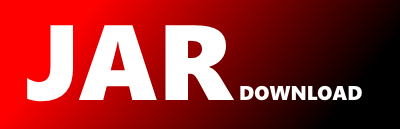
com.vaadin.polymer.paper.element.PaperTooltipElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from paper-tooltip project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.paper.element;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.core.client.js.JsProperty;
import com.google.gwt.core.client.js.JsType;
/**
* <paper-tooltip>
is a label that appears on hover and focus when the user
hovers over an element with the cursor or with the keyboard. It will be centered
to an anchor element specified in the for
attribute, or, if that doesn’t exist,
centered to the parent node containing it.
* Example:
* <div style="display:inline-block">
* <button>Click me!</button>
* <paper-tooltip>Tooltip text</paper-tooltip>
* </div>
*
* <div>
* <button id="btn">Click me!</button>
* <paper-tooltip for="btn">Tooltip text</paper-tooltip>
* </div>
*
*
*
The tooltip can be positioned on the top|bottom|left|right of the anchor using
the position
attribute. The default position is bottom.
* <paper-tooltip for="btn" position="left">Tooltip text</paper-tooltip>
* <paper-tooltip for="btn" position="top">Tooltip text</paper-tooltip>
*
*
*
Styling
* The following custom properties and mixins are available for styling:
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --paper-tooltip-background
* The background color of the tooltip
* #616161
*
*
* --paper-tooltip-opacity
* The opacity of the tooltip
* 0.9
*
*
* --paper-tooltip-text-color
* The text color of the tooltip
* white
*
*
* --paper-tooltip
* Mixin applied to the tooltip
* {}
*
*
*
*/
@JsType
public interface PaperTooltipElement extends HTMLElement {
public static final String TAG = "paper-tooltip";
public static final String SRC = "paper-tooltip/paper-tooltip.html";
/**
*
*
* JavaScript Info:
* @property animationConfig
* @type Object
*
*/
@JsProperty JavaScriptObject getAnimationConfig();
/**
*
*
* JavaScript Info:
* @property animationConfig
* @type Object
*
*/
@JsProperty void setAnimationConfig(JavaScriptObject value);
/**
* If true, no parts of the tooltip will ever be shown offscreen.
*
* JavaScript Info:
* @property fitToVisibleBounds
* @type Boolean
*
*/
@JsProperty boolean getFitToVisibleBounds();
/**
* If true, no parts of the tooltip will ever be shown offscreen.
*
* JavaScript Info:
* @property fitToVisibleBounds
* @type Boolean
*
*/
@JsProperty void setFitToVisibleBounds(boolean value);
/**
* The id of the element that the tooltip is anchored to. This element
must be a sibling of the tooltip.
*
* JavaScript Info:
* @property for
* @type String
*
*/
@JsProperty String getFor();
/**
* The id of the element that the tooltip is anchored to. This element
must be a sibling of the tooltip.
*
* JavaScript Info:
* @property for
* @type String
*
*/
@JsProperty void setFor(String value);
/**
*
*
* JavaScript Info:
* @method hide
*
*/
void hide();
/**
* This property is deprecated, but left over so that it doesn’t
break exiting code. Please use offset
instead. If both offset
and
marginTop
are provided, marginTop
will be ignored.
*
* JavaScript Info:
* @property marginTop
* @type Number
*
*/
@JsProperty double getMarginTop();
/**
* This property is deprecated, but left over so that it doesn’t
break exiting code. Please use offset
instead. If both offset
and
marginTop
are provided, marginTop
will be ignored.
*
* JavaScript Info:
* @property marginTop
* @type Number
*
*/
@JsProperty void setMarginTop(double value);
/**
* The spacing between the top of the tooltip and the element it is
anchored to.
*
* JavaScript Info:
* @property offset
* @type Number
*
*/
@JsProperty double getOffset();
/**
* The spacing between the top of the tooltip and the element it is
anchored to.
*
* JavaScript Info:
* @property offset
* @type Number
*
*/
@JsProperty void setOffset(double value);
/**
* Positions the tooltip to the top, right, bottom, left of its content.
*
* JavaScript Info:
* @property position
* @type String
*
*/
@JsProperty String getPosition();
/**
* Positions the tooltip to the top, right, bottom, left of its content.
*
* JavaScript Info:
* @property position
* @type String
*
*/
@JsProperty void setPosition(String value);
/**
*
*
* JavaScript Info:
* @method show
*
*/
void show();
/**
* Returns the target element that this tooltip is anchored to. It is
either the element given by the for
attribute, or the immediate parent
of the tooltip.
*
* JavaScript Info:
* @method target
*
*/
void target();
/**
*
*
* JavaScript Info:
* @method updatePosition
*
*/
void updatePosition();
/**
*
*
* JavaScript Info:
* @method attached
*
*/
void attached();
/**
*
*
* JavaScript Info:
* @method detached
*
*/
void detached();
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
*
*/
@JsProperty JavaScriptObject getHostAttributes();
/**
*
*
* JavaScript Info:
* @property hostAttributes
* @type Object
*
*/
@JsProperty void setHostAttributes(JavaScriptObject value);
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
@JsProperty JavaScriptObject getListeners();
/**
*
*
* JavaScript Info:
* @property listeners
* @type Object
*
*/
@JsProperty void setListeners(JavaScriptObject value);
/**
* Cancels the currently running animation.
*
* JavaScript Info:
* @method cancelAnimation
* @behavior NeonAnimatedPages
*/
void cancelAnimation();
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior NeonAnimatedPages
*/
@JsProperty String getEntryAnimation();
/**
* Convenience property for setting an ‘entry’ animation. Do not set animationConfig.entry
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property entryAnimation
* @type String
* @behavior NeonAnimatedPages
*/
@JsProperty void setEntryAnimation(String value);
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior NeonAnimatedPages
*/
@JsProperty String getExitAnimation();
/**
* Convenience property for setting an ‘exit’ animation. Do not set animationConfig.exit
manually if using this. The animated node is set to this
if using this property.
*
* JavaScript Info:
* @property exitAnimation
* @type String
* @behavior NeonAnimatedPages
*/
@JsProperty void setExitAnimation(String value);
/**
* An element implementing Polymer.NeonAnimationRunnerBehavior
calls this method to configure
an animation with an optional type. Elements implementing Polymer.NeonAnimatableBehavior
should define the property animationConfig
, which is either a configuration object
or a map of animation type to array of configuration objects.
*
* JavaScript Info:
* @method getAnimationConfig
* @param {} type
* @behavior NeonAnimatedPages
*/
void getAnimationConfig(JavaScriptObject type);
/**
* Plays an animation with an optional type
.
*
* JavaScript Info:
* @method playAnimation
* @param {string=} type
* @param {!Object=} cookie
* @behavior NeonAnimatedPages
*/
void playAnimation(JavaScriptObject type, JavaScriptObject cookie);
}