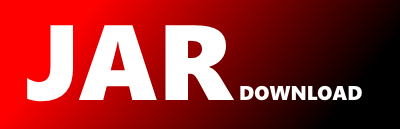
com.urbanairship.api.createandsend.model.notification.email.CreateAndSendEmailPayload Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
package com.urbanairship.api.createandsend.model.notification.email;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.urbanairship.api.push.model.DeviceType;
import com.urbanairship.api.push.model.PushModelObject;
import com.urbanairship.api.push.model.notification.DevicePayloadOverride;
import com.urbanairship.api.push.model.notification.email.Attachment;
import com.urbanairship.api.push.model.notification.email.MessageType;
import org.apache.commons.lang.StringUtils;
import java.util.Optional;
/**
* Represents the payload to be used for registering or updating an email channel.
*/
public class CreateAndSendEmailPayload extends PushModelObject implements DevicePayloadOverride {
private final Optional bypassOptInLevel;
private final Optional alert;
private final Optional subject;
private final Optional htmlBody;
private final Optional plaintextBody;
private final Optional messageType;
private final Optional senderName;
private final Optional senderAddress;
private final Optional replyTo;
private final Optional emailTemplate;
private final Optional> attachments;
private final Optional clickTracking;
private final Optional openTracking;
private CreateAndSendEmailPayload(Builder builder) {
this.alert = Optional.ofNullable(builder.alert);
this.subject = Optional.ofNullable(builder.subject);
this.htmlBody = Optional.ofNullable(builder.htmlBody);
this.plaintextBody = Optional.ofNullable(builder.plaintextBody);
this.messageType = Optional.ofNullable(builder.messageType);
this.senderName = Optional.ofNullable(builder.senderName);
this.senderAddress = Optional.ofNullable((builder.senderAddress));
this.replyTo = Optional.ofNullable((builder.replyTo));
this.bypassOptInLevel = Optional.ofNullable(builder.byPassOptInLevel);
this.emailTemplate = Optional.ofNullable(builder.emailTemplate);
this.clickTracking = Optional.ofNullable(builder.clickTracking);
this.openTracking = Optional.ofNullable(builder.openTracking);
if (builder.attachments.build().isEmpty()) {
this.attachments = Optional.empty();
} else {
this.attachments = Optional.ofNullable(builder.attachments.build());
}
}
public static Builder newBuilder() {
return new Builder();
}
/**
* Returns Devicetype: Email when requested
*
* @return String email
*/
@Override
public DeviceType getDeviceType() {
return DeviceType.EMAIL;
}
/**
* Get the email template, Using a template enables you to provide and populate
* variables in your notification
*
* @return Optional, EmailTemplate
*/
public Optional getEmailTemplate() {
return emailTemplate;
}
/**
* Optional, override the alert value provided at the top level, if any.
*
* @return Optional String alert.
*/
public Optional getAlert() {
return alert;
}
/**
* Optional, a string representing the subject of the notification.
*
* @return Optional String subject.
*/
public Optional getSubject() {
return subject;
}
/**
* Optional, a string value for providing a the HTML body of the notification.
*
* @return Optional String htmlBody
*/
public Optional getHtmlBody() {
return htmlBody;
}
/**
* Optional, a string value for providing a the plaintext Body of the notification.
*
* @return Optional String plaintextBody
*/
public Optional getPlaintextBody() {
return plaintextBody;
}
/**
* Optional, an enum of potential values representing the possible message types.
*
* @return Optional enum messageType.
*/
public Optional getMessageType() {
return messageType;
}
/**
* Optional, a string representing the name of the sender.
*
* @return Optional String senderName.
*/
public Optional getSenderName() {
return senderName;
}
/**
* Optional, a string representing the address of the sender.
*
* @return Optional String senderAddress.
*/
public Optional getSenderAddress() {
return senderAddress;
}
/**
* Optional, a string representing the "reply to" address of the notification.
*
* @return Optional String replyTo.
*/
public Optional getReplyTo() {
return replyTo;
}
/**
* Optional, a boolean toggle you can set when message_type is set to transactional to send a business critical
* email. If true, the message will be sent to your entire audience, ignoring transactional_opted_out status.
*
* @return Optional Boolean bypassOptInLevel
*/
public Optional getBypassOptInLevel() {
return bypassOptInLevel;
}
/**
* Optional, Get the Attachment objects, each containing an id string which represents an email attachment.
*
* @return Optional ImmutableList attachments
*/
public Optional> getAttachments() {
return attachments;
}
/**
* Optional, Get the clickTracking value.
*
* @return Optional Boolean clickTracking
*/
public Optional getClickTracking() {
return clickTracking;
}
/**
* Optional, Get the openTracking value.
*
* @return Optional Boolean openTracking
*/
public Optional getOpenTracking() {
return openTracking;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CreateAndSendEmailPayload that = (CreateAndSendEmailPayload) o;
return Objects.equal(bypassOptInLevel, that.bypassOptInLevel) &&
Objects.equal(alert, that.alert) &&
Objects.equal(subject, that.subject) &&
Objects.equal(htmlBody, that.htmlBody) &&
Objects.equal(plaintextBody, that.plaintextBody) &&
Objects.equal(messageType, that.messageType) &&
Objects.equal(senderName, that.senderName) &&
Objects.equal(senderAddress, that.senderAddress) &&
Objects.equal(replyTo, that.replyTo) &&
Objects.equal(emailTemplate, that.emailTemplate) &&
Objects.equal(attachments, that.attachments) &&
Objects.equal(clickTracking, that.clickTracking) &&
Objects.equal(openTracking, that.openTracking);
}
@Override
public int hashCode() {
return Objects.hashCode(bypassOptInLevel, alert, subject, htmlBody, plaintextBody, messageType, senderName, senderAddress, replyTo, emailTemplate, attachments, clickTracking, openTracking);
}
@Override
public String toString() {
return "CreateAndSendEmailPayload{" +
"bypassOptInLevel=" + bypassOptInLevel +
", alert=" + alert +
", subject=" + subject +
", htmlBody=" + htmlBody +
", plaintextBody=" + plaintextBody +
", messageType=" + messageType +
", senderName=" + senderName +
", senderAddress=" + senderAddress +
", replyTo=" + replyTo +
", emailTemplate=" + emailTemplate +
", attachments=" + attachments +
", clickTracking=" + clickTracking +
", openTracking=" + openTracking +
'}';
}
/**
* CreateAndSendEmailPayload Builder.
*/
public static class Builder {
private String alert = null;
private String subject = null;
private String htmlBody = null;
private String plaintextBody = null;
private MessageType messageType = null;
private String senderName = null;
private String senderAddress = null;
private String replyTo = null;
private DeviceType deviceType = null;
private Boolean byPassOptInLevel = null;
private EmailTemplate emailTemplate = null;
private ImmutableList.Builder attachments = ImmutableList.builder();
private Boolean clickTracking = null;
private Boolean openTracking = null;
private Builder() {
}
/**
* Optional, a string representing the subject of the notification.
*
* @param subject Optional String
* @return CreateAndSendEmailPayload Builder
*/
public Builder setSubject(String subject) {
this.subject = subject;
return this;
}
/**
* Optional, a boolean you can set this toggle when message_type is set to transactional to send a business critical
* email. If true, the message will be sent to your entire audience, ignoring transactional_opted_out status.
* @param byPassOptInLevel Boolean
* @return CreateAndSendEmailPayload Builder
*/
public Builder setByPassOptInLevel(Boolean byPassOptInLevel) {
this.byPassOptInLevel = byPassOptInLevel;
return this;
}
/**
* Optional, a string representing the HTML body of the notification.
