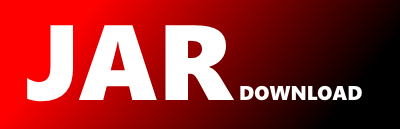
com.vaadin.addon.jpacontainer.BufferedContainerDelegate Maven / Gradle / Ivy
/*
JPAContainer
Copyright (C) 2009-2011 Oy Vaadin Ltd
This program is available under GNU Affero General Public License (version
3 or later at your option).
See the file licensing.txt distributed with this software for more
information about licensing.
You should have received a copy of the GNU Affero General Public License
along with this program. If not, see .
*/
package com.vaadin.addon.jpacontainer;
import com.vaadin.data.Buffered.SourceException;
import com.vaadin.data.Validator.InvalidValueException;
import java.io.Serializable;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
/**
* A delegate class used by {@link JPAContainer} to handle buffered changes.
* This class is not part of the public API and should not be used outside of
* JPAContainer.
*
* If the entity implements the {@link Cloneable} interface, clones of the entities
* will be stored instead of the entities themselves. This has the advantage of
* tracking exactly which changes have been made to an entity and in which order
* (e.g. if the same entity is modified twice before the changes are committed).
*
* @author Petter Holmström (Vaadin Ltd)
* @since 1.0
*/
final class BufferedContainerDelegate implements Serializable {
private static final long serialVersionUID = -4471665710680629463L;
/**
* Creates a new BufferedContainerDelegate
for the specified container.
*
* @param container the JPAContainer
(must not be null).
*/
BufferedContainerDelegate(JPAContainer container) {
assert container != null : "container must not be null";
this.container = container;
}
enum DeltaType {
ADD, UPDATE, DELETE
}
final class Delta implements Serializable {
private static final long serialVersionUID = -5907859901553818040L;
final DeltaType type;
final Object itemId;
final T entity;
Delta(DeltaType type, Object itemId, T entity) {
this.type = type;
this.itemId = itemId;
this.entity = entity;
}
}
private JPAContainer container;
// Delta list contains all changes
private List deltaList = new LinkedList();
// We need a list to maintain the order in which the items were added...
private List