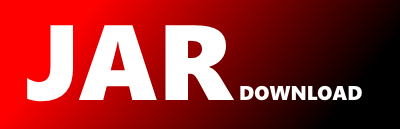
com.vaadin.polymer.paper.widget.PaperDrawerPanel Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-drawer-panel project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.element.*;
import com.vaadin.polymer.paper.widget.event.PaperResponsiveChangeEvent;
import com.vaadin.polymer.paper.widget.event.PaperResponsiveChangeEventHandler;
import com.vaadin.polymer.paper.widget.event.PaperSelectEvent;
import com.vaadin.polymer.paper.widget.event.PaperSelectEventHandler;
import com.vaadin.polymer.PolymerWidget;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* paper-drawer-panel
contains a drawer panel and a main panel. The drawer
and the main panel are side-by-side with drawer on the left. When the browser
window size is smaller than the responsiveWidth
, paper-drawer-panel
changes to narrow layout. In narrow layout, the drawer will be stacked on top
of the main panel. The drawer will slide in/out to hide/reveal the main
panel.
* Use the attribute drawer
to indicate that the element is the drawer panel and
main
to indicate that the element is the main panel.
* Example:
* <paper-drawer-panel>
* <div drawer> Drawer panel... </div>
* <div main> Main panel... </div>
* </paper-drawer-panel>
*
*
*
The drawer and the main panels are not scrollable. You can set CSS overflow
property on the elements to make them scrollable or use paper-header-panel
.
* Example:
* <paper-drawer-panel>
* <paper-header-panel drawer>
* <paper-toolbar></paper-toolbar>
* <div> Drawer content... </div>
* </paper-header-panel>
* <paper-header-panel main>
* <paper-toolbar></paper-toolbar>
* <div> Main content... </div>
* </paper-header-panel>
* </paper-drawer-panel>
*
*
*
An element that should toggle the drawer will automatically do so if it’s
given the paper-drawer-toggle
attribute. Also this element will automatically
be hidden in wide layout.
* Example:
* <paper-drawer-panel>
* <paper-header-panel drawer>
* <paper-toolbar>
* <div>Application</div>
* </paper-toolbar>
* <div> Drawer content... </div>
* </paper-header-panel>
* <paper-header-panel main>
* <paper-toolbar>
* <paper-icon-button icon="menu" paper-drawer-toggle></paper-icon-button>
* <div>Title</div>
* </paper-toolbar>
* <div> Main content... </div>
* </paper-header-panel>
* </paper-drawer-panel>
*
*
*
To position the drawer to the right, add right-drawer
attribute.
* <paper-drawer-panel right-drawer>
* <div drawer> Drawer panel... </div>
* <div main> Main panel... </div>
* </paper-drawer-panel>
*
*
*
Styling paper-drawer-panel:
* To change the main container:
paper-drawer-panel {
—paper-drawer-panel-main-container: {
background-color: gray;
};
}
* To change the drawer container when it’s in the left side:
paper-drawer-panel {
—paper-drawer-panel-left-drawer-container: {
background-color: white;
};
}
* To change the drawer container when it’s in the right side:
* paper-drawer-panel {
—paper-drawer-panel-right-drawer-container: {
background-color: white;
};
}
*/
public class PaperDrawerPanel extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperDrawerPanel() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperDrawerPanel(String html) {
super(PaperDrawerPanelElement.TAG, PaperDrawerPanelElement.SRC, html);
getPolymerElement().addEventListener(
com.vaadin.polymer.paper.element.event.PaperResponsiveChangeEvent.NAME,
new com.vaadin.polymer.paper.element.event.PaperResponsiveChangeEvent.Listener() {
@Override
protected void handleEvent(com.vaadin.polymer.paper.element.event.PaperResponsiveChangeEvent event) {
fireEvent(new PaperResponsiveChangeEvent(event));
}
});
getPolymerElement().addEventListener(
com.vaadin.polymer.paper.element.event.PaperSelectEvent.NAME,
new com.vaadin.polymer.paper.element.event.PaperSelectEvent.Listener() {
@Override
protected void handleEvent(com.vaadin.polymer.paper.element.event.PaperSelectEvent event) {
fireEvent(new PaperSelectEvent(event));
}
});
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperDrawerPanelElement getPolymerElement() {
return (PaperDrawerPanelElement) getElement();
}
/**
* Closes the drawer.
*
* JavaScript Info:
* @method closeDrawer
*
*/
public void closeDrawer() {
getPolymerElement().closeDrawer();
}
/**
* The panel to be selected when paper-drawer-panel
changes to narrow
layout.
*
* JavaScript Info:
* @property defaultSelected
* @type String
*
*/
public String getDefaultSelected(){
return getPolymerElement().getDefaultSelected();
}
/**
* The panel to be selected when paper-drawer-panel
changes to narrow
layout.
*
* JavaScript Info:
* @property defaultSelected
* @type String
*
*/
public void setDefaultSelected(String value) {
getPolymerElement().setDefaultSelected(value);
}
/**
* If true, swipe from the edge is disable.
*
* JavaScript Info:
* @property disableEdgeSwipe
* @type Boolean
*
*/
public boolean getDisableEdgeSwipe(){
return getPolymerElement().getDisableEdgeSwipe();
}
/**
* If true, swipe from the edge is disable.
*
* JavaScript Info:
* @property disableEdgeSwipe
* @type Boolean
*
*/
public void setDisableEdgeSwipe(boolean value) {
getPolymerElement().setDisableEdgeSwipe(value);
}
/**
* If true, swipe to open/close the drawer is disabled.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
public boolean getDisableSwipe(){
return getPolymerElement().getDisableSwipe();
}
/**
* If true, swipe to open/close the drawer is disabled.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
public void setDisableSwipe(boolean value) {
getPolymerElement().setDisableSwipe(value);
}
/**
* Whether the user is dragging the drawer interactively.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
public boolean getDragging(){
return getPolymerElement().getDragging();
}
/**
* Whether the user is dragging the drawer interactively.
*
* JavaScript Info:
* @property dragging
* @type Boolean
*
*/
public void setDragging(boolean value) {
getPolymerElement().setDragging(value);
}
/**
* The attribute on elements that should toggle the drawer on tap, also elements will
automatically be hidden in wide layout.
*
* JavaScript Info:
* @property drawerToggleAttribute
* @type String
*
*/
public String getDrawerToggleAttribute(){
return getPolymerElement().getDrawerToggleAttribute();
}
/**
* The attribute on elements that should toggle the drawer on tap, also elements will
automatically be hidden in wide layout.
*
* JavaScript Info:
* @property drawerToggleAttribute
* @type String
*
*/
public void setDrawerToggleAttribute(String value) {
getPolymerElement().setDrawerToggleAttribute(value);
}
/**
* Width of the drawer panel.
*
* JavaScript Info:
* @property drawerWidth
* @type String
*
*/
public String getDrawerWidth(){
return getPolymerElement().getDrawerWidth();
}
/**
* Width of the drawer panel.
*
* JavaScript Info:
* @property drawerWidth
* @type String
*
*/
public void setDrawerWidth(String value) {
getPolymerElement().setDrawerWidth(value);
}
/**
* How many pixels on the side of the screen are sensitive to edge
swipes and peek.
*
* JavaScript Info:
* @property edgeSwipeSensitivity
* @type Number
*
*/
public double getEdgeSwipeSensitivity(){
return getPolymerElement().getEdgeSwipeSensitivity();
}
/**
* How many pixels on the side of the screen are sensitive to edge
swipes and peek.
*
* JavaScript Info:
* @property edgeSwipeSensitivity
* @type Number
*
*/
public void setEdgeSwipeSensitivity(double value) {
getPolymerElement().setEdgeSwipeSensitivity(value);
}
/**
* How many pixels on the side of the screen are sensitive to edge
swipes and peek.
*
* JavaScript Info:
* @attribute edge-swipe-sensitivity
*
*/
public void setEdgeSwipeSensitivity(String value) {
getPolymerElement().setAttribute("edge-swipe-sensitivity", value);
}
/**
* If true, ignore responsiveWidth
setting and force the narrow layout.
*
* JavaScript Info:
* @property forceNarrow
* @type Boolean
*
*/
public boolean getForceNarrow(){
return getPolymerElement().getForceNarrow();
}
/**
* If true, ignore responsiveWidth
setting and force the narrow layout.
*
* JavaScript Info:
* @property forceNarrow
* @type Boolean
*
*/
public void setForceNarrow(boolean value) {
getPolymerElement().setForceNarrow(value);
}
/**
* Whether the browser has support for the transform CSS property.
*
* JavaScript Info:
* @property hasTransform
* @type Boolean
*
*/
public boolean getHasTransform(){
return getPolymerElement().getHasTransform();
}
/**
* Whether the browser has support for the transform CSS property.
*
* JavaScript Info:
* @property hasTransform
* @type Boolean
*
*/
public void setHasTransform(boolean value) {
getPolymerElement().setHasTransform(value);
}
/**
* Whether the browser has support for the will-change CSS property.
*
* JavaScript Info:
* @property hasWillChange
* @type Boolean
*
*/
public boolean getHasWillChange(){
return getPolymerElement().getHasWillChange();
}
/**
* Whether the browser has support for the will-change CSS property.
*
* JavaScript Info:
* @property hasWillChange
* @type Boolean
*
*/
public void setHasWillChange(boolean value) {
getPolymerElement().setHasWillChange(value);
}
/**
* Returns true if the panel is in narrow layout. This is useful if you
need to show/hide elements based on the layout.
*
* JavaScript Info:
* @property narrow
* @type Boolean
*
*/
public boolean getNarrow(){
return getPolymerElement().getNarrow();
}
/**
* Returns true if the panel is in narrow layout. This is useful if you
need to show/hide elements based on the layout.
*
* JavaScript Info:
* @property narrow
* @type Boolean
*
*/
public void setNarrow(boolean value) {
getPolymerElement().setNarrow(value);
}
/**
* Opens the drawer.
*
* JavaScript Info:
* @method openDrawer
*
*/
public void openDrawer() {
getPolymerElement().openDrawer();
}
/**
* Whether the drawer is peeking out from the edge.
*
* JavaScript Info:
* @property peeking
* @type Boolean
*
*/
public boolean getPeeking(){
return getPolymerElement().getPeeking();
}
/**
* Whether the drawer is peeking out from the edge.
*
* JavaScript Info:
* @property peeking
* @type Boolean
*
*/
public void setPeeking(boolean value) {
getPolymerElement().setPeeking(value);
}
/**
* Max-width when the panel changes to narrow layout.
*
* JavaScript Info:
* @property responsiveWidth
* @type String
*
*/
public String getResponsiveWidth(){
return getPolymerElement().getResponsiveWidth();
}
/**
* Max-width when the panel changes to narrow layout.
*
* JavaScript Info:
* @property responsiveWidth
* @type String
*
*/
public void setResponsiveWidth(String value) {
getPolymerElement().setResponsiveWidth(value);
}
/**
* If true, position the drawer to the right.
*
* JavaScript Info:
* @property rightDrawer
* @type Boolean
*
*/
public boolean getRightDrawer(){
return getPolymerElement().getRightDrawer();
}
/**
* If true, position the drawer to the right.
*
* JavaScript Info:
* @property rightDrawer
* @type Boolean
*
*/
public void setRightDrawer(boolean value) {
getPolymerElement().setRightDrawer(value);
}
/**
* The panel that is being selected. drawer
for the drawer panel and
main
for the main panel.
*
* JavaScript Info:
* @property selected
* @type String
*
*/
public String getSelected(){
return getPolymerElement().getSelected();
}
/**
* The panel that is being selected. drawer
for the drawer panel and
main
for the main panel.
*
* JavaScript Info:
* @property selected
* @type String
*
*/
public void setSelected(String value) {
getPolymerElement().setSelected(value);
}
/**
* Toggles the panel open and closed.
*
* JavaScript Info:
* @method togglePanel
*
*/
public void togglePanel() {
getPolymerElement().togglePanel();
}
/**
* Whether the transition is enabled.
*
* JavaScript Info:
* @property transition
* @type Boolean
*
*/
public boolean getTransition(){
return getPolymerElement().getTransition();
}
/**
* Whether the transition is enabled.
*
* JavaScript Info:
* @property transition
* @type Boolean
*
*/
public void setTransition(boolean value) {
getPolymerElement().setTransition(value);
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
public JavaScriptObject getListeners(){
return getPolymerElement().getListeners();
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
public void setListeners(JavaScriptObject value) {
getPolymerElement().setListeners(value);
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public JsArray getObservers(){
return getPolymerElement().getObservers();
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public void setObservers(JsArray value) {
getPolymerElement().setObservers(value);
}
/**
* Fired when the narrow layout changes.
*
* JavaScript Info:
* @event paper-responsive-change
*/
public HandlerRegistration addPaperResponsiveChangeHandler(PaperResponsiveChangeEventHandler handler) {
return addHandler(handler, PaperResponsiveChangeEvent.TYPE);
}
/**
* Fired when the selected panel changes.
* Listening for this event is an alternative to observing changes in the selected
attribute.
This event is fired both when a panel is selected and deselected.
The isSelected
detail property contains the selection state.
*
* JavaScript Info:
* @event paper-select
*/
public HandlerRegistration addPaperSelectHandler(PaperSelectEventHandler handler) {
return addHandler(handler, PaperSelectEvent.TYPE);
}
}