*
* @param htmlBody Optional String
* @return CreateAndSendEmailPayload Builder
*/
public Builder setHtmlBody(String htmlBody) {
this.htmlBody = htmlBody;
return this;
}
/**
* Optional, a string representing the plaintext body of the notification.
*
* @param plaintextBody Optional String
* @return CreateAndSendEmailPayload Builder
*/
public Builder setPlaintextBody(String plaintextBody) {
this.plaintextBody = plaintextBody;
return this;
}
/**
* Optional, an enum representing the possible message types of the notification.
*
* @param value Optional Map of Strings
* @return CreateAndSendEmailPayload Builder
*/
public Builder setMessageType(MessageType value) {
this.messageType = value;
return this;
}
/**
* Optional, a string representing the sender address.
*
* @param senderAddress Optional String
* @return CreateAndSendEmailPayload Builder
*/
public Builder setSenderAddress(String senderAddress) {
this.senderAddress = senderAddress;
return this;
}
/**
* Optional, a string representing the reply-to address.
*
* @param replyTo Optional String
* @return CreateAndSendEmailPayload Builder
*/
public Builder setReplyTo(String replyTo) {
this.replyTo = replyTo;
return this;
}
/**
* Optional, a string representing the sender name.
*
* @param senderName Optional String
* Must be set up by Urban Airship before use.
* @return CreateAndSendEmailPayload Builder
*/
public Builder setSenderName(String senderName) {
this.senderName = senderName;
return this;
}
/**
* Provide the ID or inline fields for a template. Using a template enables you to provide and populate
* variables in your notification.
*
* @param emailTemplate EmailTemplate
* @return CreateAndSendEmailPayload Builder
*/
public Builder setEmailTemplate(EmailTemplate emailTemplate) {
this.emailTemplate = emailTemplate;
return this;
}
/**
* Add an Attachment objects, each containing an id string which represents an email attachment.
*
* @param attachment Attachment
* @return CreateAndSendEmailPayload Builder
*/
public Builder addAttachment(Attachment attachment) {
this.attachments.add(attachment);
return this;
}
/**
* Optional, True by default. Set to false to prevent click tracking for GDPR compliance.
* @param clickTracking Boolean
* @return CreateAndSendEmailPayload Builder
*/
public Builder setClickTracking(Boolean clickTracking) {
this.clickTracking = clickTracking;
return this;
}
/**
* Optional, True by default. Set to false to prevent “open” tracking for GDPR compliance.
* @param openTracking Boolean
* @return CreateAndSendEmailPayload Builder
*/
public Builder setOpenTracking(Boolean openTracking) {
this.openTracking = openTracking;
return this;
}
public CreateAndSendEmailPayload build() {
Preconditions.checkArgument(!(emailTemplate != null && subject != null), "subject cannot be used if email template is also set.");
Preconditions.checkArgument(!(subject == null && emailTemplate == null), "subject or email template must be set.");
Preconditions.checkArgument(!(plaintextBody != null && emailTemplate != null), "email plaintext_body must not be specified if email payload is templated");
Preconditions.checkArgument(!(plaintextBody == null && emailTemplate == null), "plaintext body or email template must be set");
Preconditions.checkArgument(!(htmlBody != null && emailTemplate != null), "email htmlBody must not be specified if email payload is templated");
Preconditions.checkNotNull(messageType, "MessageType must be set.");
Preconditions.checkNotNull(senderName, "SenderName must be set.");
Preconditions.checkArgument(StringUtils.isNotBlank(senderName),
"SenderName must not be blank");
Preconditions.checkNotNull(senderAddress, "SenderAddress must be set.");
Preconditions.checkArgument(StringUtils.isNotBlank(senderAddress),
"SenderAddress must not be blank");
Preconditions.checkNotNull(replyTo, "ReplyTo must be set.");
Preconditions.checkArgument(StringUtils.isNotBlank(replyTo),
"ReplyTo must not be blank");
return new CreateAndSendEmailPayload(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy