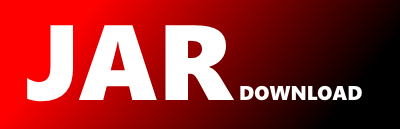
com.vaadin.polymer.paper.element.PaperMenuElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-menu project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.element;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.core.client.js.JsProperty;
import com.google.gwt.core.client.js.JsType;
/**
* <paper-menu>
implements an accessible menu control with Material Design styling. The focused item
is highlighted, and the selected item has bolded text.
* <paper-menu>
* <paper-item>Item 1</paper-item>
* <paper-item>Item 2</paper-item>
* </paper-menu>
*
*
*
Make a multi-select menu with the multi
attribute. Items in a multi-select menu can be deselected,
and multiple item can be selected.
* <paper-menu multi>
* <paper-item>Item 1</paper-item>
* <paper-item>Item 2</paper-item>
* </paper-menu>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-menu-background-color
* Menu background color
* --primary-background-color
*
*
* -paper-menu-color
* Menu foreground color
* --primary-text-color
*
*
* --paper-menu-disabled-color
* Foreground color for a disabled item
* --disabled-text-color
*
*
* --paper-menu
* Mixin applied to the menu
* {}
*
*
* --paper-menu-selected-item
* Mixin applied to the selected item
* {}
*
*
* --paper-menu-focused-item
* Mixin applied to the focused item
* {}
*
*
* --paper-menu-focused-item-after
* Mixin applied to the ::after pseudo-element for the focused item
* {}
*
*
*
* Accessibility
* <paper-menu>
has role="menu"
by default. A multi-select menu will also have
aria-multiselectable
set. It implements key bindings to navigate through the menu with the up and
down arrow keys, esc to exit the menu, and enter to activate a menu item. Typing the first letter
of a menu item will also focus it.
*/
@JsType
public interface PaperMenuElement extends HTMLElement {
public static final String TAG = "paper-menu";
public static final String SRC = "paper-menu/paper-menu.html";
/**
* The attribute to use on menu items to look up the item title. Typing the first
letter of an item when the menu is open focuses that item. If unset, textContent
will be used.
*
* JavaScript Info:
* @property attrForItemTitle
* @type String
* @behavior PaperTabs
*/
@JsProperty String getAttrForItemTitle();
/**
* The attribute to use on menu items to look up the item title. Typing the first
letter of an item when the menu is open focuses that item. If unset, textContent
will be used.
*
* JavaScript Info:
* @property attrForItemTitle
* @type String
* @behavior PaperTabs
*/
@JsProperty void setAttrForItemTitle(String value);
/**
* Returns the currently focused item.
*
* JavaScript Info:
* @property focusedItem
* @type Object
* @behavior PaperTabs
*/
@JsProperty JavaScriptObject getFocusedItem();
/**
* Returns the currently focused item.
*
* JavaScript Info:
* @property focusedItem
* @type Object
* @behavior PaperTabs
*/
@JsProperty void setFocusedItem(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTabs
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTabs
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @method select
* @param {} value
* @behavior PaperTabs
*/
void select(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
* @behavior PaperTabs
*/
@JsProperty JavaScriptObject getHostAttributes();
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
* @behavior PaperTabs
*/
@JsProperty void setHostAttributes(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
* @behavior PaperTabs
*/
@JsProperty JavaScriptObject getListeners();
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
* @behavior PaperTabs
*/
@JsProperty void setListeners(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperTabs
*/
@JsProperty JsArray getObservers();
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperTabs
*/
@JsProperty void setObservers(JsArray value);
/**
* If true, multiple selections are allowed.
*
* JavaScript Info:
* @property multi
* @type Boolean
* @behavior PaperTabs
*/
@JsProperty boolean getMulti();
/**
* If true, multiple selections are allowed.
*
* JavaScript Info:
* @property multi
* @type Boolean
* @behavior PaperTabs
*/
@JsProperty void setMulti(boolean value);
/**
*
*
* JavaScript Info:
* @method multiChanged
* @param {} multi
* @behavior PaperTabs
*/
void multiChanged(JavaScriptObject multi);
/**
* Returns an array of currently selected items.
*
* JavaScript Info:
* @property selectedItems
* @type Array
* @behavior PaperTabs
*/
@JsProperty JsArray getSelectedItems();
/**
* Returns an array of currently selected items.
*
* JavaScript Info:
* @property selectedItems
* @type Array
* @behavior PaperTabs
*/
@JsProperty void setSelectedItems(JsArray value);
/**
* Gets or sets the selected elements. This is used instead of selected
when multi
is true.
*
* JavaScript Info:
* @property selectedValues
* @type Array
* @behavior PaperTabs
*/
@JsProperty JsArray getSelectedValues();
/**
* Gets or sets the selected elements. This is used instead of selected
when multi
is true.
*
* JavaScript Info:
* @property selectedValues
* @type Array
* @behavior PaperTabs
*/
@JsProperty void setSelectedValues(JsArray value);
/**
* The event that fires from items when they are selected. Selectable
will listen for this event from items and update the selection state.
Set to empty string to listen to no events.
*
* JavaScript Info:
* @property activateEvent
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty String getActivateEvent();
/**
* The event that fires from items when they are selected. Selectable
will listen for this event from items and update the selection state.
Set to empty string to listen to no events.
*
* JavaScript Info:
* @property activateEvent
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty void setActivateEvent(String value);
/**
* If you want to use the attribute value of an element for selected
instead of the index,
set this to the name of the attribute.
*
* JavaScript Info:
* @property attrForSelected
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty String getAttrForSelected();
/**
* If you want to use the attribute value of an element for selected
instead of the index,
set this to the name of the attribute.
*
* JavaScript Info:
* @property attrForSelected
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty void setAttrForSelected(String value);
/**
*
*
* JavaScript Info:
* @property excludedLocalNames
* @type Object
* @behavior NeonAnimatedPages
*/
@JsProperty JavaScriptObject getExcludedLocalNames();
/**
*
*
* JavaScript Info:
* @property excludedLocalNames
* @type Object
* @behavior NeonAnimatedPages
*/
@JsProperty void setExcludedLocalNames(JavaScriptObject value);
/**
* Returns the index of the given item.
*
* JavaScript Info:
* @method indexOf
* @param {Object} item
* @behavior NeonAnimatedPages
*/
void indexOf(JavaScriptObject item);
/**
* Returns an array of selectable items.
*
* JavaScript Info:
* @method items
* @behavior NeonAnimatedPages
*/
void items();
/**
* Selects the next item.
*
* JavaScript Info:
* @method selectNext
* @behavior NeonAnimatedPages
*/
void selectNext();
/**
* Selects the previous item.
*
* JavaScript Info:
* @method selectPrevious
* @behavior NeonAnimatedPages
*/
void selectPrevious();
/**
* This is a CSS selector sting. If this is set, only items that matches the CSS selector
are selectable.
*
* JavaScript Info:
* @property selectable
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty String getSelectable();
/**
* This is a CSS selector sting. If this is set, only items that matches the CSS selector
are selectable.
*
* JavaScript Info:
* @property selectable
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty void setSelectable(String value);
/**
* Gets or sets the selected element. The default is to use the index of the item.
*
* JavaScript Info:
* @property selected
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty String getSelected();
/**
* Gets or sets the selected element. The default is to use the index of the item.
*
* JavaScript Info:
* @property selected
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty void setSelected(String value);
/**
* The attribute to set on elements when selected.
*
* JavaScript Info:
* @property selectedAttribute
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty String getSelectedAttribute();
/**
* The attribute to set on elements when selected.
*
* JavaScript Info:
* @property selectedAttribute
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty void setSelectedAttribute(String value);
/**
* The class to set on elements when selected.
*
* JavaScript Info:
* @property selectedClass
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty String getSelectedClass();
/**
* The class to set on elements when selected.
*
* JavaScript Info:
* @property selectedClass
* @type string
* @behavior NeonAnimatedPages
*/
@JsProperty void setSelectedClass(String value);
/**
* Returns the currently selected item.
*
* JavaScript Info:
* @property selectedItem
* @type Object
* @behavior NeonAnimatedPages
*/
@JsProperty JavaScriptObject getSelectedItem();
/**
* Returns the currently selected item.
*
* JavaScript Info:
* @property selectedItem
* @type Object
* @behavior NeonAnimatedPages
*/
@JsProperty void setSelectedItem(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @method attached
* @behavior NeonAnimatedPages
*/
void attached();
/**
*
*
* JavaScript Info:
* @method detached
* @behavior NeonAnimatedPages
*/
void detached();
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior PaperTabs
*/
void addOwnKeyBinding(JavaScriptObject eventString, JavaScriptObject handlerName);
/**
* The HTMLElement that will be firing relevant KeyboardEvents.
*
* JavaScript Info:
* @property keyEventTarget
* @type Object
* @behavior PaperTabs
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The HTMLElement that will be firing relevant KeyboardEvents.
*
* JavaScript Info:
* @property keyEventTarget
* @type Object
* @behavior PaperTabs
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {} event
* @param {} eventString
* @behavior PaperTabs
*/
void keyboardEventMatchesKeys(JavaScriptObject event, JavaScriptObject eventString);
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior PaperTabs
*/
void removeOwnKeyBindings();
/**
*
*
* JavaScript Info:
* @method registered
* @behavior PaperTabs
*/
void registered();
}