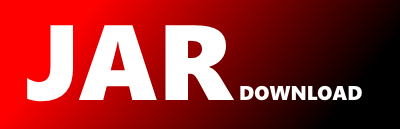
com.vaadin.polymer.iron.widget.IronAjax Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-ajax project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.element.*;
import com.vaadin.polymer.error.widget.event.ErrorEvent;
import com.vaadin.polymer.error.widget.event.ErrorEventHandler;
import com.vaadin.polymer.request.widget.event.RequestEvent;
import com.vaadin.polymer.request.widget.event.RequestEventHandler;
import com.vaadin.polymer.response.widget.event.ResponseEvent;
import com.vaadin.polymer.response.widget.event.ResponseEventHandler;
import com.vaadin.polymer.PolymerWidget;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* The iron-ajax
element exposes network request functionality.
* <iron-ajax
* auto
* url="http://gdata.youtube.com/feeds/api/videos/"
* params='{"alt":"json", "q":"chrome"}'
* handle-as="json"
* on-response="handleResponse"
* debounce-duration="300"></iron-ajax>
*
*
*
With auto
set to true
, the element performs a request whenever
its url
, params
or body
properties are changed. Automatically generated
requests will be debounced in the case that multiple attributes are changed
sequentially.
* Note: The params
attribute must be double quoted JSON.
* You can trigger a request explicitly by calling generateRequest
on the
element.
*/
public class IronAjax extends PolymerWidget {
/**
* Default Constructor.
*/
public IronAjax() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronAjax(String html) {
super(IronAjaxElement.TAG, IronAjaxElement.SRC, html);
getPolymerElement().addEventListener(
com.vaadin.polymer.error.element.event.ErrorEvent.NAME,
new com.vaadin.polymer.error.element.event.ErrorEvent.Listener() {
@Override
protected void handleEvent(com.vaadin.polymer.error.element.event.ErrorEvent event) {
fireEvent(new ErrorEvent(event));
}
});
getPolymerElement().addEventListener(
com.vaadin.polymer.request.element.event.RequestEvent.NAME,
new com.vaadin.polymer.request.element.event.RequestEvent.Listener() {
@Override
protected void handleEvent(com.vaadin.polymer.request.element.event.RequestEvent event) {
fireEvent(new RequestEvent(event));
}
});
getPolymerElement().addEventListener(
com.vaadin.polymer.response.element.event.ResponseEvent.NAME,
new com.vaadin.polymer.response.element.event.ResponseEvent.Listener() {
@Override
protected void handleEvent(com.vaadin.polymer.response.element.event.ResponseEvent event) {
fireEvent(new ResponseEvent(event));
}
});
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronAjaxElement getPolymerElement() {
try {
return (IronAjaxElement) getElement();
} catch (ClassCastException e) {
jsinteropError();
return null;
}
}
/**
* An Array of all in-flight requests originating from this iron-ajax
element.
*
* JavaScript Info:
* @property activeRequests
* @type Array
*
*/
public JsArray getActiveRequests(){
return getPolymerElement().getActiveRequests();
}
/**
* An Array of all in-flight requests originating from this iron-ajax
element.
*
* JavaScript Info:
* @property activeRequests
* @type Array
*
*/
public void setActiveRequests(JsArray value) {
getPolymerElement().setActiveRequests(value);
}
/**
* An Array of all in-flight requests originating from this iron-ajax
element.
*
* JavaScript Info:
* @attribute active-requests
*
*/
public void setActiveRequests(String value) {
getPolymerElement().setAttribute("active-requests", value);
}
/**
* If true, automatically performs an Ajax request when either url
or
params
changes.
*
* JavaScript Info:
* @property auto
* @type Boolean
*
*/
public boolean getAuto(){
return getPolymerElement().getAuto();
}
/**
* If true, automatically performs an Ajax request when either url
or
params
changes.
*
* JavaScript Info:
* @property auto
* @type Boolean
*
*/
public void setAuto(boolean value) {
getPolymerElement().setAuto(value);
}
/**
* Body content to send with the request, typically used with “POST”
requests.
* If body is a string it will be sent unmodified.
* If Content-Type is set to a value listed below, then
the body will be encoded accordingly.
*
* content-type="application/json"
* - body is encoded like
{"foo":"bar baz","x":1}
*
*
* content-type="application/x-www-form-urlencoded"
* - body is encoded like
foo=bar+baz&x=1
*
*
*
* Otherwise the body will be passed to the browser unmodified, and it
will handle any encoding (e.g. for FormData, Blob, ArrayBuffer).
*
* JavaScript Info:
* @property body
* @type Object
*
*/
public JavaScriptObject getBody(){
return getPolymerElement().getBody();
}
/**
* Body content to send with the request, typically used with “POST”
requests.
* If body is a string it will be sent unmodified.
* If Content-Type is set to a value listed below, then
the body will be encoded accordingly.
*
* content-type="application/json"
* - body is encoded like
{"foo":"bar baz","x":1}
*
*
* content-type="application/x-www-form-urlencoded"
* - body is encoded like
foo=bar+baz&x=1
*
*
*
* Otherwise the body will be passed to the browser unmodified, and it
will handle any encoding (e.g. for FormData, Blob, ArrayBuffer).
*
* JavaScript Info:
* @property body
* @type Object
*
*/
public void setBody(JavaScriptObject value) {
getPolymerElement().setBody(value);
}
/**
* Body content to send with the request, typically used with “POST”
requests.
* If body is a string it will be sent unmodified.
* If Content-Type is set to a value listed below, then
the body will be encoded accordingly.
*
* content-type="application/json"
* - body is encoded like
{"foo":"bar baz","x":1}
*
*
* content-type="application/x-www-form-urlencoded"
* - body is encoded like
foo=bar+baz&x=1
*
*
*
* Otherwise the body will be passed to the browser unmodified, and it
will handle any encoding (e.g. for FormData, Blob, ArrayBuffer).
*
* JavaScript Info:
* @attribute body
*
*/
public void setBody(String value) {
getPolymerElement().setAttribute("body", value);
}
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
public String getContentType(){
return getPolymerElement().getContentType();
}
/**
* Content type to use when sending data. If the contentType
property
is set and a Content-Type
header is specified in the headers
property, the headers
property value will take precedence.
*
* JavaScript Info:
* @property contentType
* @type String
*
*/
public void setContentType(String value) {
getPolymerElement().setContentType(value);
}
/**
* Length of time in milliseconds to debounce multiple requests.
*
* JavaScript Info:
* @property debounceDuration
* @type Number
*
*/
public double getDebounceDuration(){
return getPolymerElement().getDebounceDuration();
}
/**
* Length of time in milliseconds to debounce multiple requests.
*
* JavaScript Info:
* @property debounceDuration
* @type Number
*
*/
public void setDebounceDuration(double value) {
getPolymerElement().setDebounceDuration(value);
}
/**
* Length of time in milliseconds to debounce multiple requests.
*
* JavaScript Info:
* @attribute debounce-duration
*
*/
public void setDebounceDuration(String value) {
getPolymerElement().setAttribute("debounce-duration", value);
}
/**
* Performs an AJAX request to the specified URL.
*
* JavaScript Info:
* @method generateRequest
*
*/
public void generateRequest() {
getPolymerElement().generateRequest();
}
/**
* Specifies what data to store in the response
property, and
to deliver as event.detail.response
in response
events.
* One of:
* text
: uses XHR.responseText
.
* xml
: uses XHR.responseXML
.
* json
: uses XHR.responseText
parsed as JSON.
* arraybuffer
: uses XHR.response
.
* blob
: uses XHR.response
.
* document
: uses XHR.response
.
*
* JavaScript Info:
* @property handleAs
* @type String
*
*/
public String getHandleAs(){
return getPolymerElement().getHandleAs();
}
/**
* Specifies what data to store in the response
property, and
to deliver as event.detail.response
in response
events.
* One of:
* text
: uses XHR.responseText
.
* xml
: uses XHR.responseXML
.
* json
: uses XHR.responseText
parsed as JSON.
* arraybuffer
: uses XHR.response
.
* blob
: uses XHR.response
.
* document
: uses XHR.response
.
*
* JavaScript Info:
* @property handleAs
* @type String
*
*/
public void setHandleAs(String value) {
getPolymerElement().setHandleAs(value);
}
/**
* HTTP request headers to send.
* Example:
* <iron-ajax
* auto
* url="http://somesite.com"
* headers='{"X-Requested-With": "XMLHttpRequest"}'
* handle-as="json"></iron-ajax>
*
*
*
Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
public JavaScriptObject getHeaders(){
return getPolymerElement().getHeaders();
}
/**
* HTTP request headers to send.
* Example:
* <iron-ajax
* auto
* url="http://somesite.com"
* headers='{"X-Requested-With": "XMLHttpRequest"}'
* handle-as="json"></iron-ajax>
*
*
*
Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
public void setHeaders(JavaScriptObject value) {
getPolymerElement().setHeaders(value);
}
/**
* HTTP request headers to send.
* Example:
* <iron-ajax
* auto
* url="http://somesite.com"
* headers='{"X-Requested-With": "XMLHttpRequest"}'
* handle-as="json"></iron-ajax>
*
*
*
Note: setting a Content-Type
header here will override the value
specified by the contentType
property of this element.
*
* JavaScript Info:
* @attribute headers
*
*/
public void setHeaders(String value) {
getPolymerElement().setAttribute("headers", value);
}
/**
* Will be set to the most recent error that resulted from a request
that originated from this iron-ajax element.
*
* JavaScript Info:
* @property lastError
* @type Object
*
*/
public JavaScriptObject getLastError(){
return getPolymerElement().getLastError();
}
/**
* Will be set to the most recent error that resulted from a request
that originated from this iron-ajax element.
*
* JavaScript Info:
* @property lastError
* @type Object
*
*/
public void setLastError(JavaScriptObject value) {
getPolymerElement().setLastError(value);
}
/**
* Will be set to the most recent error that resulted from a request
that originated from this iron-ajax element.
*
* JavaScript Info:
* @attribute last-error
*
*/
public void setLastError(String value) {
getPolymerElement().setAttribute("last-error", value);
}
/**
* Will be set to the most recent request made by this iron-ajax element.
*
* JavaScript Info:
* @property lastRequest
* @type Object
*
*/
public JavaScriptObject getLastRequest(){
return getPolymerElement().getLastRequest();
}
/**
* Will be set to the most recent request made by this iron-ajax element.
*
* JavaScript Info:
* @property lastRequest
* @type Object
*
*/
public void setLastRequest(JavaScriptObject value) {
getPolymerElement().setLastRequest(value);
}
/**
* Will be set to the most recent request made by this iron-ajax element.
*
* JavaScript Info:
* @attribute last-request
*
*/
public void setLastRequest(String value) {
getPolymerElement().setAttribute("last-request", value);
}
/**
* Will be set to the most recent response received by a request
that originated from this iron-ajax element. The type of the response
is determined by the value of handleAs
at the time that the request
was generated.
*
* JavaScript Info:
* @property lastResponse
* @type Object
*
*/
public JavaScriptObject getLastResponse(){
return getPolymerElement().getLastResponse();
}
/**
* Will be set to the most recent response received by a request
that originated from this iron-ajax element. The type of the response
is determined by the value of handleAs
at the time that the request
was generated.
*
* JavaScript Info:
* @property lastResponse
* @type Object
*
*/
public void setLastResponse(JavaScriptObject value) {
getPolymerElement().setLastResponse(value);
}
/**
* Will be set to the most recent response received by a request
that originated from this iron-ajax element. The type of the response
is determined by the value of handleAs
at the time that the request
was generated.
*
* JavaScript Info:
* @attribute last-response
*
*/
public void setLastResponse(String value) {
getPolymerElement().setAttribute("last-response", value);
}
/**
* Will be set to true if there is at least one in-flight request
associated with this iron-ajax element.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
public boolean getLoading(){
return getPolymerElement().getLoading();
}
/**
* Will be set to true if there is at least one in-flight request
associated with this iron-ajax element.
*
* JavaScript Info:
* @property loading
* @type Boolean
*
*/
public void setLoading(boolean value) {
getPolymerElement().setLoading(value);
}
/**
* The HTTP method to use such as ‘GET’, ‘POST’, ‘PUT’, or ‘DELETE’.
Default is ‘GET’.
*
* JavaScript Info:
* @property method
* @type String
*
*/
public String getMethod(){
return getPolymerElement().getMethod();
}
/**
* The HTTP method to use such as ‘GET’, ‘POST’, ‘PUT’, or ‘DELETE’.
Default is ‘GET’.
*
* JavaScript Info:
* @property method
* @type String
*
*/
public void setMethod(String value) {
getPolymerElement().setMethod(value);
}
/**
* An object that contains query parameters to be appended to the
specified url
when generating a request. If you wish to set the body
content when making a POST request, you should use the body
property
instead.
