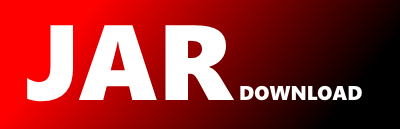
com.vaadin.addon.jpacontainer.EntityProvider Maven / Gradle / Ivy
/*
* JPAContainer
* Copyright (C) 2010 Oy IT Mill Ltd
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package com.vaadin.addon.jpacontainer;
import java.io.Serializable;
import java.util.List;
/**
* Like the name suggests, the purpose of the EntityProvider
is to
* provide entities to {@link EntityContainer}s. It basically contains a subset
* of the methods found in the standard {@link com.vaadin.data.Container} interface.
* Note, that most of the methods return entity IDs and not entity instances - only
* {@link #getEntity(java.lang.Object) } actually returns instances.
*
* Entity providers should at least implement this interface according to the
* contracts specified in the methods JavaDocs. Additional functionality may be
* added by also implementing e.g. {@link MutableEntityProvider}.
*
* Once implemented, the entity provider can be plugged into an entity container
* by using the {@link EntityContainer#setEntityProvider(com.vaadin.addon.jpacontainer.EntityProvider) } method.
*
* Please note the {@link #isEntitiesDetached() } flag, as this may have weird
* consequences if used inproperly.
*
* @see MutableEntityProvider
* @see CachingEntityProvider
* @see BatchableEntityProvider
* @see EntityProviderChangeNotifier
* @author Petter Holmström (IT Mill)
* @since 1.0
*/
public interface EntityProvider extends Serializable {
/**
* Loads the entity identified by entityId
from the persistence
* storage.
*
* @param entityId
* the entity identifier (must not be null).
* @return the entity, or null if not found.
*/
public T getEntity(Object entityId);
/**
* If this method returns true, all entities returned from this entity
* provider are explicitly detached from the persistence context before
* returned, regardless of whether the persistence context is extended or
* transaction-scoped. Thus, no lazy-loaded associations will work and any
* changes made to the entities will not be reflected in the persistence
* context unless the entity is merged.
*
* If this method returns false, the entities returned may be managed or
* detached, depending on the scope of the persistence context.
*
* The default value is implementation specific.
*
* @see #setEntitiesDetached(boolean)
*
* @return true if the entities are explicitly detached, false otherwise.
*/
public boolean isEntitiesDetached();
/**
* Specifies whether the entities returned by the entity provider should be
* explicitly detached or not. See {@link #isEntitiesDetached() } for a more
* detailed description of the consequences.
*
* @param detached
* true to request explicitly detached entities, false otherwise.
* @throws UnsupportedOperationException
* if the implementation does not allow the user to change the
* way entities are returned.
*/
public void setEntitiesDetached(boolean detached)
throws UnsupportedOperationException;
/**
* Gets the identifier of the entity at position index
in the
* result set determined from filter
and sortBy
.
*
* @param filter
* the filter that should be used to filter the entities (may be
* null).
* @param sortBy
* the properties to sort by, if any (may be null).
* @param index
* the index of the entity to fetch.
* @return the entity identifier, or null if not found.
*/
public Object getEntityIdentifierAt(Filter filter, List sortBy,
int index);
/**
* Gets the identifier of the first item in the list of entities determined
* by filter
and sortBy
.
*
* @param filter
* the filter that should be used to filter the entities (may be
* null).
* @param sortBy
* the properties to sort by, if any (may be null).
* @return the identifier of the first entity, or null if there are no
* entities matching filter
.
*/
public Object getFirstEntityIdentifier(Filter filter, List sortBy);
/**
* Gets the identifier of the last item in the list of entities determined
* by filter
and sortBy
.
*
* @param filter
* the filter that should be used to filter the entities (may be
* null).
* @param sortBy
* the properties to sort by, if any (may be null).
* @return the identifier of the last entity, or null if there are no
* entities matching filter
.
*/
public Object getLastEntityIdentifier(Filter filter, List sortBy);
/**
* Gets the identifier of the item next to the item identified by
* entityId
in the list of entities determined by
* filter
and sortBy
.
*
* @param filter
* the filter that should be used to filter the entities (may be
* null).
* @param sortBy
* the properties to sort by, if any (may be null).
* @return the identifier of the next entity, or null if there are no
* entities matching filter
or entityId
is
* the last item.
*/
public Object getNextEntityIdentifier(Object entityId, Filter filter,
List sortBy);
/**
* Gets the identifier of the item previous to the item identified by
* entityId
in the list of entities determined by
* filter
and sortBy
.
*
* @param filter
* the filter that should be used to filter the entities (may be
* null).
* @param sortBy
* the properties to sort by, if any (may be null).
* @return the identifier of the previous entity, or null if there are no
* entities matching filter
or entityId
is
* the first item.
*/
public Object getPreviousEntityIdentifier(Object entityId, Filter filter,
List sortBy);
/**
* Gets the identifiers of all items that match filter
. This
* method only exists to speed up
* {@link com.vaadin.data.Container#getItemIds() }, which in turn is used by
* {@link com.vaadin.ui.AbstractSelect} and its subclasses (e.g. ComboBox).
* Using this method is not recommended, as it does not use lazy loading.
*
* @param filter
* the filter that should be used to filter the entities (may be
* null).
* @param sortBy
* the properties to sort by, if any (may be null).
* @return an unmodifiable list of entity identifiers (never null).
*/
public List