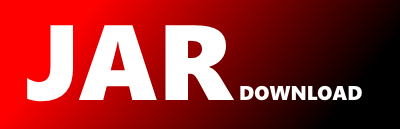
godot.gen.godot.PhysicsTestMotionResult2D.kt Maven / Gradle / Ivy
// THIS FILE IS GENERATED! DO NOT EDIT IT MANUALLY!
@file:Suppress("PackageDirectoryMismatch", "unused", "FunctionName", "RedundantModalityModifier",
"UNCHECKED_CAST", "JoinDeclarationAndAssignment", "USELESS_CAST",
"RemoveRedundantQualifierName", "NOTHING_TO_INLINE", "NON_FINAL_MEMBER_IN_OBJECT",
"RedundantVisibilityModifier", "RedundantUnitReturnType", "MemberVisibilityCanBePrivate")
package godot
import godot.`annotation`.GodotBaseType
import godot.core.RID
import godot.core.VariantType.DOUBLE
import godot.core.VariantType.LONG
import godot.core.VariantType.OBJECT
import godot.core.VariantType.VECTOR2
import godot.core.VariantType._RID
import godot.core.Vector2
import godot.core.memory.TransferContext
import kotlin.Boolean
import kotlin.Double
import kotlin.Float
import kotlin.Int
import kotlin.Long
import kotlin.Suppress
/**
* Describes the motion and collision result from [godot.PhysicsServer2D.bodyTestMotion].
*
* Describes the motion and collision result from [godot.PhysicsServer2D.bodyTestMotion].
*/
@GodotBaseType
public open class PhysicsTestMotionResult2D : RefCounted() {
public override fun new(scriptIndex: Int): Boolean {
callConstructor(ENGINECLASS_PHYSICSTESTMOTIONRESULT2D, scriptIndex)
return true
}
/**
* Returns the moving object's travel before collision.
*/
public fun getTravel(): Vector2 {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_TRAVEL, VECTOR2)
return (TransferContext.readReturnValue(VECTOR2, false) as Vector2)
}
/**
* Returns the moving object's remaining movement vector.
*/
public fun getRemainder(): Vector2 {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_REMAINDER, VECTOR2)
return (TransferContext.readReturnValue(VECTOR2, false) as Vector2)
}
/**
* Returns the point of collision in global coordinates, if a collision occurred.
*/
public fun getCollisionPoint(): Vector2 {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLISION_POINT, VECTOR2)
return (TransferContext.readReturnValue(VECTOR2, false) as Vector2)
}
/**
* Returns the colliding body's shape's normal at the point of collision, if a collision occurred.
*/
public fun getCollisionNormal(): Vector2 {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLISION_NORMAL, VECTOR2)
return (TransferContext.readReturnValue(VECTOR2, false) as Vector2)
}
/**
* Returns the colliding body's velocity, if a collision occurred.
*/
public fun getColliderVelocity(): Vector2 {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLIDER_VELOCITY, VECTOR2)
return (TransferContext.readReturnValue(VECTOR2, false) as Vector2)
}
/**
* Returns the unique instance ID of the colliding body's attached [godot.Object], if a collision occurred. See [godot.Object.getInstanceId].
*/
public fun getColliderId(): Long {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLIDER_ID, LONG)
return (TransferContext.readReturnValue(LONG, false) as Long)
}
/**
* Returns the colliding body's [RID] used by the [godot.PhysicsServer2D], if a collision occurred.
*/
public fun getColliderRid(): RID {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLIDER_RID, _RID)
return (TransferContext.readReturnValue(_RID, false) as RID)
}
/**
* Returns the colliding body's attached [godot.Object], if a collision occurred.
*/
public fun getCollider(): Object? {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLIDER, OBJECT)
return (TransferContext.readReturnValue(OBJECT, true) as Object?)
}
/**
* Returns the colliding body's shape index, if a collision occurred. See [godot.CollisionObject2D].
*/
public fun getColliderShape(): Int {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLIDER_SHAPE, LONG)
return (TransferContext.readReturnValue(LONG, false) as Long).toInt()
}
/**
* Returns the moving object's colliding shape, if a collision occurred.
*/
public fun getCollisionLocalShape(): Int {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLISION_LOCAL_SHAPE, LONG)
return (TransferContext.readReturnValue(LONG, false) as Long).toInt()
}
/**
* Returns the length of overlap along the collision normal, if a collision occurred.
*/
public fun getCollisionDepth(): Float {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLISION_DEPTH, DOUBLE)
return (TransferContext.readReturnValue(DOUBLE, false) as Double).toFloat()
}
/**
* Returns the maximum fraction of the motion that can occur without a collision, between `0` and `1`.
*/
public fun getCollisionSafeFraction(): Float {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLISION_SAFE_FRACTION, DOUBLE)
return (TransferContext.readReturnValue(DOUBLE, false) as Double).toFloat()
}
/**
* Returns the minimum fraction of the motion needed to collide, if a collision occurred, between `0` and `1`.
*/
public fun getCollisionUnsafeFraction(): Float {
TransferContext.writeArguments()
TransferContext.callMethod(rawPtr,
ENGINEMETHOD_ENGINECLASS_PHYSICSTESTMOTIONRESULT2D_GET_COLLISION_UNSAFE_FRACTION, DOUBLE)
return (TransferContext.readReturnValue(DOUBLE, false) as Double).toFloat()
}
public companion object
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy