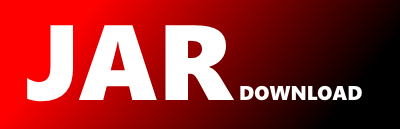
com.vaadin.polymer.paper.element.PaperDialogElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-dialog project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.element;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.core.client.js.JsProperty;
import com.google.gwt.core.client.js.JsType;
/**
* <paper-dialog>
is a dialog with Material Design styling and optional animations when it is
opened or closed. It provides styles for a header, content area, and an action area for buttons.
You can use the <paper-dialog-scrollable
element (in its own repository) if you need a scrolling
content area. See Polymer.PaperDialogBehavior
for specifics.
* For example, the following code implements a dialog with a header, scrolling content area and
buttons.
* <paper-dialog>
* <h2>Header</h2>
* <paper-dialog-scrollable>
* Lorem ipsum...
* </paper-dialog-scrollable>
* <div class="buttons">
* <paper-button dialog-dismiss>Cancel</paper-button>
* <paper-button dialog-confirm>Accept</paper-button>
* </div>
* </paper-dialog>
*
*
*
Styling
* See the docs for Polymer.PaperDialogBehavior
for the custom properties available for styling
this element.
* Animations
* Set the entry-animation
and/or exit-animation
attributes to add an animation when the dialog
is opened or closed. See the documentation in
PolymerElements/neon-animation for more info.
* For example:
* <link rel="import" href="components/neon-animation/animations/scale-up-animation.html">
* <link rel="import" href="components/neon-animation/animations/fade-out-animation.html">
*
* <paper-dialog entry-animation="scale-up-animation"
* exit-animation="fade-out-animation">
* <h2>Header</h2>
* <div>Dialog body</div>
* </paper-dialog>
*
*
*
Accessibility
* See the docs for Polymer.PaperDialogBehavior
for accessibility features implemented by this
element.
*/
@JsType
public interface PaperDialogElement extends HTMLElement {
public static final String TAG = "paper-dialog";
public static final String SRC = "paper-dialog/paper-dialog.html";
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
@JsProperty JavaScriptObject getListeners();
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
@JsProperty void setListeners(JavaScriptObject value);
/**
* If modal
is true, this implies no-cancel-on-outside-click
and with-backdrop
.
*
* JavaScript Info:
* @property modal
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getModal();
/**
* If modal
is true, this implies no-cancel-on-outside-click
and with-backdrop
.
*
* JavaScript Info:
* @property modal
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setModal(boolean value);
/**
*
*
* JavaScript Info:
* @method attached
* @behavior PaperDialog
*/
void attached();
/**
*
*
* JavaScript Info:
* @method detached
* @behavior PaperDialog
*/
void detached();
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
* @behavior PaperDialog
*/
@JsProperty JavaScriptObject getHostAttributes();
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
* @behavior PaperDialog
*/
@JsProperty void setHostAttributes(JavaScriptObject value);
/**
* The backdrop element.
*
* JavaScript Info:
* @method backdropElement
* @behavior PaperDialog
*/
void backdropElement();
/**
* Cancels the overlay.
*
* JavaScript Info:
* @method cancel
* @behavior PaperDialog
*/
void cancel();
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getCanceled();
/**
* True if the overlay was canceled when it was last closed.
*
* JavaScript Info:
* @property canceled
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setCanceled(boolean value);
/**
* Close the overlay.
*
* JavaScript Info:
* @method close
* @behavior PaperDialog
*/
void close();
/**
* Returns the reason this dialog was last closed.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior PaperDialog
*/
@JsProperty JavaScriptObject getClosingReason();
/**
* Returns the reason this dialog was last closed.
*
* JavaScript Info:
* @property closingReason
* @type Object
* @behavior PaperDialog
*/
@JsProperty void setClosingReason(JavaScriptObject value);
/**
* Set to true to disable auto-focusing the overlay or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getNoAutoFocus();
/**
* Set to true to disable auto-focusing the overlay or child nodes with
the autofocus
attribute` when the overlay is opened.
*
* JavaScript Info:
* @property noAutoFocus
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setNoAutoFocus(boolean value);
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getNoCancelOnEscKey();
/**
* Set to true to disable canceling the overlay with the ESC key.
*
* JavaScript Info:
* @property noCancelOnEscKey
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setNoCancelOnEscKey(boolean value);
/**
* Set to true to disable canceling the overlay by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getNoCancelOnOutsideClick();
/**
* Set to true to disable canceling the overlay by clicking outside it.
*
* JavaScript Info:
* @property noCancelOnOutsideClick
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setNoCancelOnOutsideClick(boolean value);
/**
* Open the overlay.
