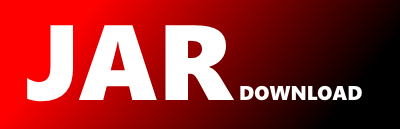
com.vaadin.polymer.vaadin.VaadinUploadElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from vaadin-upload project by Vaadin Ltd
* that is licensed with Apache-2.0 license.
*/
package com.vaadin.polymer.vaadin;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* <vaadin-upload>
is a Polymer element for uploading multiple files with drag and drop support.
* Example:
* <vaadin-upload></vaadin-upload>
*
* Styling
* The following custom properties are available for styling the component.
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --vaadin-upload-drag-ripple
* A mixin that is applied to the ripple animation in the drop area
* {}
*
*
* --vaadin-upload-drop-label
* A mixin that is applied to the drop label
* {}
*
*
* --vaadin-upload-drop-label-dragover
* A mixin that is applied to the drop label when overing the component with files
* {}
*
*
* --vaadin-upload-drop-label-icon
* A mixin that is applied to the drop icon
* {}
*
*
* --vaadin-upload-file-list
* A mixin that is applied to the file list
* {}
*
*
* --vaadin-upload-buttons
* A mixin that is applied to the buttons container
* {}
*
*
* --vaadin-upload-buttons-primary
* A mixin that is applied to the primary buttons container (left side)
* {}
*
*
* --vaadin-upload-buttons-secondary
* A mixin that is applied to the secondary buttons container (right side)
* {}
*
*
* --vaadin-upload-button-add
* A mixin that is applied to the upload button
* {}
*
*
* --vaadin-upload-button-add-disabled
* A mixin that is applied to the upload button when maxFiles
limit is reached
* {}
*
*
*
*/
@JsType(isNative=true)
public interface VaadinUploadElement extends HTMLElement {
@JsOverlay public static final String TAG = "vaadin-upload";
@JsOverlay public static final String SRC = "vaadin-upload/vaadin-upload.html";
/**
* Key-Value map to send to the server. If you set this property as an
attribute, use a valid JSON string, for example:
* <vaadin-upload headers='{"X-Foo": "Bar"}'></vaadin-upload>
*
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty JavaScriptObject getHeaders();
/**
* Key-Value map to send to the server. If you set this property as an
attribute, use a valid JSON string, for example:
* <vaadin-upload headers='{"X-Foo": "Bar"}'></vaadin-upload>
*
*
* JavaScript Info:
* @property headers
* @type Object
*
*/
@JsProperty void setHeaders(JavaScriptObject value);
/**
* The object used to localize this component.
For changing the default localization, change the entire
i18n object or just the property you want to modify.
* The object has the following JSON structure and default values:
* {
* dropFiles: {
* one: 'Drop file here...',
* many: 'Drop files here...'
* },
* addFiles: {
* one: 'Select File',
* many: 'Upload Files'
* },
* cancel: 'Cancel',
* error: {
* tooManyFiles: 'Too Many Files.',
* fileIsTooBig: 'File is Too Big.',
* incorrectFileType: 'Incorrect File Type.'
* },
* uploading: {
* status: {
* connecting: 'Connecting...',
* stalled: 'Stalled.',
* processing: 'Processing File...'
* },
* remainingTime: {
* prefix: 'remaining time: ',
* unknown: 'unknown remaining time'
* },
* error: {
* serverUnavailable: 'Server Unavailable',
* unexpectedServerError: 'Unexpected Server Error',
* forbidden: 'Forbidden'
* }
* },
* units: {
* size: ['B', 'kB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB']
* },
* formatSize: function(bytes) {
* // returns the size followed by the best suitable unit
* },
* formatTime: function(seconds, [secs, mins, hours]) {
* // returns a 'HH:MM:SS' string
* }
* }
*
*
*
*
*
* JavaScript Info:
* @property i18n
* @type Object
*
*/
@JsProperty JavaScriptObject getI18n();
/**
* The object used to localize this component.
For changing the default localization, change the entire
i18n object or just the property you want to modify.
* The object has the following JSON structure and default values:
* {
* dropFiles: {
* one: 'Drop file here...',
* many: 'Drop files here...'
* },
* addFiles: {
* one: 'Select File',
* many: 'Upload Files'
* },
* cancel: 'Cancel',
* error: {
* tooManyFiles: 'Too Many Files.',
* fileIsTooBig: 'File is Too Big.',
* incorrectFileType: 'Incorrect File Type.'
* },
* uploading: {
* status: {
* connecting: 'Connecting...',
* stalled: 'Stalled.',
* processing: 'Processing File...'
* },
* remainingTime: {
* prefix: 'remaining time: ',
* unknown: 'unknown remaining time'
* },
* error: {
* serverUnavailable: 'Server Unavailable',
* unexpectedServerError: 'Unexpected Server Error',
* forbidden: 'Forbidden'
* }
* },
* units: {
* size: ['B', 'kB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB']
* },
* formatSize: function(bytes) {
* // returns the size followed by the best suitable unit
* },
* formatTime: function(seconds, [secs, mins, hours]) {
* // returns a 'HH:MM:SS' string
* }
* }
*
*
*
*
*
* JavaScript Info:
* @property i18n
* @type Object
*
*/
@JsProperty void setI18n(JavaScriptObject value);
/**
* The array of files being processed, or already uploaded.
* Each element is a File
object with a number of extra properties to track the upload process:
*
* uploadTarget
: The target URL used to upload this file.
* elapsed
: Elapsed time since the upload started.
* elapsedStr
: Human-readable elapsed time.
* remaining
: Number of seconds remaining for the upload to finish.
* remainingStr
: Human-readable remaining time for the upload to finish.
* progress
: Percentage of the file already uploaded.
* speed
: Upload speed in kB/s.
* size
: File size in bytes.
* totalStr
: Human-readable total size of the file.
* loaded
: Bytes transferred so far.
* loadedStr
: Human-readable uploaded size at the moment.
* status
: Status of the upload process.
* error
: Error message in case the upload failed.
* abort
: True if the file was canceled by the user.
* complete
: True when the file was transferred to the server.
* uploading
: True while trasferring data to the server.
*
*
* JavaScript Info:
* @property files
* @type Array
*
*/
@JsProperty JsArray getFiles();
/**
* The array of files being processed, or already uploaded.
* Each element is a File
object with a number of extra properties to track the upload process:
*
* uploadTarget
: The target URL used to upload this file.
* elapsed
: Elapsed time since the upload started.
* elapsedStr
: Human-readable elapsed time.
* remaining
: Number of seconds remaining for the upload to finish.
* remainingStr
: Human-readable remaining time for the upload to finish.
* progress
: Percentage of the file already uploaded.
* speed
: Upload speed in kB/s.
* size
: File size in bytes.
* totalStr
: Human-readable total size of the file.
* loaded
: Bytes transferred so far.
* loadedStr
: Human-readable uploaded size at the moment.
* status
: Status of the upload process.
* error
: Error message in case the upload failed.
* abort
: True if the file was canceled by the user.
* complete
: True when the file was transferred to the server.
* uploading
: True while trasferring data to the server.
*
*
* JavaScript Info:
* @property files
* @type Array
*
*/
@JsProperty void setFiles(JsArray value);
/**
* Define whether the element supports dropping files on it for uploading.
By default it’s enabled in desktop and disabled in touch devices
because mobile devices do not support drag events in general. Setting
it false means that drop is enabled even in touch-devices, and true
disables drop in all devices.
*
* JavaScript Info:
* @property nodrop
* @type Boolean
*
*/
@JsProperty boolean getNodrop();
/**
* Define whether the element supports dropping files on it for uploading.
