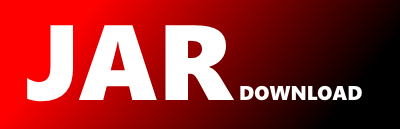
com.urbanairship.api.push.model.audience.location.LocationIdentifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
/*
* Copyright (c) 2013-2016. Urban Airship and Contributors
*/
package com.urbanairship.api.push.model.audience.location;
import com.google.common.base.Preconditions;
import com.urbanairship.api.push.model.PushModelObject;
import java.util.Optional;
/**
* An identifier for a location definition, as seen in either an API
* audience selector expression, or in a segment definition. It can
* contain either an id or an alias, but not both.
*/
public final class LocationIdentifier extends PushModelObject {
private final Optional id;
private final Optional alias;
private LocationIdentifier(Optional id, Optional alias) {
this.id = id;
this.alias = alias;
}
public static Builder newBuilder() {
return new Builder();
}
public boolean isAlias() {
return alias != null && alias.isPresent();
}
public Optional getAlias() {
return alias;
}
public Optional getId() {
return id;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LocationIdentifier that = (LocationIdentifier) o;
if (alias.isPresent() && that.getAlias().isPresent()){
return (alias.hashCode() == that.getAlias().hashCode());
}
else if (id.isPresent() && that.getId().isPresent()){
return (id.hashCode() == that.getId().hashCode());
}
else { return false; }
}
@Override
public int hashCode() {
int result = id != null ? id.hashCode() : 0;
result = 31 * result + (alias != null ? alias.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "LocationIdentifier{" +
"alias=" + alias +
", id='" + id + '\'' +
'}';
}
public static class Builder {
private String id;
private LocationAlias alias;
private Builder() { }
public Builder setId(String value) {
id = value;
return this;
}
public Builder setAlias(LocationAlias value) {
alias = value;
return this;
}
public LocationIdentifier build() {
Preconditions.checkArgument(id != null || alias != null, "Must have only one of 'id' or an alias");
return new LocationIdentifier(Optional.ofNullable(id),
Optional.ofNullable(alias));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy