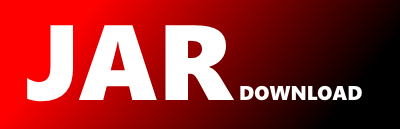
com.urbanairship.api.push.model.notification.wns.WNSBinding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
/*
* Copyright (c) 2013-2016. Urban Airship and Contributors
*/
package com.urbanairship.api.push.model.notification.wns;
import com.google.common.collect.ImmutableList;
import java.util.Optional;
public class WNSBinding {
private final String template;
private final Optional version;
private final Optional fallback;
private final Optional lang;
private final Optional baseUri;
private final Optional addImageQuery;
private final Optional> images;
private final Optional> text;
private WNSBinding(String template,
Optional version,
Optional fallback,
Optional lang,
Optional baseUri,
Optional addImageQuery,
Optional> images,
Optional> text)
{
this.template = template;
this.version = version;
this.fallback = fallback;
this.lang = lang;
this.baseUri = baseUri;
this.addImageQuery = addImageQuery;
this.images = images;
this.text = text;
}
public static Builder newBuilder() {
return new Builder();
}
public String getTemplate() {
return this.template;
}
public Optional getVersion() {
return this.version;
}
public Optional getFallback() {
return this.fallback;
}
public Optional getLang() {
return this.lang;
}
public Optional getBaseUri() {
return this.baseUri;
}
public Optional getAddImageQuery() {
return this.addImageQuery;
}
public int getImageCount() {
return images.isPresent() ? images.get().size() : 0;
}
public String getImage(int i) {
return images.get().get(i);
}
public Optional> getImages() {
return this.images;
}
public int getTextCount() {
return text.isPresent() ? text.get().size() : 0;
}
public String getText(int i) {
return text.get().get(i);
}
public Optional> getText() {
return this.text;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WNSBinding that = (WNSBinding)o;
if (template != null ? !template.equals(that.template) : that.template != null) {
return false;
}
if (version != null ? !version.equals(that.version) : that.version != null) {
return false;
}
if (fallback != null ? !fallback.equals(that.fallback) : that.fallback != null) {
return false;
}
if (lang != null ? !lang.equals(that.lang) : that.lang != null) {
return false;
}
if (baseUri != null ? !baseUri.equals(that.baseUri) : that.baseUri != null) {
return false;
}
if (addImageQuery != null ? !addImageQuery.equals(that.addImageQuery) : that.addImageQuery != null) {
return false;
}
if (images != null ? !images.equals(that.images) : that.images != null) {
return false;
}
if (text != null ? !text.equals(that.text) : that.text != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = (template != null ? template.hashCode() : 0);
result = 31 * result + (version != null ? version.hashCode() : 0);
result = 31 * result + (fallback != null ? fallback.hashCode() : 0);
result = 31 * result + (lang != null ? lang.hashCode() : 0);
result = 31 * result + (baseUri != null ? baseUri.hashCode() : 0);
result = 31 * result + (addImageQuery != null ? addImageQuery.hashCode() : 0);
result = 31 * result + (images != null ? images.hashCode() : 0);
result = 31 * result + (text != null ? text.hashCode() : 0);
return result;
}
public static class Builder {
private String template;
private Integer version;
private String fallback;
private String lang;
private String baseUri;
private Boolean addImageQuery;
private ImmutableList.Builder images;
private ImmutableList.Builder text;
private Builder() { }
public Builder setTemplate(String value) {
this.template = value;
return this;
}
public Builder setVersion(Integer value) {
this.version = value;
return this;
}
public Builder setFallback(String value) {
this.fallback = value;
return this;
}
public Builder setLang(String value) {
this.lang = value;
return this;
}
public Builder setBaseUri(String value) {
this.baseUri = value;
return this;
}
public Builder setAddImageQuery(boolean value) {
this.addImageQuery = value;
return this;
}
public Builder addImage(String value) {
if (this.images == null) {
this.images = ImmutableList.builder();
}
this.images.add(value);
return this;
}
public Builder addAllImages(Iterable values) {
if (this.images == null) {
this.images = ImmutableList.builder();
}
this.images.addAll(values);
return this;
}
public Builder addText(String value) {
if (this.text == null) {
this.text = ImmutableList.builder();
}
this.text.add(value);
return this;
}
public Builder addAllText(Iterable values) {
if (this.text == null) {
this.text = ImmutableList.builder();
}
this.text.addAll(values);
return this;
}
public WNSBinding build() {
if (template == null) {
throw new IllegalArgumentException("Must supply a value for 'template'.");
}
return new WNSBinding(template,
Optional.ofNullable(version),
Optional.ofNullable(fallback),
Optional.ofNullable(lang),
Optional.ofNullable(baseUri),
Optional.ofNullable(addImageQuery),
Optional.ofNullable(images != null ? images.build() : null),
Optional.ofNullable(text != null ? text.build() : null));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy