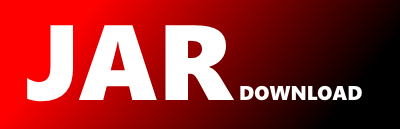
com.urbanairship.api.client.OAuthCredentials Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
package com.urbanairship.api.client;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicNameValuePair;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
public class OAuthCredentials {
private final String clientId;
private final Optional clientSecret;
private final Optional assertionJWT;
private final String grantType = "client_credentials";
private final List ipAddresses;
private final List scopes;
private final Optional sub;
private OAuthCredentials(Builder builder) {
this.clientId = builder.clientId;
this.clientSecret = builder.clientSecret;
this.assertionJWT = builder.assertionJWT;
this.ipAddresses = builder.ipAddresses;
this.scopes = builder.scopes;
this.sub = builder.sub;
}
public static Builder newBuilder(String clientId) {
return new Builder(clientId);
}
public String getClientId() {
return clientId;
}
public Optional getClientSecret() {
return clientSecret;
}
public Optional getAssertionJWT() {
return assertionJWT;
}
public String getGrantType() {
return grantType;
}
public List getIpAddresses() {
return ipAddresses;
}
public List getScopes() {
return scopes;
}
public Optional getSub() {
return sub;
}
/**
* Converts the OAuth credentials to a list of NameValuePair objects suitable for URL encoding.
*
* @return List of NameValuePair objects representing the OAuth credentials.
*/
public List toParams() {
List params = new ArrayList<>();
params.add(new BasicNameValuePair("grant_type", grantType));
assertionJWT.ifPresent(a -> params.add(new BasicNameValuePair("assertion", a)));
ipAddresses.forEach(ipAddress -> params.add(new BasicNameValuePair("ipaddr", ipAddress)));
scopes.forEach(scope -> params.add(new BasicNameValuePair("scope", scope)));
sub.ifPresent(s -> params.add(new BasicNameValuePair("sub", s)));
return params;
}
public static class Builder {
private final String clientId;
private Optional clientSecret = Optional.empty();
private Optional assertionJWT = Optional.empty();
private List ipAddresses = new ArrayList<>();
private List scopes = new ArrayList<>();
private Optional sub = Optional.empty();
public Builder(String clientId) {
this.clientId = clientId;
}
public Builder setClientSecret(String clientSecret) {
this.clientSecret = Optional.ofNullable(clientSecret);
return this;
}
public Builder setAssertionJWT(String assertionJWT) {
this.assertionJWT = Optional.ofNullable(assertionJWT);
return this;
}
public Builder setIpAddresses(List ipAddresses) {
this.ipAddresses = ipAddresses;
return this;
}
public Builder setScopes(List scopes) {
this.scopes = scopes;
return this;
}
public Builder setSub(String sub) {
this.sub = Optional.of(sub);
return this;
}
public OAuthCredentials build() {
if (clientId == null) {
throw new IllegalStateException("clientId must not be null");
}
if (!clientSecret.isPresent() && !assertionJWT.isPresent()) {
throw new IllegalStateException("Either clientSecret or assertionJWT must be provided");
}
if (assertionJWT.isPresent() && (clientSecret.isPresent() || sub.isPresent() || !scopes.isEmpty() || !ipAddresses.isEmpty())) {
throw new IllegalStateException("assertionJWT should not be set with clientSecret, sub, scopes, or ipAddresses.");
}
if (clientSecret.isPresent() && !sub.isPresent()) {
throw new IllegalStateException("sub is required when clientSecret is provided.");
}
return new OAuthCredentials(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy