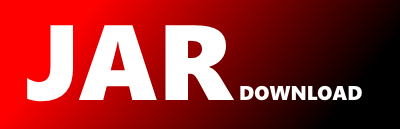
com.vaadin.polymer.vaadin.Column Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from vaadin-grid project by Vaadin Ltd
* that is licensed with Apache-2.0 license.
*/
package com.vaadin.polymer.vaadin;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Object for controlling and accessing a column in a vaadin-grid
.
* Example:
* var grid = document.querySelector('vaadin-grid');
*
* // First get a reference to one column object, which has
* // all the properties described in this documentation
* var column = grid.columns[0];
*
* // Then get or set any properties of the column, such as 'width'
* column.width = "200px";
*
*/
@JsType(isNative=true)
public interface Column {
@JsOverlay public static final String NAME = "Column";
@JsOverlay public static final String SRC = "vaadin-grid/vaadin-grid-doc.html";
/**
* Flex ratio of the column, used to distribute any available horizontal
space among all flexed columns.
* JavaScript example:
* grid.columns[0].flex = 2;
*
* Declarative example:
* <!-- If you omit the value of the attribute,
* it is considered as "1" -->
* <col>
* <col flex>
* <col flex="2">
*
*
* JavaScript Info:
* @property flex
* @type number
*
*/
@JsProperty double getFlex();
/**
* Flex ratio of the column, used to distribute any available horizontal
space among all flexed columns.
* JavaScript example:
* grid.columns[0].flex = 2;
*
* Declarative example:
* <!-- If you omit the value of the attribute,
* it is considered as "1" -->
* <col>
* <col flex>
* <col flex="2">
*
*
* JavaScript Info:
* @property flex
* @type number
*
*/
@JsProperty void setFlex(double value);
/**
* Set whether it is possible for the user to hide this column or not.
* JavaScript example:
* grid.columns[0].hidable = true;
*
* Declarative example:
* <col hidable>
*
*
* JavaScript Info:
* @property hidable
* @type boolean
*
*/
@JsProperty boolean getHidable();
/**
* Set whether it is possible for the user to hide this column or not.
* JavaScript example:
* grid.columns[0].hidable = true;
*
* Declarative example:
* <col hidable>
*
*
* JavaScript Info:
* @property hidable
* @type boolean
*
*/
@JsProperty void setHidable(boolean value);
/**
* Hides or shows the column. By default columns are visible before
explicitly hiding them. A column can be programmatically hidden even if
the hidable
property would be false
.
* JavaScript example:
* grid.columns[0].hidden = true;
*
* Declarative example:
* <col hidden>
*
*
* JavaScript Info:
* @property hidden
* @type boolean
*
*/
@JsProperty boolean getHidden();
/**
* Hides or shows the column. By default columns are visible before
explicitly hiding them. A column can be programmatically hidden even if
the hidable
property would be false
.
* JavaScript example:
* grid.columns[0].hidden = true;
*
* Declarative example:
* <col hidden>
*
*
* JavaScript Info:
* @property hidden
* @type boolean
*
*/
@JsProperty void setHidden(boolean value);
/**
* Maximum width of the column in pixels.
* JavaScript example:
* grid.columns[0].maxWidth = 400;
*
* Declarative example:
* <col max-width="400">
*
*
* JavaScript Info:
* @property maxWidth
* @type number
*
*/
@JsProperty double getMaxWidth();
/**
* Maximum width of the column in pixels.
* JavaScript example:
* grid.columns[0].maxWidth = 400;
*
* Declarative example:
* <col max-width="400">
*
*
* JavaScript Info:
* @property maxWidth
* @type number
*
*/
@JsProperty void setMaxWidth(double value);
/**
* Minimum width of the column in pixels.
* JavaScript example:
* grid.columns[0].minWidth = 100;
*
* Declarative example:
* <col min-width="100">
*
*
* JavaScript Info:
* @property minWidth
* @type number
*
*/
@JsProperty double getMinWidth();
/**
* Minimum width of the column in pixels.
* JavaScript example:
* grid.columns[0].minWidth = 100;
*
* Declarative example:
* <col min-width="100">
*
*
* JavaScript Info:
* @property minWidth
* @type number
*
*/
@JsProperty void setMinWidth(double value);
/**
* Custom render function for the column’s data items. Default is
undefined.
* Currently it is not possible to set the renderer declaratively.
* JavaScript example:
* // Prefix the cell content with a dollar sign
* grid.columns[0].renderer = function(cell) {
* cell.element.innerHTML = '$' + cell.data;
* };
*
*
* JavaScript Info:
* @property renderer
* @type Function
*
*/
@JsProperty Function getRenderer();
/**
* Custom render function for the column’s data items. Default is
undefined.
* Currently it is not possible to set the renderer declaratively.
* JavaScript example:
* // Prefix the cell content with a dollar sign
* grid.columns[0].renderer = function(cell) {
* cell.element.innerHTML = '$' + cell.data;
* };
*
*
* JavaScript Info:
* @property renderer
* @type Function
*
*/
@JsProperty void setRenderer(Function value);
/**
* Enables sorting the column data by the end user.
* To programmatically or declaratively sort the columns, use the
grid.sortOrder
property, or the sort-direction
attribute. Columns
can be sorted even if this property is false
, it only controls the
end user sorting.
* JavaScript example:
* grid.columns[0].sortable = true;
* grid.sortOrder = [{column: 0, direction: "asc"}];
*
* Declarative example:
* <col sortable sort-direction="asc">
*
*
* JavaScript Info:
* @property sortable
* @type boolean
*
*/
@JsProperty boolean getSortable();
/**
* Enables sorting the column data by the end user.
* To programmatically or declaratively sort the columns, use the
grid.sortOrder
property, or the sort-direction
attribute. Columns
can be sorted even if this property is false
, it only controls the
end user sorting.
* JavaScript example:
* grid.columns[0].sortable = true;
* grid.sortOrder = [{column: 0, direction: "asc"}];
*
* Declarative example:
* <col sortable sort-direction="asc">
*
*
* JavaScript Info:
* @property sortable
* @type boolean
*
*/
@JsProperty void setSortable(boolean value);
/**
* Width of the column in pixels.
* JavaScript example:
* grid.columns[0].width = 200;
*
* Declarative example:
* <col width="200">
*
*
* JavaScript Info:
* @property width
* @type number
*
*/
@JsProperty double getWidth();
/**
* Width of the column in pixels.
* JavaScript example:
* grid.columns[0].width = 200;
*
* Declarative example:
* <col width="200">
*
*
* JavaScript Info:
* @property width
* @type number
*
*/
@JsProperty void setWidth(double value);
/**
* Sets the hiding toggle’s caption for this column. Shown in the grid’s
column hiding dropdown menu if the column is hidable. Useful if the
header content is something else than text (i.e. a DOM element) or
empty.
* If not specified, the default column header content/text is used.
* JavaScript example:
* grid.columns[0].hidingToggleText = "Profile picture";
*
* Declarative example:
* <col hiding-toggle-text="Profile picture">
*
*
* JavaScript Info:
* @property hidingToggleText
* @type string
*
*/
@JsProperty String getHidingToggleText();
/**
* Sets the hiding toggle’s caption for this column. Shown in the grid’s
column hiding dropdown menu if the column is hidable. Useful if the
header content is something else than text (i.e. a DOM element) or
empty.
* If not specified, the default column header content/text is used.
* JavaScript example:
* grid.columns[0].hidingToggleText = "Profile picture";
*
* Declarative example:
* <col hiding-toggle-text="Profile picture">
*
*
* JavaScript Info:
* @property hidingToggleText
* @type string
*
*/
@JsProperty void setHidingToggleText(String value);
/**
* Name of the column, used in mapping the column to a property in the row
item/object provided by the items
property.
* Must be a valid JavaScript object reference
(e.g. “user.name.first”, “url”)
* JavaScript example:
* grid.columns[0].name = "user.name.first";
*
* Declarative example:
* <col name="user.name.first">
*
*
* JavaScript Info:
* @property name
* @type string
*
*/
@JsProperty String getName();
/**
* Name of the column, used in mapping the column to a property in the row
item/object provided by the items
property.
* Must be a valid JavaScript object reference
(e.g. “user.name.first”, “url”)
* JavaScript example:
* grid.columns[0].name = "user.name.first";
*
* Declarative example:
* <col name="user.name.first">
*
*
* JavaScript Info:
* @property name
* @type string
*
*/
@JsProperty void setName(String value);
}