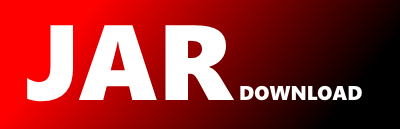
com.urbanairship.api.channel.model.web.Subscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
package com.urbanairship.api.channel.model.web;
import com.google.common.base.Objects;
import java.util.Optional;
/**
* Required for signing the push package, includes the keys "p256dh" and "auth".
*/
public final class Subscription {
private final Optional p256dh;
private final Optional auth;
private Subscription(Optional p256dh, Optional auth) {
this.p256dh = p256dh;
this.auth = auth;
}
/**
* Get the p256dh String
*
* @return p256dh String
*/
public Optional getP256dh() {
return p256dh;
}
/**
* Get the auth String
*
* @return auth String
*/
public Optional getAuth() {
return auth;
}
/**
* New Subscription Builder.
*
* @return Subscription Builder
*/
public static Builder newBuilder() {
return new Builder();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Subscription that = (Subscription) o;
return Objects.equal(p256dh, that.p256dh) &&
Objects.equal(auth, that.auth);
}
@Override
public int hashCode() {
return Objects.hashCode(p256dh, auth);
}
@Override
public String toString() {
return "Subscription{" +
"p256dh=" + p256dh +
", auth=" + auth +
'}';
}
/**
* Subscription Builder.
*/
public final static class Builder {
private String p256dh = null;
private String auth = null;
private Builder() {
}
/**
* Set the p256dh key used in signing the push package.
*
* @param p256dh String key
* @return Subscription Builder
*/
public Builder setP256dh(String p256dh) {
this.p256dh = p256dh;
return this;
}
/**
* Set the auth key used in signing the push package.
*
* @param auth String key
* @return Subscription Builder
*/
public Builder setAuth(String auth) {
this.auth = auth;
return this;
}
public Subscription build() {
return new Subscription(
Optional.ofNullable(p256dh),
Optional.ofNullable(auth)
);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy