optional launchImage;
private final Optional summaryArg;
private final Optional summaryArgCount;
private final Optional title;
private final Optional> titleLocArgs;
private final Optional titleLocKey;
private final Optional> subtitleLocArgs;
private final Optional subtitleLocKey;
private IOSAlertData(Optional body,
Optional actionLocKey,
Optional locKey,
Optional> locArgs,
Optional launchImage,
Optional summaryArg,
Optional summaryArgCount,
Optional title,
Optional> titleLocArgs,
Optional titleLocKey,
Optional> subtitleLocArgs,
Optional subtitleLocKey) {
this.body = body;
this.actionLocKey = actionLocKey;
this.locKey = locKey;
this.locArgs = locArgs;
this.launchImage = launchImage;
this.summaryArg = summaryArg;
this.summaryArgCount = summaryArgCount;
this.title = title;
this.titleLocArgs = titleLocArgs;
this.titleLocKey = titleLocKey;
this.subtitleLocArgs = subtitleLocArgs;
this.subtitleLocKey = subtitleLocKey;
}
public static Builder newBuilder() {
return new Builder();
}
public boolean isCompound() {
return actionLocKey.isPresent() ||
locKey.isPresent() ||
locArgs.isPresent() ||
launchImage.isPresent() ||
summaryArg.isPresent() ||
summaryArgCount.isPresent() ||
title.isPresent() ||
titleLocArgs.isPresent() ||
titleLocKey.isPresent() ||
subtitleLocArgs.isPresent() ||
subtitleLocKey.isPresent();
}
public Optional getBody() {
return body;
}
public Optional getActionLocKey() {
return actionLocKey;
}
public Optional getLocKey() {
return locKey;
}
public Optional> getLocArgs() {
return locArgs;
}
public Optional getLaunchImage() {
return launchImage;
}
public Optional getSummaryArg() {
return summaryArg;
}
public Optional getSummaryArgCount() {
return summaryArgCount;
}
public Optional getTitle() {
return title;
}
public Optional> getTitleLocArgs() {
return titleLocArgs;
}
public Optional getTitleLocKey() {
return titleLocKey;
}
public Optional> getSubtitleLocArgs() {
return subtitleLocArgs;
}
public Optional getSubtitleLocKey() {
return subtitleLocKey;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
IOSAlertData that = (IOSAlertData) o;
return Objects.equals(body, that.body) &&
Objects.equals(actionLocKey, that.actionLocKey) &&
Objects.equals(locKey, that.locKey) &&
Objects.equals(locArgs, that.locArgs) &&
Objects.equals(launchImage, that.launchImage) &&
Objects.equals(summaryArg, that.summaryArg) &&
Objects.equals(summaryArgCount, that.summaryArgCount) &&
Objects.equals(title, that.title) &&
Objects.equals(titleLocArgs, that.titleLocArgs) &&
Objects.equals(titleLocKey, that.titleLocKey) &&
Objects.equals(subtitleLocArgs, that.subtitleLocArgs) &&
Objects.equals(subtitleLocKey, that.subtitleLocKey);
}
@Override
public int hashCode() {
return Objects.hash(body, actionLocKey, locKey, locArgs, launchImage, summaryArg, summaryArgCount, title, titleLocArgs, titleLocKey, subtitleLocArgs, subtitleLocKey);
}
@Override
public String toString() {
return "IOSAlertData{" +
"body=" + body +
", actionLocKey=" + actionLocKey +
", locKey=" + locKey +
", locArgs=" + locArgs +
", launchImage=" + launchImage +
", summaryArg=" + summaryArg +
", summaryArgCount=" + summaryArgCount +
", title=" + title +
", titleLocArgs=" + titleLocArgs +
", titleLocKey=" + titleLocKey +
", subtitleLocArgs=" + subtitleLocArgs +
", subtitleLocKey=" + subtitleLocKey +
'}';
}
public static class Builder {
private String body = null;
private String actionLocKey = null;
private String locKey = null;
private List locArgs = null;
private String launchImage = null;
private String summaryArg = null;
private Integer summaryArgCount = null;
private String title = null;
private List titleLocArgs = null;
private String titleLocKey = null;
private List subtitleLocArgs = null;
private String subtitleLocKey = null;
private Builder() { }
public Builder setBody(String body) {
this.body = body;
return this;
}
public Builder setActionLocKey(String value) {
this.actionLocKey = value;
return this;
}
public Builder setLocKey(String value) {
this.locKey = value;
return this;
}
public Builder setLocArgs(List value) {
this.locArgs = value;
return this;
}
public Builder setLaunchImage(String value) {
this.launchImage = value;
return this;
}
public Builder setSummaryArg(String value) {
this.summaryArg = value;
return this;
}
public Builder setSummaryArgCount(Integer value) {
this.summaryArgCount = value;
return this;
}
public Builder setTitle(String value) {
this.title = value;
return this;
}
public Builder setTitleLocArgs(List value) {
this.titleLocArgs = value;
return this;
}
public Builder setTitleLocKey(String value) {
this.titleLocKey = value;
return this;
}
public Builder setSubtitleLocArgs(List value) {
this.subtitleLocArgs = value;
return this;
}
public Builder setSubtitleLocKey(String value) {
this.subtitleLocKey = value;
return this;
}
public IOSAlertData build() {
return new IOSAlertData(Optional.ofNullable(body),
Optional.ofNullable(actionLocKey),
Optional.ofNullable(locKey),
Optional.ofNullable(locArgs),
Optional.ofNullable(launchImage),
Optional.ofNullable(summaryArg),
Optional.ofNullable(summaryArgCount),
Optional.ofNullable(title),
Optional.ofNullable(titleLocArgs),
Optional.ofNullable(titleLocKey),
Optional.ofNullable(subtitleLocArgs),
Optional.ofNullable(subtitleLocKey));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy