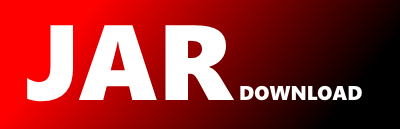
com.vaadin.polymer.paper.widget.PaperInput Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-input project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.widget;
import com.vaadin.polymer.paper.element.*;
import com.vaadin.polymer.PolymerWidget;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* <paper-input>
is a single-line text field with Material Design styling.
* <paper-input label="Input label"></paper-input>
*
*
*
It may include an optional error message or character counter.
* <paper-input error-message="Invalid input!" label="Input label"></paper-input>
* <paper-input char-counter label="Input label"></paper-input>
*
*
*
See Polymer.PaperInputBehavior
for more API docs.
* Styling
* See Polymer.PaperInputContainer
for a list of custom properties used to
style this element.
*/
public class PaperInput extends PolymerWidget {
/**
* Default Constructor.
*/
public PaperInput() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public PaperInput(String html) {
super(PaperInputElement.TAG, PaperInputElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public PaperInputElement getPolymerElement() {
return (PaperInputElement) getElement();
}
/**
* Set this to specify the pattern allowed by preventInvalidInput
. Bind this to the
<input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
* @behavior PaperTextarea
*/
public String getAllowedPattern(){
return getPolymerElement().getAllowedPattern();
}
/**
* Set this to specify the pattern allowed by preventInvalidInput
. Bind this to the
<input is="iron-input">
‘s allowedPattern
property.
*
* JavaScript Info:
* @property allowedPattern
* @type String
* @behavior PaperTextarea
*/
public void setAllowedPattern(String value) {
getPolymerElement().setAllowedPattern(value);
}
/**
* Set to true to always float the label. Bind this to the <paper-input-container>
‘s
alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getAlwaysFloatLabel(){
return getPolymerElement().getAlwaysFloatLabel();
}
/**
* Set to true to always float the label. Bind this to the <paper-input-container>
‘s
alwaysFloatLabel
property.
*
* JavaScript Info:
* @property alwaysFloatLabel
* @type Boolean
* @behavior PaperTextarea
*/
public void setAlwaysFloatLabel(boolean value) {
getPolymerElement().setAlwaysFloatLabel(value);
}
/**
* Set to true to auto-validate the input value. Bind this to the <paper-input-container>
‘s
autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getAutoValidate(){
return getPolymerElement().getAutoValidate();
}
/**
* Set to true to auto-validate the input value. Bind this to the <paper-input-container>
‘s
autoValidate
property.
*
* JavaScript Info:
* @property autoValidate
* @type Boolean
* @behavior PaperTextarea
*/
public void setAutoValidate(boolean value) {
getPolymerElement().setAutoValidate(value);
}
/**
* Bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
* @behavior PaperTextarea
*/
public String getAutocomplete(){
return getPolymerElement().getAutocomplete();
}
/**
* Bind this to the <input is="iron-input">
‘s autocomplete
property.
*
* JavaScript Info:
* @property autocomplete
* @type String
* @behavior PaperTextarea
*/
public void setAutocomplete(String value) {
getPolymerElement().setAutocomplete(value);
}
/**
* Bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getAutofocus(){
return getPolymerElement().getAutofocus();
}
/**
* Bind this to the <input is="iron-input">
‘s autofocus
property.
*
* JavaScript Info:
* @property autofocus
* @type Boolean
* @behavior PaperTextarea
*/
public void setAutofocus(boolean value) {
getPolymerElement().setAutofocus(value);
}
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getCharCounter(){
return getPolymerElement().getCharCounter();
}
/**
* Set to true to show a character counter.
*
* JavaScript Info:
* @property charCounter
* @type Boolean
* @behavior PaperTextarea
*/
public void setCharCounter(boolean value) {
getPolymerElement().setCharCounter(value);
}
/**
* Set to true to disable this input. Bind this to both the <paper-input-container>
‘s
and the input’s disabled
property.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getDisabled(){
return getPolymerElement().getDisabled();
}
/**
* Set to true to disable this input. Bind this to both the <paper-input-container>
‘s
and the input’s disabled
property.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTextarea
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* The error message to display when the input is invalid. Bind this to the
<paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
* @behavior PaperTextarea
*/
public String getErrorMessage(){
return getPolymerElement().getErrorMessage();
}
/**
* The error message to display when the input is invalid. Bind this to the
<paper-input-error>
‘s content, if using.
*
* JavaScript Info:
* @property errorMessage
* @type String
* @behavior PaperTextarea
*/
public void setErrorMessage(String value) {
getPolymerElement().setErrorMessage(value);
}
/**
* Returns a reference to the input element.
*
* JavaScript Info:
* @method inputElement
* @behavior PaperTextarea
*/
public void inputElement() {
getPolymerElement().inputElement();
}
/**
* Bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
* @behavior PaperTextarea
*/
public String getInputmode(){
return getPolymerElement().getInputmode();
}
/**
* Bind this to the <input is="iron-input">
‘s inputmode
property.
*
* JavaScript Info:
* @property inputmode
* @type String
* @behavior PaperTextarea
*/
public void setInputmode(String value) {
getPolymerElement().setInputmode(value);
}
/**
* Returns true if the value is invalid. Bind this to both the <paper-input-container>
‘s
and the input’s invalid
property.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getInvalid(){
return getPolymerElement().getInvalid();
}
/**
* Returns true if the value is invalid. Bind this to both the <paper-input-container>
‘s
and the input’s invalid
property.
*
* JavaScript Info:
* @property invalid
* @type Boolean
* @behavior PaperTextarea
*/
public void setInvalid(boolean value) {
getPolymerElement().setInvalid(value);
}
/**
* The label for this input. Bind this to <paper-input-container>
‘s label
property.
*
* JavaScript Info:
* @property label
* @type String
* @behavior PaperTextarea
*/
public String getLabel(){
return getPolymerElement().getLabel();
}
/**
* The label for this input. Bind this to <paper-input-container>
‘s label
property.
*
* JavaScript Info:
* @property label
* @type String
* @behavior PaperTextarea
*/
public void setLabel(String value) {
getPolymerElement().setLabel(value);
}
/**
* The datalist of the input (if any). This should match the id of an existing
*
* JavaScript Info:
* @property list
* @type String
* @behavior PaperTextarea
*/
public String getList(){
return getPolymerElement().getList();
}
/**
* The datalist of the input (if any). This should match the id of an existing
*
* JavaScript Info:
* @property list
* @type String
* @behavior PaperTextarea
*/
public void setList(String value) {
getPolymerElement().setList(value);
}
/**
* The maximum length of the input value. Bind this to the <input is="iron-input">
‘s
maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
* @behavior PaperTextarea
*/
public double getMaxlength(){
return getPolymerElement().getMaxlength();
}
/**
* The maximum length of the input value. Bind this to the <input is="iron-input">
‘s
maxlength
property.
*
* JavaScript Info:
* @property maxlength
* @type Number
* @behavior PaperTextarea
*/
public void setMaxlength(double value) {
getPolymerElement().setMaxlength(value);
}
/**
* The maximum length of the input value. Bind this to the <input is="iron-input">
‘s
maxlength
property.
*
* JavaScript Info:
* @attribute maxlength
* @behavior PaperTextarea
*/
public void setMaxlength(String value) {
getPolymerElement().setAttribute("maxlength", value);
}
/**
* Bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
* @behavior PaperTextarea
*/
public double getMinlength(){
return getPolymerElement().getMinlength();
}
/**
* Bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @property minlength
* @type Number
* @behavior PaperTextarea
*/
public void setMinlength(double value) {
getPolymerElement().setMinlength(value);
}
/**
* Bind this to the <input is="iron-input">
‘s minlength
property.
*
* JavaScript Info:
* @attribute minlength
* @behavior PaperTextarea
*/
public void setMinlength(String value) {
getPolymerElement().setAttribute("minlength", value);
}
/**
* Bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
* @behavior PaperTextarea
*/
public String getName(){
return getPolymerElement().getName();
}
/**
* Bind this to the <input is="iron-input">
‘s name
property.
*
* JavaScript Info:
* @property name
* @type String
* @behavior PaperTextarea
*/
public void setName(String value) {
getPolymerElement().setName(value);
}
/**
* Set to true to disable the floating label. Bind this to the <paper-input-container>
‘s
noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getNoLabelFloat(){
return getPolymerElement().getNoLabelFloat();
}
/**
* Set to true to disable the floating label. Bind this to the <paper-input-container>
‘s
noLabelFloat
property.
*
* JavaScript Info:
* @property noLabelFloat
* @type Boolean
* @behavior PaperTextarea
*/
public void setNoLabelFloat(boolean value) {
getPolymerElement().setNoLabelFloat(value);
}
/**
* A pattern to validate the input
with. Bind this to the <input is="iron-input">
‘s
pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
* @behavior PaperTextarea
*/
public String getPattern(){
return getPolymerElement().getPattern();
}
/**
* A pattern to validate the input
with. Bind this to the <input is="iron-input">
‘s
pattern
property.
*
* JavaScript Info:
* @property pattern
* @type String
* @behavior PaperTextarea
*/
public void setPattern(String value) {
getPolymerElement().setPattern(value);
}
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
* @behavior PaperTextarea
*/
public String getPlaceholder(){
return getPolymerElement().getPlaceholder();
}
/**
* A placeholder string in addition to the label. If this is set, the label will always float.
*
* JavaScript Info:
* @property placeholder
* @type String
* @behavior PaperTextarea
*/
public void setPlaceholder(String value) {
getPolymerElement().setPlaceholder(value);
}
/**
* Set to true to prevent the user from entering invalid input. Bind this to the
<input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getPreventInvalidInput(){
return getPolymerElement().getPreventInvalidInput();
}
/**
* Set to true to prevent the user from entering invalid input. Bind this to the
<input is="iron-input">
‘s preventInvalidInput
property.
*
* JavaScript Info:
* @property preventInvalidInput
* @type Boolean
* @behavior PaperTextarea
*/
public void setPreventInvalidInput(boolean value) {
getPolymerElement().setPreventInvalidInput(value);
}
/**
* Bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getReadonly(){
return getPolymerElement().getReadonly();
}
/**
* Bind this to the <input is="iron-input">
‘s readonly
property.
*
* JavaScript Info:
* @property readonly
* @type Boolean
* @behavior PaperTextarea
*/
public void setReadonly(boolean value) {
getPolymerElement().setReadonly(value);
}
/**
* Set to true to mark the input as required. Bind this to the <input is="iron-input">
‘s
required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior PaperTextarea
*/
public boolean getRequired(){
return getPolymerElement().getRequired();
}
/**
* Set to true to mark the input as required. Bind this to the <input is="iron-input">
‘s
required
property.
*
* JavaScript Info:
* @property required
* @type Boolean
* @behavior PaperTextarea
*/
public void setRequired(boolean value) {
getPolymerElement().setRequired(value);
}
/**
* Bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
* @behavior PaperTextarea
*/
public double getSize(){
return getPolymerElement().getSize();
}
/**
* Bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @property size
* @type Number
* @behavior PaperTextarea
*/
public void setSize(double value) {
getPolymerElement().setSize(value);
}
/**
* Bind this to the <input is="iron-input">
‘s size
property.
*
* JavaScript Info:
* @attribute size
* @behavior PaperTextarea
*/
public void setSize(String value) {
getPolymerElement().setAttribute("size", value);
}
/**
* The type of the input. The supported types are text
, number
and password
. Bind this
to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
* @behavior PaperTextarea
*/
public String getType(){
return getPolymerElement().getType();
}
/**
* The type of the input. The supported types are text
, number
and password
. Bind this
to the <input is="iron-input">
‘s type
property.
*
* JavaScript Info:
* @property type
* @type String
* @behavior PaperTextarea
*/
public void setType(String value) {
getPolymerElement().setType(value);
}
/**
* Restores the cursor to its original position after updating the value.
*
* JavaScript Info:
* @method updateValueAndPreserveCaret
* @param {string} newValue
* @behavior PaperTextarea
*/
public void updateValueAndPreserveCaret(String newValue) {
getPolymerElement().updateValueAndPreserveCaret(newValue);
}
/**
* Validates the input element and sets an error style if needed.
*
* JavaScript Info:
* @method validate
* @behavior PaperTextarea
*/
public void validate() {
getPolymerElement().validate();
}
/**
* Name of the validator to use. Bind this to the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior PaperTextarea
*/
public String getValidator(){
return getPolymerElement().getValidator();
}
/**
* Name of the validator to use. Bind this to the <input is="iron-input">
‘s validator
property.
*
* JavaScript Info:
* @property validator
* @type String
* @behavior PaperTextarea
*/
public void setValidator(String value) {
getPolymerElement().setValidator(value);
}
/**
* The value for this input. Bind this to the <input is="iron-input">
‘s bindValue
property, or the value property of your input that is notify:true
.
*
* JavaScript Info:
* @property value
* @type String
* @behavior PaperTextarea
*/
public String getValue(){
return getPolymerElement().getValue();
}
/**
* The value for this input. Bind this to the <input is="iron-input">
‘s bindValue
property, or the value property of your input that is notify:true
.
*
* JavaScript Info:
* @property value
* @type String
* @behavior PaperTextarea
*/
public void setValue(String value) {
getPolymerElement().setValue(value);
}
/**
*
*
* JavaScript Info:
* @method attached
* @behavior PaperTextarea
*/
public void attached() {
getPolymerElement().attached();
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
* @behavior PaperTextarea
*/
public JavaScriptObject getListeners(){
return getPolymerElement().getListeners();
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
* @behavior PaperTextarea
*/
public void setListeners(JavaScriptObject value) {
getPolymerElement().setListeners(value);
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperInput
*/
public boolean getFocused(){
return getPolymerElement().getFocused();
}
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperInput
*/
public void setFocused(boolean value) {
getPolymerElement().setFocused(value);
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperInput
*/
public JsArray getObservers(){
return getPolymerElement().getObservers();
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperInput
*/
public void setObservers(JsArray value) {
getPolymerElement().setObservers(value);
}
}