*
* JavaScript Info:
* @property params
* @type Object
*
*/
public JavaScriptObject getParams(){
return getPolymerElement().getParams();
}
/**
* An object that contains query parameters to be appended to the
specified url
when generating a request. If you wish to set the body
content when making a POST request, you should use the body
property
instead.
*
* JavaScript Info:
* @property params
* @type Object
*
*/
public void setParams(JavaScriptObject value) {
getPolymerElement().setParams(value);
}
/**
* An object that contains query parameters to be appended to the
specified url
when generating a request. If you wish to set the body
content when making a POST request, you should use the body
property
instead.
*
* JavaScript Info:
* @attribute params
*
*/
public void setParams(String value) {
getPolymerElement().setAttribute("params", value);
}
/**
* The query string that should be appended to the url
, serialized from
the current value of params
.
*
* JavaScript Info:
* @method queryString
*
*/
public void queryString() {
getPolymerElement().queryString();
}
/**
* An object that maps header names to header values, first applying the
the value of Content-Type
and then overlaying the headers specified
in the headers
property.
*
* JavaScript Info:
* @method requestHeaders
*
*/
public void requestHeaders() {
getPolymerElement().requestHeaders();
}
/**
* The url
with query string (if params
are specified), suitable for
providing to an iron-request
instance.
*
* JavaScript Info:
* @method requestUrl
*
*/
public void requestUrl() {
getPolymerElement().requestUrl();
}
/**
* Toggle whether XHR is synchronous or asynchronous. Don’t change this
to true unless You Know What You Are Doing™.
*
* JavaScript Info:
* @property sync
* @type Boolean
*
*/
public boolean getSync(){
return getPolymerElement().getSync();
}
/**
* Toggle whether XHR is synchronous or asynchronous. Don’t change this
to true unless You Know What You Are Doing™.
*
* JavaScript Info:
* @property sync
* @type Boolean
*
*/
public void setSync(boolean value) {
getPolymerElement().setSync(value);
}
/**
* Request options suitable for generating an iron-request
instance based
on the current state of the iron-ajax
instance’s properties.
*
* JavaScript Info:
* @method toRequestOptions
*
*/
public void toRequestOptions() {
getPolymerElement().toRequestOptions();
}
/**
* The URL target of the request.
*
* JavaScript Info:
* @property url
* @type String
*
*/
public String getUrl(){
return getPolymerElement().getUrl();
}
/**
* The URL target of the request.
*
* JavaScript Info:
* @property url
* @type String
*
*/
public void setUrl(String value) {
getPolymerElement().setUrl(value);
}
/**
* If true, error messages will automatically be logged to the console.
*
* JavaScript Info:
* @property verbose
* @type Boolean
*
*/
public boolean getVerbose(){
return getPolymerElement().getVerbose();
}
/**
* If true, error messages will automatically be logged to the console.
*
* JavaScript Info:
* @property verbose
* @type Boolean
*
*/
public void setVerbose(boolean value) {
getPolymerElement().setVerbose(value);
}
/**
* Set the withCredentials flag on the request.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
public boolean getWithCredentials(){
return getPolymerElement().getWithCredentials();
}
/**
* Set the withCredentials flag on the request.
*
* JavaScript Info:
* @property withCredentials
* @type Boolean
*
*/
public void setWithCredentials(boolean value) {
getPolymerElement().setWithCredentials(value);
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public JsArray getObservers(){
return getPolymerElement().getObservers();
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public void setObservers(JsArray value) {
getPolymerElement().setObservers(value);
}
/**
* Fired when an error is received.
*
* JavaScript Info:
* @event error
*/
public HandlerRegistration addErrorHandler(ErrorEventHandler handler) {
return addHandler(handler, ErrorEvent.TYPE);
}
/**
* Fired when a request is sent.
*
* JavaScript Info:
* @event request
*/
public HandlerRegistration addRequestHandler(RequestEventHandler handler) {
return addHandler(handler, RequestEvent.TYPE);
}
/**
* Fired when a response is received.
*
* JavaScript Info:
* @event response
*/
public HandlerRegistration addResponseHandler(ResponseEventHandler handler) {
return addHandler(handler, ResponseEvent.TYPE);
}
}