By default it’s enabled in desktop and disabled in touch devices
because mobile devices do not support drag events in general. Setting
it false means that drop is enabled even in touch-devices, and true
disables drop in all devices.
*
* JavaScript Info:
* @property nodrop
* @type Boolean
*
*/
@JsProperty void setNodrop(boolean value);
/**
* Specifies the maximum file size in bytes allowed to upload.
Notice that it is a client-side constraint, which will be checked before
sending the request. Obviously you need to do the same validation in
the server-side and be sure that they are aligned.
*
* JavaScript Info:
* @property maxFileSize
* @type Number
*
*/
@JsProperty double getMaxFileSize();
/**
* Specifies the maximum file size in bytes allowed to upload.
Notice that it is a client-side constraint, which will be checked before
sending the request. Obviously you need to do the same validation in
the server-side and be sure that they are aligned.
*
* JavaScript Info:
* @property maxFileSize
* @type Number
*
*/
@JsProperty void setMaxFileSize(double value);
/**
* Limit of files to upload, by default it is unlimited. If the value is
set to one, native file browser will prevent selecting multiple files.
*
* JavaScript Info:
* @property maxFiles
* @type Number
*
*/
@JsProperty double getMaxFiles();
/**
* Limit of files to upload, by default it is unlimited. If the value is
set to one, native file browser will prevent selecting multiple files.
*
* JavaScript Info:
* @property maxFiles
* @type Number
*
*/
@JsProperty void setMaxFiles(double value);
/**
* Max time in milliseconds for the entire upload process, if exceeded the
request will be aborted. Zero means that there is no timeout.
*
* JavaScript Info:
* @property timeout
* @type Number
*
*/
@JsProperty double getTimeout();
/**
* Max time in milliseconds for the entire upload process, if exceeded the
request will be aborted. Zero means that there is no timeout.
*
* JavaScript Info:
* @property timeout
* @type Number
*
*/
@JsProperty void setTimeout(double value);
/**
* HTTP Method used to send the files. Only POST and PUT are allowed.
*
* JavaScript Info:
* @property method
* @type String
*
*/
@JsProperty String getMethod();
/**
* HTTP Method used to send the files. Only POST and PUT are allowed.
*
* JavaScript Info:
* @property method
* @type String
*
*/
@JsProperty void setMethod(String value);
/**
* The server URL. The default value is an empty string, which means that
window.location will be used.
*
* JavaScript Info:
* @property target
* @type String
*
*/
@JsProperty String getTarget();
/**
* The server URL. The default value is an empty string, which means that
window.location will be used.
*
* JavaScript Info:
* @property target
* @type String
*
*/
@JsProperty void setTarget(String value);
/**
* Specifies the ‘name’ property at Content-Disposition
*
* JavaScript Info:
* @property formDataName
* @type String
*
*/
@JsProperty String getFormDataName();
/**
* Specifies the ‘name’ property at Content-Disposition
*
* JavaScript Info:
* @property formDataName
* @type String
*
*/
@JsProperty void setFormDataName(String value);
/**
* Specifies the types of files that the server accepts.
Syntax: a comma-separated list of MIME type patterns (wildcards are
allowed) or file extensions.
Notice that MIME types are widely supported, while file extensions
are only implemented in certain browsers, so avoid using it.
Example: accept=”video/*,image/tiff” or accept=”.pdf,audio/mp3”
*
* JavaScript Info:
* @property accept
* @type String
*
*/
@JsProperty String getAccept();
/**
* Specifies the types of files that the server accepts.
Syntax: a comma-separated list of MIME type patterns (wildcards are
allowed) or file extensions.
Notice that MIME types are widely supported, while file extensions
are only implemented in certain browsers, so avoid using it.
Example: accept=”video/*,image/tiff” or accept=”.pdf,audio/mp3”
*
* JavaScript Info:
* @property accept
* @type String
*
*/
@JsProperty void setAccept(String value);
}