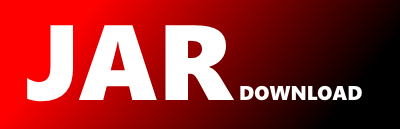
com.urbanairship.api.reports.model.DevicesReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The Urban Airship Java client library
package com.urbanairship.api.reports.model;
import com.google.common.collect.ImmutableList;
import com.urbanairship.api.common.model.ErrorDetails;
import java.util.Objects;
import java.util.Optional;
public class DevicesReport {
private final Optional dateClosed;
private final Optional dateComputed;
private final Optional totalUniqueDevices;
private final Optional> counts;
private final boolean ok;
private final Optional error;
private final Optional errorDetails;
private DevicesReport(Builder builder) {
this.dateClosed = Optional.ofNullable(builder.dateClosed);
this.dateComputed = Optional.ofNullable(builder.dateComputed);
this.totalUniqueDevices = Optional.ofNullable(builder.totalUniqueDevices);
this.counts = Optional.ofNullable(builder.counts.build());
this.ok = builder.ok;
this.error = Optional.ofNullable(builder.error);
this.errorDetails = Optional.ofNullable(builder.errorDetails);
}
public static Builder newBuilder() { return new Builder(); }
/**
* Get the date closed attribute if present for a DevicesReportRequest.
*
* @return An optional string
*/
public Optional getDateClosed() {
return dateClosed;
}
/**
* Get the date computed attribute if present for a DevicesReportRequest.
*
* @return An optional string
*/
public Optional getDateComputed() {
return dateComputed;
}
/**
* Get the total unique devices attribute if present for a DevicesReportRequest.
*
* @return An optional integer
*/
public Optional getTotalUniqueDevices() {
return totalUniqueDevices;
}
/**
* Get the list of DevicesReportResponse objects for a DevicesReportRequest
*
* @return An optional immutable list of DevicesReportResponse objects
*/
public Optional> getCounts() {
return counts;
}
/**
* Get the OK status as a boolean
*
* @return boolean
*/
public boolean getOk() {
return ok;
}
/**
* Get the error if present
*
* @return An Optional String
*/
public Optional getError() {
return error;
}
/**
* Get the error details if present
*
* @return An Optional String
*/
public Optional getErrorDetails() {
return errorDetails;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof DevicesReport)) return false;
DevicesReport that = (DevicesReport) o;
return Objects.equals(dateClosed, that.dateClosed) &&
Objects.equals(dateComputed, that.dateComputed) &&
Objects.equals(totalUniqueDevices, that.totalUniqueDevices) &&
Objects.equals(getCounts(), that.getCounts()) &&
Objects.equals(ok, that.ok) &&
Objects.equals(error, that.error) &&
Objects.equals(errorDetails, that.errorDetails);
}
@Override
public int hashCode() {
return Objects.hash(dateClosed, dateComputed, totalUniqueDevices, getCounts(), ok, error, errorDetails);
}
@Override
public String toString() {
return "DevicesReport{" +
"dateClosed=" + dateClosed +
", dateComputed=" + dateComputed +
", totalUniqueDevices=" + totalUniqueDevices +
", counts=" + counts +
", ok=" + ok +
", error=" + error +
", errorDetails=" + errorDetails +
'}';
}
public static class Builder {
private String dateClosed;
private String dateComputed;
private Integer totalUniqueDevices;
private ImmutableList.Builder counts = ImmutableList.builder();
private boolean ok;
private String error;
private ErrorDetails errorDetails;
private Builder() {}
/**
* Set the date closed
*
* @param dateClosed String
* @return Builder
*/
public Builder setDateClosed(String dateClosed) {
this.dateClosed = dateClosed;
return this;
}
/**
* Set the date computed
*
* @param dateComputed String
* @return Builder
*/
public Builder setDateComputed(String dateComputed) {
this.dateComputed = dateComputed;
return this;
}
/**
* Set the total unique devices
*
* @param totalUniqueDevices String
* @return Builder
*/
public Builder setTotalUniqueDevices(Integer totalUniqueDevices) {
this.totalUniqueDevices = totalUniqueDevices;
return this;
}
/**
* Add a DevicesReportResponse object for a listing
*
* @param object DevicesReportResponse
* @return Builder
*/
public Builder addDevicesReportResponseObject(DevicesReportResponse object) {
this.counts.add(object);
return this;
}
/**
* Set the ok status
*
* @param value boolean
* @return Builder
*/
public Builder setOk(boolean value) {
this.ok = value;
return this;
}
/**
* Set the error
*
* @param error String
* @return Builder
*/
public Builder setError(String error) {
this.error = error;
return this;
}
/**
* Set the errorDetails
*
* @param errorDetails String
* @return Builder
*/
public Builder setErrorDetails(ErrorDetails errorDetails) {
this.errorDetails = errorDetails;
return this;
}
/**
* Build the DevicesReport object
*
* @return DevicesReport
*/
public DevicesReport build() {
return new DevicesReport(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy