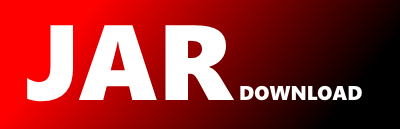
com.vaadin.polymer.iron.element.IronAjaxElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-ajax project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.element;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.core.client.js.JsProperty;
import com.google.gwt.core.client.js.JsType;
/**
* The iron-ajax
element exposes network request functionality.
* <iron-ajax
* auto
* url="http://gdata.youtube.com/feeds/api/videos/"
* params='{"alt":"json", "q":"chrome"}'
* handle-as="json"
* on-response="handleResponse"
* debounce-duration="300"></iron-ajax>
*
*
*
With auto
set to true
, the element performs a request whenever
its url
, params
or body
properties are changed. Automatically generated
requests will be debounced in the case that multiple attributes are changed
sequentially.
* Note: The params
attribute must be double quoted JSON.
* You can trigger a request explicitly by calling generateRequest
on the
element.
*/
@JsType
public interface IronAjaxElement extends HTMLElement {
public static final String TAG = "iron-ajax";
public static final String SRC = "iron-ajax/iron-ajax.html";
/**
* An Array of all in-flight requests originating from this iron-ajax
element.
*
* JavaScript Info:
* @property activeRequests
* @type Array
*
*/
@JsProperty JsArray getActiveRequests();
/**
* An Array of all in-flight requests originating from this iron-ajax
element.
*
* JavaScript Info:
* @property activeRequests
* @type Array
*
*/
@JsProperty void setActiveRequests(JsArray value);
/**
* If true, automatically performs an Ajax request when either url
or
params
changes.
*
* JavaScript Info:
* @property auto
* @type Boolean
*
*/
@JsProperty boolean getAuto();
/**
* If true, automatically performs an Ajax request when either url
or
params
changes.
*
* JavaScript Info:
* @property auto
* @type Boolean
*
*/
@JsProperty void setAuto(boolean value);
/**
* Optional raw body content to send when method === “POST”.
* Example:
* <iron-ajax method="POST" auto url="http://somesite.com"
* body='{"foo":1, "bar":2}'>
* </iron-ajax>
*
*
*
* JavaScript Info:
* @property body
* @type String
*
*/
@JsProperty String getBody();
/**
* Optional raw body content to send when method === “POST”.
* Example:
* <iron-ajax method="POST" auto url="http://somesite.com"
* body='{"foo":1, "bar":2}'>
* </iron-ajax>
*
*
*
* JavaScript Info:
* @property body
* @type String
*
*/
@JsProperty void setBody(String value);
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
@JsProperty String getContentType();
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
@JsProperty void setContentType(String value);
/**
* Length of time in milliseconds to debounce multiple requests.
*
* JavaScript Info:
* @property debounceDuration
* @type Number
*
*/
@JsProperty double getDebounceDuration();
/**
* Length of time in milliseconds to debounce multiple requests.
*
* JavaScript Info:
* @property debounceDuration
* @type Number
*
*/
@JsProperty void setDebounceDuration(double value);
/**
* Performs an AJAX request to the specified URL.
*
* JavaScript Info:
* @method generateRequest
*
*/
void generateRequest();
/**
* Specifies what data to store in the response
property, and
to deliver as event.response
in response
events.
* One of:
* text
: uses XHR.responseText
.
* xml
: uses XHR.responseXML
.
* json
: uses XHR.responseText
parsed as JSON.
* arraybuffer
: uses XHR.response
.
* blob
: uses XHR.response
.
* document
: uses XHR.response
.
*
* JavaScript Info:
* @property handleAs
* @type String
*
*/
@JsProperty String getHandleAs();
/**
* Specifies what data to store in the response
property, and
to deliver as event.response
in response
events.
* One of:
* text
: uses XHR.responseText
.
* xml
: uses XHR.responseXML
.
* json
: uses XHR.responseText
parsed as JSON.
* arraybuffer
: uses XHR.response
.
* blob
: uses XHR.response
.
* document
: uses XHR.response
.
*
* JavaScript Info:
* @property handleAs
* @type String
*
*/
@JsProperty void setHandleAs(String value);
/**
* HTTP request headers to send.
* Example:
* <iron-ajax
* auto
* url="http://somesite.com"
* headers='{"X-Requested-With": "XMLHttpRequest"}'
* handle-as="json"
* last-response-changed="{{handleResponse}}"></iron-ajax>
*
*
*
Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty JavaScriptObject getHeaders();
/**
* HTTP request headers to send.
* Example:
* <iron-ajax
* auto
* url="http://somesite.com"
* headers='{"X-Requested-With": "XMLHttpRequest"}'
* handle-as="json"
* last-response-changed="{{handleResponse}}"></iron-ajax>
*
*
*
Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty void setHeaders(JavaScriptObject value);
/**
* Will be set to the most recent error that resulted from a request
that originated from this iron-ajax element.
*
* JavaScript Info:
* @property lastError
* @type Object
*
*/
@JsProperty JavaScriptObject getLastError();
/**
* Will be set to the most recent error that resulted from a request
that originated from this iron-ajax element.
*
* JavaScript Info:
* @property lastError
* @type Object
*
*/
@JsProperty void setLastError(JavaScriptObject value);
/**
* Will be set to the most recent request made by this iron-ajax element.
*
* JavaScript Info:
* @property lastRequest
* @type Object
*
*/
@JsProperty JavaScriptObject getLastRequest();
/**
* Will be set to the most recent request made by this iron-ajax element.
*
* JavaScript Info:
* @property lastRequest
* @type Object
*
*/
@JsProperty void setLastRequest(JavaScriptObject value);
/**
* Will be set to the most recent response received by a request
that originated from this iron-ajax element. The type of the response
is determined by the value of handleAs
at the time that the request
was generated.
*
* JavaScript Info:
* @property lastResponse
* @type Object
*
*/
@JsProperty JavaScriptObject getLastResponse();
/**
* Will be set to the most recent response received by a request
that originated from this iron-ajax element. The type of the response
is determined by the value of handleAs
at the time that the request
was generated.
*
* JavaScript Info:
* @property lastResponse
* @type Object
*
*/
@JsProperty void setLastResponse(JavaScriptObject value);
/**
* Will be set to true if there is at least one in-flight request
associated with this iron-ajax element.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
@JsProperty boolean getLoading();
/**
* Will be set to true if there is at least one in-flight request
associated with this iron-ajax element.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
@JsProperty void setLoading(boolean value);
/**
* The HTTP method to use such as ‘GET’, ‘POST’, ‘PUT’, or ‘DELETE’.
Default is ‘GET’.
*
* JavaScript Info:
* @property method
* @type String
*
*/
@JsProperty String getMethod();
/**
* The HTTP method to use such as ‘GET’, ‘POST’, ‘PUT’, or ‘DELETE’.
Default is ‘GET’.
*
* JavaScript Info:
* @property method
* @type String
*
*/
@JsProperty void setMethod(String value);
/**
* An object that contains query parameters to be appended to the
specified url
when generating a request. If you wish to set the body
content when making a POST request, you should use the body
property
instead.
*
* JavaScript Info:
* @property params
* @type Object
*
*/
@JsProperty JavaScriptObject getParams();
/**
* An object that contains query parameters to be appended to the
specified url
when generating a request. If you wish to set the body
content when making a POST request, you should use the body
property
instead.
*
* JavaScript Info:
* @property params
* @type Object
*
*/
@JsProperty void setParams(JavaScriptObject value);
/**
* The query string that should be appended to the url
, serialized from
the current value of params
.
*
* JavaScript Info:
* @method queryString
*
*/
void queryString();
/**
* An object that maps header names to header values, first applying the
the value of Content-Type
and then overlaying the headers specified
in the headers
property.
*
* JavaScript Info:
* @method requestHeaders
*
*/
void requestHeaders();
/**
* The url
with query string (if params
are specified), suitable for
providing to an iron-request
instance.
*
* JavaScript Info:
* @method requestUrl
*
*/
void requestUrl();
/**
* Toggle whether XHR is synchronous or asynchronous. Don’t change this
to true unless You Know What You Are Doing™.
*
* JavaScript Info:
* @property sync
* @type Boolean
*
*/
@JsProperty boolean getSync();
/**
* Toggle whether XHR is synchronous or asynchronous. Don’t change this
to true unless You Know What You Are Doing™.
*
* JavaScript Info:
* @property sync
* @type Boolean
*
*/
@JsProperty void setSync(boolean value);
/**
* Request options suitable for generating an iron-request
instance based
on the current state of the iron-ajax
instance’s properties.
*
* JavaScript Info:
* @method toRequestOptions
*
*/
void toRequestOptions();
/**
* The URL target of the request.
*
* JavaScript Info:
* @property url
* @type String
*
*/
@JsProperty String getUrl();
/**
* The URL target of the request.
*
* JavaScript Info:
* @property url
* @type String
*
*/
@JsProperty void setUrl(String value);
/**
* If true, error messages will automatically be logged to the console.
*
* JavaScript Info:
* @property verbose
* @type Boolean
*
*/
@JsProperty boolean getVerbose();
/**
* If true, error messages will automatically be logged to the console.
*
* JavaScript Info:
* @property verbose
* @type Boolean
*
*/
@JsProperty void setVerbose(boolean value);
/**
* Set the withCredentials flag on the request.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
@JsProperty boolean getWithCredentials();
/**
* Set the withCredentials flag on the request.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
@JsProperty void setWithCredentials(boolean value);
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
@JsProperty JsArray getObservers();
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
@JsProperty void setObservers(JsArray value);
}