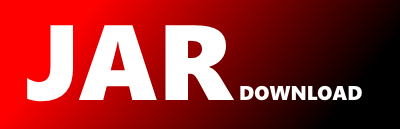
com.vaadin.polymer.paper.element.PaperMenuButtonElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-menu-button project by The Polymer Authors
* that is licensed with MIT license.
*/
package com.vaadin.polymer.paper.element;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.core.client.js.JsProperty;
import com.google.gwt.core.client.js.JsType;
/**
* paper-menu-button
allows one to compose a designated “trigger” element with
another element that represents “content”, to create a dropdown menu that
displays the “content” when the “trigger” is clicked.
* The child element with the class dropdown-trigger
will be used as the
“trigger” element. The child element with the class dropdown-content
will be
used as the “content” element.
* The paper-menu-button
is sensitive to its content’s iron-select
events. If
the “content” element triggers an iron-select
event, the paper-menu-button
will close automatically.
* Example:
* <paper-menu-button>
* <paper-icon-button icon="menu" class="dropdown-trigger"></paper-icon-button>
* <paper-menu class="dropdown-content">
* <paper-item>Share</paper-item>
* <paper-item>Settings</paper-item>
* <paper-item>Help</paper-item>
* </paper-menu>
* </paper-menu-button>
*
*
*
Styling
* The following custom properties and mixins are also available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-menu-button-dropdown-background
* Background color of the paper-menu-button dropdown
* #fff
*
*
* --paper-menu-button
* Mixin applied to the paper-menu-button
* {}
*
*
* --paper-menu-button-disabled
* Mixin applied to the paper-menu-button when disabled
* {}
*
*
* --paper-menu-button-dropdown
* Mixin applied to the paper-menu-button dropdown
* {}
*
*
*
*/
@JsType
public interface PaperMenuButtonElement extends HTMLElement {
public static final String TAG = "paper-menu-button";
public static final String SRC = "paper-menu-button/paper-menu-button.html";
/**
* Hide the dropdown content.
*
* JavaScript Info:
* @method close
*
*/
void close();
/**
* An animation config. If provided, this will be used to animate the
closing of the dropdown.
*
* JavaScript Info:
* @property closeAnimationConfig
* @type Object
*
*/
@JsProperty JavaScriptObject getCloseAnimationConfig();
/**
* An animation config. If provided, this will be used to animate the
closing of the dropdown.
*
* JavaScript Info:
* @property closeAnimationConfig
* @type Object
*
*/
@JsProperty void setCloseAnimationConfig(JavaScriptObject value);
/**
* The content element that is contained by the menu button, if any.
*
* JavaScript Info:
* @method contentElement
*
*/
void contentElement();
/**
* The orientation against which to align the menu dropdown
horizontally relative to the dropdown trigger.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
@JsProperty String getHorizontalAlign();
/**
* The orientation against which to align the menu dropdown
horizontally relative to the dropdown trigger.
*
* JavaScript Info:
* @property horizontalAlign
* @type String
*
*/
@JsProperty void setHorizontalAlign(String value);
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
. Use a negative value to offset to the
left, or a positive value to offset to the right.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
*
*/
@JsProperty double getHorizontalOffset();
/**
* A pixel value that will be added to the position calculated for the
given horizontalAlign
. Use a negative value to offset to the
left, or a positive value to offset to the right.
*
* JavaScript Info:
* @property horizontalOffset
* @type Number
*
*/
@JsProperty void setHorizontalOffset(double value);
/**
* Set to true to disable automatically closing the dropdown after
a selection has been made.
*
* JavaScript Info:
* @property ignoreSelect
* @type Boolean
*
*/
@JsProperty boolean getIgnoreSelect();
/**
* Set to true to disable automatically closing the dropdown after
a selection has been made.
*
* JavaScript Info:
* @property ignoreSelect
* @type Boolean
*
*/
@JsProperty void setIgnoreSelect(boolean value);
/**
* Set to true to disable animations when opening and closing the
dropdown.
*
* JavaScript Info:
* @property noAnimations
* @type Boolean
*
*/
@JsProperty boolean getNoAnimations();
/**
* Set to true to disable animations when opening and closing the
dropdown.
