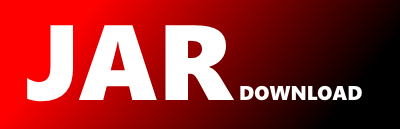
godot.gen.godot.CollisionPolygon2D.kt Maven / Gradle / Ivy
// THIS FILE IS GENERATED! DO NOT EDIT IT MANUALLY!
@file:Suppress("PackageDirectoryMismatch", "unused", "FunctionName", "RedundantModalityModifier",
"UNCHECKED_CAST", "JoinDeclarationAndAssignment", "USELESS_CAST",
"RemoveRedundantQualifierName", "NOTHING_TO_INLINE", "NON_FINAL_MEMBER_IN_OBJECT",
"RedundantVisibilityModifier", "RedundantUnitReturnType", "MemberVisibilityCanBePrivate")
package godot
import godot.`annotation`.GodotBaseType
import godot.core.PackedVector2Array
import godot.core.VariantType.BOOL
import godot.core.VariantType.DOUBLE
import godot.core.VariantType.LONG
import godot.core.VariantType.NIL
import godot.core.VariantType.PACKED_VECTOR2_ARRAY
import godot.core.memory.TransferContext
import kotlin.Boolean
import kotlin.Double
import kotlin.Float
import kotlin.Int
import kotlin.Long
import kotlin.Suppress
/**
* A node that provides a polygon shape to a [godot.CollisionObject2D] parent.
*
* A node that provides a thickened polygon shape (a prism) to a [godot.CollisionObject2D] parent and allows to edit it. The polygon can be concave or convex. This can give a detection shape to an [godot.Area2D] or turn [godot.PhysicsBody2D] into a solid object.
*
* **Warning:** A non-uniformly scaled [godot.CollisionShape2D] will likely not behave as expected. Make sure to keep its scale the same on all axes and adjust its shape resource instead.
*/
@GodotBaseType
public open class CollisionPolygon2D : Node2D() {
/**
* Collision build mode. Use one of the [enum BuildMode] constants.
*/
public var buildMode: BuildMode
get() {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr, ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_GET_BUILD_MODE,
LONG)
return CollisionPolygon2D.BuildMode.from(TransferContext.readReturnValue(LONG) as Long)
}
set(`value`) {
TransferContext.writeArguments(LONG to value.id)
TransferContext.callMethod(rawPtr, ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_SET_BUILD_MODE,
NIL)
}
/**
* The polygon's list of vertices. Each point will be connected to the next, and the final point will be connected to the first.
*
* **Warning:** The returned value is a clone of the [godot.PackedVector2Array], not a reference.
*/
public var polygon: PackedVector2Array
get() {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr, ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_GET_POLYGON,
PACKED_VECTOR2_ARRAY)
return (TransferContext.readReturnValue(PACKED_VECTOR2_ARRAY, false) as PackedVector2Array)
}
set(`value`) {
TransferContext.writeArguments(PACKED_VECTOR2_ARRAY to value)
TransferContext.callMethod(rawPtr, ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_SET_POLYGON,
NIL)
}
/**
* If `true`, no collisions will be detected.
*/
public var disabled: Boolean
get() {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr, ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_IS_DISABLED,
BOOL)
return (TransferContext.readReturnValue(BOOL, false) as Boolean)
}
set(`value`) {
TransferContext.writeArguments(BOOL to value)
TransferContext.callMethod(rawPtr, ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_SET_DISABLED,
NIL)
}
/**
* If `true`, only edges that face up, relative to [godot.CollisionPolygon2D]'s rotation, will collide with other objects.
*
* **Note:** This property has no effect if this [godot.CollisionPolygon2D] is a child of an [godot.Area2D] node.
*/
public var oneWayCollision: Boolean
get() {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_IS_ONE_WAY_COLLISION_ENABLED, BOOL)
return (TransferContext.readReturnValue(BOOL, false) as Boolean)
}
set(`value`) {
TransferContext.writeArguments(BOOL to value)
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_SET_ONE_WAY_COLLISION, NIL)
}
/**
* The margin used for one-way collision (in pixels). Higher values will make the shape thicker, and work better for colliders that enter the polygon at a high velocity.
*/
public var oneWayCollisionMargin: Float
get() {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_GET_ONE_WAY_COLLISION_MARGIN, DOUBLE)
return (TransferContext.readReturnValue(DOUBLE, false) as Double).toFloat()
}
set(`value`) {
TransferContext.writeArguments(DOUBLE to value.toDouble())
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_COLLISIONPOLYGON2D_SET_ONE_WAY_COLLISION_MARGIN, NIL)
}
public override fun new(scriptIndex: Int): Boolean {
callConstructor(ENGINECLASS_COLLISIONPOLYGON2D, scriptIndex)
return true
}
public enum class BuildMode(
id: Long,
) {
/**
* Collisions will include the polygon and its contained area. In this mode the node has the same effect as several [godot.ConvexPolygonShape2D] nodes, one for each convex shape in the convex decomposition of the polygon (but without the overhead of multiple nodes).
*/
BUILD_SOLIDS(0),
/**
* Collisions will only include the polygon edges. In this mode the node has the same effect as a single [godot.ConcavePolygonShape2D] made of segments, with the restriction that each segment (after the first one) starts where the previous one ends, and the last one ends where the first one starts (forming a closed but hollow polygon).
*/
BUILD_SEGMENTS(1),
;
public val id: Long
init {
this.id = id
}
public companion object {
public fun from(`value`: Long) = entries.single { it.id == `value` }
}
}
public companion object
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy