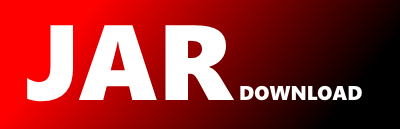
com.vaadin.polymer.iron.widget.IronA11yKeys Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-a11y-keys project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.element.*;
import com.vaadin.polymer.keys.widget.event.KeysPressedEvent;
import com.vaadin.polymer.keys.widget.event.KeysPressedEventHandler;
import com.vaadin.polymer.PolymerWidget;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* iron-a11y-keys
provides a normalized interface for processing keyboard commands that pertain to WAI-ARIA best
practices. The element takes care of browser differences
with respect to Keyboard events and uses an expressive syntax to filter key presses.
* Use the keys
attribute to express what combination of keys will trigger the event to fire.
* Use the target
attribute to set up event handlers on a specific node.
The keys-pressed
event will fire when one of the key combinations set with the keys
attribute is pressed.
* Example:
* This element will call arrowHandler
on all arrow keys:
* <iron-a11y-keys target="{{}}" keys="up down left right" on-keys-pressed="{{arrowHandler}}"></iron-a11y-keys>
*
*
*
Keys Syntax:
* The keys
attribute can accepts a space seprated, +
concatenated set of modifier keys and some common keyboard keys.
* The common keys are a-z
, 0-9
(top row and number pad), *
(shift 8 and number pad), F1-F12
, Page Up
, Page
* Down
, Left Arrow
, Right Arrow
, Down Arrow
, Up Arrow
, Home
, End
, Escape
, Space
, Tab
, and Enter
keys.
* The modifier keys are Shift
, Control
, and Alt
.
* All keys are expected to be lowercase and shortened:
Left Arrow
is left
, Page Down
is pagedown
, Control
is ctrl
, F1
is f1
, Escape
is esc
etc.
* Keys Syntax Example:
* Given the keys
attribute value “ctrl+shift+f7 up pagedown esc space alt+m”, the <iron-a11y-keys>
element will send
the keys-pressed
event if any of the follow key combos are pressed: Control and Shift and F7 keys, Up Arrow key, Page
Down key, Escape key, Space key, Alt and M key.
* Slider Example:
* The following is an example of the set of keys that fulfil the WAI-ARIA “slider” role best
practices:
* <iron-a11y-keys target="{{}}" keys="left pagedown down" on-keys-pressed="{{decrement}}"></iron-a11y-keys>
* <iron-a11y-keys target="{{}}" keys="right pageup up" on-keys-pressed="{{increment}}"></iron-a11y-keys>
* <iron-a11y-keys target="{{}}" keys="home" on-keys-pressed="{{setMin}}"></iron-a11y-keys>
* <iron-a11y-keys target="{{}}" keys="end" on-keys-pressed="{{setMax}}"></iron-a11y-keys>
*
*
*
The increment
function will move the slider a set amount toward the maximum value.
The decrement
function will move the slider a set amount toward the minimum value.
The setMin
function will move the slider to the minimum value.
The setMax
function will move the slider to the maximum value.
* Keys Syntax Grammar:
* EBNF Grammar of the keys
attribute.
* modifier = "shift" | "ctrl" | "alt";
* ascii = ? /[a-z0-9]/ ? ;
* fnkey = ? f1 through f12 ? ;
* arrow = "up" | "down" | "left" | "right" ;
* key = "tab" | "esc" | "space" | "*" | "pageup" | "pagedown" | "home" | "end" | arrow | ascii | fnkey ;
* keycombo = { modifier, "+" }, key ;
* keys = keycombo, { " ", keycombo } ;
*
*
*
*/
public class IronA11yKeys extends PolymerWidget {
/**
* Default Constructor.
*/
public IronA11yKeys() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronA11yKeys(String html) {
super(IronA11yKeysElement.TAG, IronA11yKeysElement.SRC, html);
getPolymerElement().addEventListener(
com.vaadin.polymer.keys.element.event.KeysPressedEvent.NAME,
new com.vaadin.polymer.keys.element.event.KeysPressedEvent.Listener() {
@Override
protected void handleEvent(com.vaadin.polymer.keys.element.event.KeysPressedEvent event) {
fireEvent(new KeysPressedEvent(event));
}
});
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronA11yKeysElement getPolymerElement() {
return (IronA11yKeysElement) getElement();
}
/**
*
*
* JavaScript Info:
* @property keys
* @type String
*
*/
public String getKeys(){
return getPolymerElement().getKeys();
}
/**
*
*
* JavaScript Info:
* @property keys
* @type String
*
*/
public void setKeys(String value) {
getPolymerElement().setKeys(value);
}
/**
*
*
* JavaScript Info:
* @property target
* @type ?Node
*
*/
public JavaScriptObject getTarget(){
return getPolymerElement().getTarget();
}
/**
*
*
* JavaScript Info:
* @property target
* @type ?Node
*
*/
public void setTarget(JavaScriptObject value) {
getPolymerElement().setTarget(value);
}
/**
*
*
* JavaScript Info:
* @attribute target
*
*/
public void setTarget(String value) {
getPolymerElement().setAttribute("target", value);
}
/**
*
*
* JavaScript Info:
* @method attached
*
*/
public void attached() {
getPolymerElement().attached();
}
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior PaperTabs
*/
public void addOwnKeyBinding(JavaScriptObject eventString, JavaScriptObject handlerName) {
getPolymerElement().addOwnKeyBinding(eventString, handlerName);
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTabs
*/
public JavaScriptObject getKeyBindings(){
return getPolymerElement().getKeyBindings();
}
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTabs
*/
public void setKeyBindings(JavaScriptObject value) {
getPolymerElement().setKeyBindings(value);
}
/**
* The HTMLElement that will be firing relevant KeyboardEvents.
*
* JavaScript Info:
* @property keyEventTarget
* @type Object
* @behavior PaperTabs
*/
public JavaScriptObject getKeyEventTarget(){
return getPolymerElement().getKeyEventTarget();
}
/**
* The HTMLElement that will be firing relevant KeyboardEvents.
*
* JavaScript Info:
* @property keyEventTarget
* @type Object
* @behavior PaperTabs
*/
public void setKeyEventTarget(JavaScriptObject value) {
getPolymerElement().setKeyEventTarget(value);
}
/**
* The HTMLElement that will be firing relevant KeyboardEvents.
*
* JavaScript Info:
* @attribute key-event-target
* @behavior PaperTabs
*/
public void setKeyEventTarget(String value) {
getPolymerElement().setAttribute("key-event-target", value);
}
/**
*
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {} event
* @param {} eventString
* @behavior PaperTabs
*/
public void keyboardEventMatchesKeys(JavaScriptObject event, JavaScriptObject eventString) {
getPolymerElement().keyboardEventMatchesKeys(event, eventString);
}
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior PaperTabs
*/
public void removeOwnKeyBindings() {
getPolymerElement().removeOwnKeyBindings();
}
/**
*
*
* JavaScript Info:
* @method detached
* @behavior PaperTabs
*/
public void detached() {
getPolymerElement().detached();
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperTabs
*/
public JsArray getObservers(){
return getPolymerElement().getObservers();
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperTabs
*/
public void setObservers(JsArray value) {
getPolymerElement().setObservers(value);
}
/**
*
*
* JavaScript Info:
* @method registered
* @behavior PaperTabs
*/
public void registered() {
getPolymerElement().registered();
}
/**
*
*
* JavaScript Info:
* @event keys-pressed
*/
public HandlerRegistration addKeysPressedHandler(KeysPressedEventHandler handler) {
return addHandler(handler, KeysPressedEvent.TYPE);
}
}