*
* JavaScript Info:
* @property noAnimations
* @type Boolean
*
*/
@JsProperty void setNoAnimations(boolean value);
/**
* Make the dropdown content appear as an overlay positioned relative
to the dropdown trigger.
*
* JavaScript Info:
* @method open
*
*/
void open();
/**
* An animation config. If provided, this will be used to animate the
opening of the dropdown.
*
* JavaScript Info:
* @property openAnimationConfig
* @type Object
*
*/
@JsProperty JavaScriptObject getOpenAnimationConfig();
/**
* An animation config. If provided, this will be used to animate the
opening of the dropdown.
*
* JavaScript Info:
* @property openAnimationConfig
* @type Object
*
*/
@JsProperty void setOpenAnimationConfig(JavaScriptObject value);
/**
* True if the content is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty boolean getOpened();
/**
* True if the content is currently displayed.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty void setOpened(boolean value);
/**
* The orientation against which to align the menu dropdown
vertically relative to the dropdown trigger.
*
* JavaScript Info:
* @property verticalAlign
* @type String
*
*/
@JsProperty String getVerticalAlign();
/**
* The orientation against which to align the menu dropdown
vertically relative to the dropdown trigger.
*
* JavaScript Info:
* @property verticalAlign
* @type String
*
*/
@JsProperty void setVerticalAlign(String value);
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
. Use a negative value to offset towards the
top, or a positive value to offset towards the bottom.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
*
*/
@JsProperty double getVerticalOffset();
/**
* A pixel value that will be added to the position calculated for the
given verticalAlign
. Use a negative value to offset towards the
top, or a positive value to offset towards the bottom.
*
* JavaScript Info:
* @property verticalOffset
* @type Number
*
*/
@JsProperty void setVerticalOffset(double value);
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
*
*/
@JsProperty JavaScriptObject getHostAttributes();
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
*
*/
@JsProperty void setHostAttributes(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
@JsProperty JavaScriptObject getListeners();
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
@JsProperty void setListeners(JavaScriptObject value);
/**
* Can be used to imperatively add a key binding to the implementing
element. This is the imperative equivalent of declaring a keybinding
in the keyBindings
prototype property.
*
* JavaScript Info:
* @method addOwnKeyBinding
* @param {} eventString
* @param {} handlerName
* @behavior PaperTab
*/
void addOwnKeyBinding(JavaScriptObject eventString, JavaScriptObject handlerName);
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTab
*/
@JsProperty JavaScriptObject getKeyBindings();
/**
*
*
* JavaScript Info:
* @property keyBindings
* @type Object
* @behavior PaperTab
*/
@JsProperty void setKeyBindings(JavaScriptObject value);
/**
* The HTMLElement that will be firing relevant KeyboardEvents.
*
* JavaScript Info:
* @property keyEventTarget
* @type Object
* @behavior PaperTab
*/
@JsProperty JavaScriptObject getKeyEventTarget();
/**
* The HTMLElement that will be firing relevant KeyboardEvents.
*
* JavaScript Info:
* @property keyEventTarget
* @type Object
* @behavior PaperTab
*/
@JsProperty void setKeyEventTarget(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @method keyboardEventMatchesKeys
* @param {} event
* @param {} eventString
* @behavior PaperTab
*/
void keyboardEventMatchesKeys(JavaScriptObject event, JavaScriptObject eventString);
/**
* When called, will remove all imperatively-added key bindings.
*
* JavaScript Info:
* @method removeOwnKeyBindings
* @behavior PaperTab
*/
void removeOwnKeyBindings();
/**
*
*
* JavaScript Info:
* @method attached
* @behavior PaperTab
*/
void attached();
/**
*
*
* JavaScript Info:
* @method detached
* @behavior PaperTab
*/
void detached();
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperTab
*/
@JsProperty JsArray getObservers();
/**
*
*
* JavaScript Info:
* @property observers
* @type Array
* @behavior PaperTab
*/
@JsProperty void setObservers(JsArray value);
/**
*
*
* JavaScript Info:
* @method registered
* @behavior PaperTab
*/
void registered();
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getDisabled();
/**
* If true, the user cannot interact with this element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setDisabled(boolean value);
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty boolean getFocused();
/**
* If true, the element currently has focus.
*
* JavaScript Info:
* @property focused
* @type Boolean
* @behavior PaperTab
*/
@JsProperty void setFocused(boolean value);
}