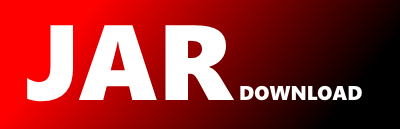
com.vaadin.polymer.neon.widget.NeonAnimatedPages Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from neon-animation project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.neon.widget;
import com.vaadin.polymer.neon.element.*;
import com.vaadin.polymer.PolymerWidget;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* neon-animated-pages
manages a set of pages and runs an animation when switching between them. Its
children pages should implement Polymer.NeonAnimatableBehavior
and define entry
and exit
animations to be run when switching to or switching out of the page.
*/
public class NeonAnimatedPages extends PolymerWidget {
/**
* Default Constructor.
*/
public NeonAnimatedPages() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public NeonAnimatedPages(String html) {
super(NeonAnimatedPagesElement.TAG, NeonAnimatedPagesElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public NeonAnimatedPagesElement getPolymerElement() {
return (NeonAnimatedPagesElement) getElement();
}
/**
*
*
* JavaScript Info:
* @property activateEvent
* @type String
*
*/
public String getActivateEvent(){
return getPolymerElement().getActivateEvent();
}
/**
*
*
* JavaScript Info:
* @property activateEvent
* @type String
*
*/
public void setActivateEvent(String value) {
getPolymerElement().setActivateEvent(value);
}
/**
* if true, the initial page selection will also be animated according to its animation config.
*
* JavaScript Info:
* @property animateInitialSelection
* @type Boolean
*
*/
public boolean getAnimateInitialSelection(){
return getPolymerElement().getAnimateInitialSelection();
}
/**
* if true, the initial page selection will also be animated according to its animation config.
*
* JavaScript Info:
* @property animateInitialSelection
* @type Boolean
*
*/
public void setAnimateInitialSelection(boolean value) {
getPolymerElement().setAnimateInitialSelection(value);
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
public JavaScriptObject getListeners(){
return getPolymerElement().getListeners();
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
public void setListeners(JavaScriptObject value) {
getPolymerElement().setListeners(value);
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public JsArray getObservers(){
return getPolymerElement().getObservers();
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public void setObservers(JsArray value) {
getPolymerElement().setObservers(value);
}
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior NeonAnimatedPages
*/
public void assignParentResizable(JavaScriptObject parentResizable) {
getPolymerElement().assignParentResizable(parentResizable);
}
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior NeonAnimatedPages
*/
public void notifyResize() {
getPolymerElement().notifyResize();
}
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior NeonAnimatedPages
*/
public void resizerShouldNotify(JavaScriptObject element) {
getPolymerElement().resizerShouldNotify(element);
}
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior NeonAnimatedPages
*/
public void stopResizeNotificationsFor(JavaScriptObject target) {
getPolymerElement().stopResizeNotificationsFor(target);
}
/**
*
*
* JavaScript Info:
* @method attached
* @behavior NeonAnimatedPages
*/
public void attached() {
getPolymerElement().attached();
}
/**
*
*
* JavaScript Info:
* @method detached
* @behavior NeonAnimatedPages
*/
public void detached() {
getPolymerElement().detached();
}
/**
* If you want to use the attribute value of an element for selected
instead of the index,
set this to the name of the attribute.
*
* JavaScript Info:
* @property attrForSelected
* @type string
* @behavior NeonAnimatedPages
*/
public String getAttrForSelected(){
return getPolymerElement().getAttrForSelected();
}
/**
* If you want to use the attribute value of an element for selected
instead of the index,
set this to the name of the attribute.
*
* JavaScript Info:
* @property attrForSelected
* @type string
* @behavior NeonAnimatedPages
*/
public void setAttrForSelected(String value) {
getPolymerElement().setAttrForSelected(value);
}
/**
*
*
* JavaScript Info:
* @property excludedLocalNames
* @type Object
* @behavior NeonAnimatedPages
*/
public JavaScriptObject getExcludedLocalNames(){
return getPolymerElement().getExcludedLocalNames();
}
/**
*
*
* JavaScript Info:
* @property excludedLocalNames
* @type Object
* @behavior NeonAnimatedPages
*/
public void setExcludedLocalNames(JavaScriptObject value) {
getPolymerElement().setExcludedLocalNames(value);
}
/**
* Returns the index of the given item.
*
* JavaScript Info:
* @method indexOf
* @param {Object} item
* @behavior NeonAnimatedPages
*/
public void indexOf(JavaScriptObject item) {
getPolymerElement().indexOf(item);
}
/**
* Returns an array of selectable items.
*
* JavaScript Info:
* @method items
* @behavior NeonAnimatedPages
*/
public void items() {
getPolymerElement().items();
}
/**
* Selects the given value.
*
* JavaScript Info:
* @method select
* @param {string} value
* @behavior NeonAnimatedPages
*/
public void select(String value) {
getPolymerElement().select(value);
}
/**
* Selects the next item.
*
* JavaScript Info:
* @method selectNext
* @behavior NeonAnimatedPages
*/
public void selectNext() {
getPolymerElement().selectNext();
}
/**
* Selects the previous item.
