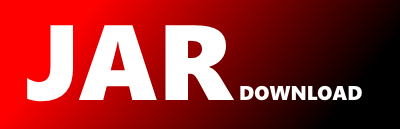
com.vaadin.polymer.iron.widget.IronList Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from iron-list project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.iron.widget;
import com.vaadin.polymer.iron.element.*;
import com.vaadin.polymer.PolymerWidget;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* iron-list
displays a virtual, ‘infinite’ list. The template inside
the iron-list element represents the DOM to create for each list item.
The items
property specifies an array of list item data.
* For performance reasons, not every item in the list is rendered at once;
instead a small subset of actual template elements (enough to fill the viewport)
are rendered and reused as the user scrolls. As such, it is important that all
state of the list template be bound to the model driving it, since the view may
be reused with a new model at any time. Particularly, any state that may change
as the result of a user interaction with the list item must be bound to the model
to avoid view state inconsistency.
* Important: iron-list
must ether be explicitly sized, or delegate scrolling to an
explicitly sized parent. By “explicitly sized”, we mean it either has an explicit
CSS height
property set via a class or inline style, or else is sized by other
layout means (e.g. the flex
or fit
classes).
* Template model
* List item templates should bind to template models of the following structure:
* {
* index: 0, // data index for this item
* item: { // user data corresponding to items[index]
* /* user item data * /
* }
* }
*
*
*
Alternatively, you can change the property name used as data index by changing the
indexAs
property. The as
property defines the name of the variable to add to the binding
scope for the array.
* For example, given the following data
array:
* data.json
* [
* {"name": "Bob"},
* {"name": "Tim"},
* {"name": "Mike"}
* ]
*
*
*
The following code would render the list (note the name and checked properties are
bound from the model object provided to the template scope):
* <template is="dom-bind">
* <iron-ajax url="data.json" last-response="{{data}}" auto></iron-ajax>
* <iron-list items="[[data]]" as="item">
* <template>
* <div>
* Name: <span>[[item.name]]</span>
* </div>
* </template>
* </iron-list>
* </template>
*
*
*
Resizing
* iron-list
lays out the items when it recives a notification via the resize
event.
This event is fired by any element that implements IronResizableBehavior
.
* By default, elements such as iron-pages
, paper-tabs
or paper-dialog
will trigger
this event automatically. If you hide the list manually (e.g. you use display: none
)
you might want to implement IronResizableBehavior
or fire this event manually right
after the list became visible again. e.g.
* document.querySelector('iron-list').fire('resize');
*
*
*
*
*/
public class IronList extends PolymerWidget {
/**
* Default Constructor.
*/
public IronList() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public IronList(String html) {
super(IronListElement.TAG, IronListElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public IronListElement getPolymerElement() {
try {
return (IronListElement) getElement();
} catch (ClassCastException e) {
jsinteropError();
return null;
}
}
/**
* The name of the variable to add to the binding scope for the array
element associated with a given template instance.
*
* JavaScript Info:
* @property as
* @type String
*
*/
public String getAs(){
return getPolymerElement().getAs();
}
/**
* The name of the variable to add to the binding scope for the array
element associated with a given template instance.
*
* JavaScript Info:
* @property as
* @type String
*
*/
public void setAs(String value) {
getPolymerElement().setAs(value);
}
/**
* Clears the current selection state of the list.
*
* JavaScript Info:
* @method clearSelection
*
*/
public void clearSelection() {
getPolymerElement().clearSelection();
}
/**
* Deselects the given item list if it is already selected.
*
* JavaScript Info:
* @method deselectItem
* @param {(Object|number)} item
*
*/
public void deselectItem(JavaScriptObject item) {
getPolymerElement().deselectItem(item);
}
/**
* Gets the first visible item in the viewport.
*
* JavaScript Info:
* @method firstVisibleIndex
*
*/
public void firstVisibleIndex() {
getPolymerElement().firstVisibleIndex();
}
/**
* The name of the variable to add to the binding scope with the index
for the row. If sort
is provided, the index will reflect the
sorted order (rather than the original array order).
*
* JavaScript Info:
* @property indexAs
* @type String
*
*/
public String getIndexAs(){
return getPolymerElement().getIndexAs();
}
/**
* The name of the variable to add to the binding scope with the index
for the row. If sort
is provided, the index will reflect the
sorted order (rather than the original array order).
*
* JavaScript Info:
* @property indexAs
* @type String
*
*/
public void setIndexAs(String value) {
getPolymerElement().setIndexAs(value);
}
/**
* An array containing items determining how many instances of the template
to stamp and that that each template instance should bind to.
*
* JavaScript Info:
* @property items
* @type Array
*
*/
public JsArray getItems(){
return getPolymerElement().getItems();
}
/**
* An array containing items determining how many instances of the template
to stamp and that that each template instance should bind to.
*
* JavaScript Info:
* @property items
* @type Array
*
*/
public void setItems(JsArray value) {
getPolymerElement().setItems(value);
}
/**
* An array containing items determining how many instances of the template
to stamp and that that each template instance should bind to.
*
* JavaScript Info:
* @attribute items
*
*/
public void setItems(String value) {
getPolymerElement().setAttribute("items", value);
}
/**
* When true
, multiple items may be selected at once (in this case,
selected
is an array of currently selected items). When false
,
only one item may be selected at a time.
*
* JavaScript Info:
* @property multiSelection
* @type Boolean
*
*/
public boolean getMultiSelection(){
return getPolymerElement().getMultiSelection();
}
/**
* When true
, multiple items may be selected at once (in this case,
selected
is an array of currently selected items). When false
,
only one item may be selected at a time.
*
* JavaScript Info:
* @property multiSelection
* @type Boolean
*
*/
public void setMultiSelection(boolean value) {
getPolymerElement().setMultiSelection(value);
}
/**
* Scroll to a specific item in the virtual list regardless
of the physical items in the DOM tree.
*
* JavaScript Info:
* @method scrollToIndex
* @param {number} idx
*
*/
public void scrollToIndex(double idx) {
getPolymerElement().scrollToIndex(idx);
}
/**
* Select the list item at the given index.
*
* JavaScript Info:
* @method selectItem
* @param {(Object|number)} item
*
*/
public void selectItem(JavaScriptObject item) {
getPolymerElement().selectItem(item);
}
/**
* The name of the variable to add to the binding scope to indicate
if the row is selected.
*
* JavaScript Info:
* @property selectedAs
* @type String
*
*/
public String getSelectedAs(){
return getPolymerElement().getSelectedAs();
}
/**
* The name of the variable to add to the binding scope to indicate
if the row is selected.
*
* JavaScript Info:
* @property selectedAs
* @type String
*
*/
public void setSelectedAs(String value) {
getPolymerElement().setSelectedAs(value);
}
/**
* When multiSelection
is false, this is the currently selected item, or null
if no item is selected.
*
* JavaScript Info:
* @property selectedItem
* @type Object
*
*/
public JavaScriptObject getSelectedItem(){
return getPolymerElement().getSelectedItem();
}
/**
* When multiSelection
is false, this is the currently selected item, or null
if no item is selected.
*
* JavaScript Info:
* @property selectedItem
* @type Object
*
*/
public void setSelectedItem(JavaScriptObject value) {
getPolymerElement().setSelectedItem(value);
}
/**
* When multiSelection
is false, this is the currently selected item, or null
if no item is selected.
*
* JavaScript Info:
* @attribute selected-item
*
*/
public void setSelectedItem(String value) {
getPolymerElement().setAttribute("selected-item", value);
}
/**
* When multiSelection
is true, this is an array that contains the selected items.
*
* JavaScript Info:
* @property selectedItems
* @type Object
*
*/
public JavaScriptObject getSelectedItems(){
return getPolymerElement().getSelectedItems();
}
/**
* When multiSelection
is true, this is an array that contains the selected items.
*
* JavaScript Info:
* @property selectedItems
* @type Object
*
*/
public void setSelectedItems(JavaScriptObject value) {
getPolymerElement().setSelectedItems(value);
}
/**
* When multiSelection
is true, this is an array that contains the selected items.
*
* JavaScript Info:
* @attribute selected-items
*
*/
public void setSelectedItems(String value) {
getPolymerElement().setAttribute("selected-items", value);
}
/**
* When true, tapping a row will select the item, placing its data model
in the set of selected items retrievable via the selection property.
* Note that tapping focusable elements within the list item will not
result in selection, since they are presumed to have their * own action.
*
* JavaScript Info:
* @property selectionEnabled
* @type Boolean
*
*/
public boolean getSelectionEnabled(){
return getPolymerElement().getSelectionEnabled();
}
/**
* When true, tapping a row will select the item, placing its data model
in the set of selected items retrievable via the selection property.
* Note that tapping focusable elements within the list item will not
result in selection, since they are presumed to have their * own action.
*
* JavaScript Info:
* @property selectionEnabled
* @type Boolean
*
*/
public void setSelectionEnabled(boolean value) {
getPolymerElement().setSelectionEnabled(value);
}
/**
* Select or deselect a given item depending on whether the item
has already been selected.
*
* JavaScript Info:
* @method toggleSelectionForItem
* @param {(Object|number)} item
*
*/
public void toggleSelectionForItem(JavaScriptObject item) {
getPolymerElement().toggleSelectionForItem(item);
}
/**
* Invoke this method if you dynamically update the viewport’s
size or CSS padding.
*
* JavaScript Info:
* @method updateViewportBoundaries
*
*/
public void updateViewportBoundaries() {
getPolymerElement().updateViewportBoundaries();
}
/**
* When the element has been attached to the DOM tree.
*
* JavaScript Info:
* @method attached
*
*/
public void attached() {
getPolymerElement().attached();
}
/**
* When the element has been removed from the DOM tree.
*
* JavaScript Info:
* @method detached
*
*/
public void detached() {
getPolymerElement().detached();
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
public JavaScriptObject getListeners(){
return getPolymerElement().getListeners();
}
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
public void setListeners(JavaScriptObject value) {
getPolymerElement().setListeners(value);
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public JsArray getObservers(){
return getPolymerElement().getObservers();
}
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
*
*/
public void setObservers(JsArray value) {
getPolymerElement().setObservers(value);
}
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior PaperTabs
*/
public void assignParentResizable(JavaScriptObject parentResizable) {
getPolymerElement().assignParentResizable(parentResizable);
}
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior PaperTabs
*/
public void notifyResize() {
getPolymerElement().notifyResize();
}
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior PaperTabs
*/
public void resizerShouldNotify(JavaScriptObject element) {
getPolymerElement().resizerShouldNotify(element);
}
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior PaperTabs
*/
public void stopResizeNotificationsFor(JavaScriptObject target) {
getPolymerElement().stopResizeNotificationsFor(target);
}
}