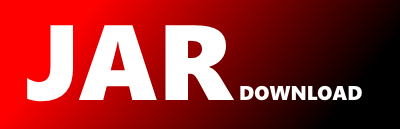
com.vaadin.addon.jpacontainer.EntityContainer Maven / Gradle / Ivy
/*
JPAContainer
Copyright (C) 2009-2011 Oy Vaadin Ltd
This program is available under GNU Affero General Public License (version
3 or later at your option).
See the file licensing.txt distributed with this software for more
information about licensing.
You should have received a copy of the GNU Affero General Public License
along with this program. If not, see .
*/
package com.vaadin.addon.jpacontainer;
import com.vaadin.addon.jpacontainer.filter.StringComparisonFilter;
import com.vaadin.data.Buffered;
import com.vaadin.data.Container;
import com.vaadin.data.Validator.InvalidValueException;
/**
* A Container for {@link EntityItem}s. The data is provided by a
* {@link EntityProvider}. Supports sorting, advanced filtering, nested
* properties and buffering.
*
* @author Petter Holmström (Vaadin Ltd)
* @since 1.0
*/
public interface EntityContainer extends Container.Sortable,
AdvancedFilterable, Container.ItemSetChangeNotifier,
Buffered, Container.Filterable {
/**
* Gets the entity provider that is used for fetching and storing entities.
*
* @return the entity provider, or null if this container has not yet been
* properly initialized.
*/
public EntityProvider getEntityProvider();
/**
* Sets the entity provider to use for fetching and storing entities. The EntityProvider
* can be changed once set, if necessary.
*
* @param entityProvider
* the entity provider to use (must not be null).
*/
public void setEntityProvider(EntityProvider entityProvider);
/**
* Gets the class of the entities that are/can be contained in this
* container.
*
* @return the entity class.
*/
public Class getEntityClass();
/**
* Adds the nested property nestedProperty
to the set of
* properties. An asterisk can be used as a wildcard to indicate all
* leaf-properties.
*
* For example, let's say there is a property named address
and
* that this property's type in turn has the properties street
,
* postalCode
and city
.
*
* If we want to be able to access the street property directly, we can add
* the nested property address.street
using this method.
*
* However, if we want to add all the address properties, we can also use
* address.*
. This will cause the nested properties
* address.street
, address.postalCode
and
* address.city
to be added to the set of properties.
*
* Note, that the wildcard cannot be used in the middle of a chain
* of property names. E.g. myprop.*.something
is illegal.
*
* Nested properties may be transient, but only persistent nested properties
* (i.e. embedded properties or ManyToOne references) may be used for filtering
* or sorting.
*
* @param nestedProperty
* the nested property to add (must not be null).
* @throws UnsupportedOperationException
* if nested properties are not supported by the container.
* @throws IllegalArgumentException if nestedProperty
is illegal.
*/
public void addNestedContainerProperty(String nestedProperty)
throws UnsupportedOperationException, IllegalArgumentException;
/**
* Adds a new entity to the container. The corresponding {@link EntityItem}
* can then be accessed by calling {@link #getItem(java.lang.Object) } using
* the entity identifier returned by this method.
*
* If {@link #isAutoCommit() } is activated, the returned identifier is
* always the actual entity ID. Otherwise, the returned identifier may,
* depending on the ID generation strategy, be either the actual entity ID
* or a temporary ID that is changed to the real ID once the changes have
* been committed using {@link #commit() }.
*
* @param entity
* the entity to add (must not be null).
* @return the identifier of the entity (never null).
* @throws UnsupportedOperationException
* if the container does not support adding new entities at all.
* @throws IllegalStateException
* if the container supports adding entities, but is currently
* in read only mode.
*/
public Object addEntity(T entity) throws UnsupportedOperationException,
IllegalStateException;
/**
* Creates a new {@link EntityItem} for entity
without adding
* it to the container. This makes it possible to use the same
* {@link com.vaadin.ui.Form} for editing both new entities and existing
* entities.
*
* To add the entity to the container, {@link #addEntity(java.lang.Object) }
* should be used.
*
* @see EntityItem#getItemId()
* @param entity
* the entity for which an item should be created.
* @return the entity item (never null).
*/
public EntityItem createEntityItem(T entity);
/**
* {@inheritDoc }
*/
public EntityItem getItem(Object itemId);
/**
* Returns whether the container is read only or writable.
*
* @return true if read only, false if writable.
*/
public boolean isReadOnly();
/**
* Changes the read only state of the container, if possible.
*
* @param readOnly
* true to make the container read only, false to make it
* writable.
* @throws UnsupportedOperationException
* if the read only state cannot be changed.
*/
public void setReadOnly(boolean readOnly)
throws UnsupportedOperationException;
/**
* Alias of {@link Buffered#setWriteThrough(boolean) }.
*/
public void setAutoCommit(boolean autoCommit) throws SourceException,
InvalidValueException;
/**
* Alias of {@link Buffered#isWriteThrough() }.
*/
public boolean isAutoCommit();
/**
* {@inheritDoc }
*
* This method creates a new {@link StringComparisonFilter} for the
* specified parameters and applies the filter immediately, regardless of
* the state of {@link #isApplyFiltersImmediately() }.
*
* @see #addFilter(com.vaadin.addon.jpacontainer.Filter)
* @see #applyFilters()
*/
public void addContainerFilter(Object propertyId, String filterString,
boolean ignoreCase, boolean onlyMatchPrefix);
/**
* {@inheritDoc}
*
* This method does the same as {@link #removeAllFilters() }, but the
* container is updated immediately regardless of the state of
* {@link #isApplyFiltersImmediately() }.
*
* @see #removeAllFilters()
*/
public void removeAllContainerFilters();
/**
* {@inheritDoc }
*
* The container is updated immediately regardless of the state of
* {@link #isApplyFiltersImmediately() }.
*
* @see #removeFilter(com.vaadin.addon.jpacontainer.Filter)
*/
public void removeContainerFilters(Object propertyId);
}