*
* JavaScript Info:
* @method selectPrevious
* @behavior NeonAnimatedPages
*/
public void selectPrevious() {
getPolymerElement().selectPrevious();
}
/**
* This is a CSS selector sting. If this is set, only items that matches the CSS selector
are selectable.
*
* JavaScript Info:
* @property selectable
* @type string
* @behavior NeonAnimatedPages
*/
public String getSelectable(){
return getPolymerElement().getSelectable();
}
/**
* This is a CSS selector sting. If this is set, only items that matches the CSS selector
are selectable.
*
* JavaScript Info:
* @property selectable
* @type string
* @behavior NeonAnimatedPages
*/
public void setSelectable(String value) {
getPolymerElement().setSelectable(value);
}
/**
* Gets or sets the selected element. The default is to use the index of the item.
*
* JavaScript Info:
* @property selected
* @type string
* @behavior NeonAnimatedPages
*/
public String getSelected(){
return getPolymerElement().getSelected();
}
/**
* Gets or sets the selected element. The default is to use the index of the item.
*
* JavaScript Info:
* @property selected
* @type string
* @behavior NeonAnimatedPages
*/
public void setSelected(String value) {
getPolymerElement().setSelected(value);
}
/**
* The attribute to set on elements when selected.
*
* JavaScript Info:
* @property selectedAttribute
* @type string
* @behavior NeonAnimatedPages
*/
public String getSelectedAttribute(){
return getPolymerElement().getSelectedAttribute();
}
/**
* The attribute to set on elements when selected.
*
* JavaScript Info:
* @property selectedAttribute
* @type string
* @behavior NeonAnimatedPages
*/
public void setSelectedAttribute(String value) {
getPolymerElement().setSelectedAttribute(value);
}
/**
* The class to set on elements when selected.
*
* JavaScript Info:
* @property selectedClass
* @type string
* @behavior NeonAnimatedPages
*/
public String getSelectedClass(){
return getPolymerElement().getSelectedClass();
}
/**
* The class to set on elements when selected.
*
* JavaScript Info:
* @property selectedClass
* @type string
* @behavior NeonAnimatedPages
*/
public void setSelectedClass(String value) {
getPolymerElement().setSelectedClass(value);
}
/**
* Returns the currently selected item.
*
* JavaScript Info:
* @property selectedItem
* @type Object
* @behavior NeonAnimatedPages
*/
public JavaScriptObject getSelectedItem(){
return getPolymerElement().getSelectedItem();
}
/**
* Returns the currently selected item.
*
* JavaScript Info:
* @property selectedItem
* @type Object
* @behavior NeonAnimatedPages
*/
public void setSelectedItem(JavaScriptObject value) {
getPolymerElement().setSelectedItem(value);
}
/**
* Returns the currently selected item.
*
* JavaScript Info:
* @attribute selected-item
* @behavior NeonAnimatedPages
*/
public void setSelectedItem(String value) {
getPolymerElement().setAttribute("selected-item", value);
}
/**
* Cancels the currently running animation.
*
* JavaScript Info:
* @method cancelAnimation
* @behavior NeonAnimatedPages
*/
public void cancelAnimation() {
getPolymerElement().cancelAnimation();
}
/**
* Plays an animation with an optional type
.
*
* JavaScript Info:
* @method playAnimation
* @param {string=} type
* @param {!Object=} cookie
* @behavior NeonAnimatedPages
*/
public void playAnimation(JavaScriptObject type, JavaScriptObject cookie) {
getPolymerElement().playAnimation(type, cookie);
}
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior NeonAnimatable
*/
public JavaScriptObject getAnimationConfig(){
return getPolymerElement().getAnimationConfig();
}
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior NeonAnimatable
*/
public void setAnimationConfig(JavaScriptObject value) {
getPolymerElement().setAnimationConfig(value);
}
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @attribute animation-config
* @behavior NeonAnimatable
*/
public void setAnimationConfig(String value) {
getPolymerElement().setAttribute("animation-config", value);
}
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior NeonAnimatable
*/
public String getEntryAnimation(){
return getPolymerElement().getEntryAnimation();
}
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior NeonAnimatable
*/
public void setEntryAnimation(String value) {
getPolymerElement().setEntryAnimation(value);
}
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior NeonAnimatable
*/
public String getExitAnimation(){
return getPolymerElement().getExitAnimation();
}
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior NeonAnimatable
*/
public void setExitAnimation(String value) {
getPolymerElement().setExitAnimation(value);
}
/**
* An element implementing Polymer.NeonAnimationRunnerBehavior
calls this method to configure
an animation with an optional type. Elements implementing Polymer.NeonAnimatableBehavior
should define the property animationConfig
, which is either a configuration object
or a map of animation type to array of configuration objects.
*
* JavaScript Info:
* @method getAnimationConfig
* @param {} type
* @behavior NeonAnimatable
*/
public void getAnimationConfig(JavaScriptObject type) {
getPolymerElement().getAnimationConfig(type);
}
}