*
* JavaScript Info:
* @method open
* @behavior PaperDialog
*/
void open();
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getOpened();
/**
* True if the overlay is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setOpened(boolean value);
/**
* Toggle the opened state of the overlay.
*
* JavaScript Info:
* @method toggle
* @behavior PaperDialog
*/
void toggle();
/**
* Set to true to display a backdrop behind the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getWithBackdrop();
/**
* Set to true to display a backdrop behind the overlay.
*
* JavaScript Info:
* @property withBackdrop
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setWithBackdrop(boolean value);
/**
*
*
* JavaScript Info:
* @method registered
* @behavior PaperDialog
*/
void registered();
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty boolean getAutoFitOnAttach();
/**
* Set to true to auto-fit on attach.
*
* JavaScript Info:
* @property autoFitOnAttach
* @type Boolean
* @behavior PaperDialog
*/
@JsProperty void setAutoFitOnAttach(boolean value);
/**
* Centers horizontally and vertically if not already positioned. This also sets
position:fixed
.
*
* JavaScript Info:
* @method center
* @behavior PaperDialog
*/
void center();
/**
* Constrains the size of the element to the window or fitInfo
by setting max-height
and/or max-width
.
*
* JavaScript Info:
* @method constrain
* @behavior PaperDialog
*/
void constrain();
/**
* Fits and optionally centers the element into the window, or fitInfo
if specified.
*
* JavaScript Info:
* @method fit
* @behavior PaperDialog
*/
void fit();
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior PaperDialog
*/
@JsProperty JavaScriptObject getFitInto();
/**
* The element to fit this
into.
*
* JavaScript Info:
* @property fitInto
* @type Object
* @behavior PaperDialog
*/
@JsProperty void setFitInto(JavaScriptObject value);
/**
* Equivalent to calling resetFit()
and fit()
. Useful to call this after the element,
the window, or the fitInfo
element has been resized.
*
* JavaScript Info:
* @method refit
* @behavior PaperDialog
*/
void refit();
/**
* Resets the target element’s position and size constraints, and clear
the memoized data.
*
* JavaScript Info:
* @method resetFit
* @behavior PaperDialog
*/
void resetFit();
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior PaperDialog
*/
@JsProperty JavaScriptObject getSizingTarget();
/**
* The element that will receive a max-height
/width
. By default it is the same as this
,
but it can be set to a child element. This is useful, for example, for implementing a
scrolling region inside the element.
*
* JavaScript Info:
* @property sizingTarget
* @type !Element
* @behavior PaperDialog
*/
@JsProperty void setSizingTarget(JavaScriptObject value);
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior NeonAnimatedPages
*/
void assignParentResizable(JavaScriptObject parentResizable);
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior NeonAnimatedPages
*/
void notifyResize();
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior NeonAnimatedPages
*/
void resizerShouldNotify(JavaScriptObject element);
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior NeonAnimatedPages
*/
void stopResizeNotificationsFor(JavaScriptObject target);
/**
* Cancels the currently running animation.
*
* JavaScript Info:
* @method cancelAnimation
* @behavior NeonAnimatedPages
*/
void cancelAnimation();
/**
* Plays an animation with an optional type
.
*
* JavaScript Info:
* @method playAnimation
* @param {string=} type
* @param {!Object=} cookie
* @behavior NeonAnimatedPages
*/
void playAnimation(JavaScriptObject type, JavaScriptObject cookie);
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior NeonAnimatable
*/
@JsProperty JavaScriptObject getAnimationConfig();
/**
* Animation configuration. See README for more info.
*
* JavaScript Info:
* @property animationConfig
* @type Object
* @behavior NeonAnimatable
*/
@JsProperty void setAnimationConfig(JavaScriptObject value);
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior NeonAnimatable
*/
@JsProperty String getEntryAnimation();
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior NeonAnimatable
*/
@JsProperty void setEntryAnimation(String value);
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior NeonAnimatable
*/
@JsProperty String getExitAnimation();
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior NeonAnimatable
*/
@JsProperty void setExitAnimation(String value);
/**
* An element implementing Polymer.NeonAnimationRunnerBehavior
calls this method to configure
an animation with an optional type. Elements implementing Polymer.NeonAnimatableBehavior
should define the property animationConfig
, which is either a configuration object
or a map of animation type to array of configuration objects.
*
* JavaScript Info:
* @method getAnimationConfig
* @param {} type
* @behavior NeonAnimatable
*/
void getAnimationConfig(JavaScriptObject type);
}