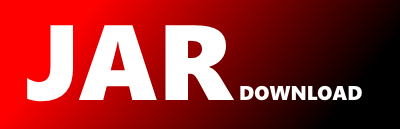
com.vaadin.polymer.vaadin.widget.VaadinUploadFile Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from vaadin-upload project by Vaadin Ltd
* that is licensed with Apache-2.0 license.
*/
package com.vaadin.polymer.vaadin.widget;
import com.vaadin.polymer.vaadin.*;
import com.vaadin.polymer.vaadin.widget.event.FileAbortEvent;
import com.vaadin.polymer.vaadin.widget.event.FileAbortEventHandler;
import com.vaadin.polymer.vaadin.widget.event.FileRemoveEvent;
import com.vaadin.polymer.vaadin.widget.event.FileRemoveEventHandler;
import com.vaadin.polymer.vaadin.widget.event.FileRetryEvent;
import com.vaadin.polymer.vaadin.widget.event.FileRetryEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* <vaadin-upload-file>
element represents a file in the file list of <vaadin-upload>
.
* Styling
* The following custom properties are available for styling the component.
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --vaadin-upload-file
* A mixin that is applied to the host element
* {}
*
*
* --vaadin-upload-file-row
* A mixin that is applied to the file row
* {}
*
*
* --vaadin-upload-file-status-icon
* A mixin that is applied to all file status icons. By default, file status icons are hidden until the upload process finishes.
* {}
*
*
* --vaadin-upload-file-status-icon-complete
* A mixin that is applied to the complete status icon when the upload process succeeds
* {}
*
*
* --vaadin-upload-file-status-icon-error
* A mixin that is applied to the error status icon when the upload process fails
* {}
*
*
* --vaadin-upload-file-meta
* A mixin that is applied to the info container
* {}
*
*
* --vaadin-upload-file-meta-complete
* A mixin that is applied to the info container when file upload is complete
* {}
*
*
* --vaadin-upload-file-meta-error
* A mixin that is applied to the info container when an error happens
* {}
*
*
* --vaadin-upload-file-name
* A mixin that is applied to the file name
* {}
*
*
* --vaadin-upload-file-status
* A mixin that is applied to the file status label
* {}
*
*
* --vaadin-upload-file-error
* A mixin that is applied to the file error label
* {}
*
*
* --vaadin-upload-file-commands
* A mixin that is applied to the buttons container
* {}
*
*
* --vaadin-upload-file-progress
* A mixin that is applied to the included paper-progress
* {}
*
*
* --vaadin-upload-file-progress-error
* A mixin that is applied to the progress bar when error is set
* {}
*
*
* --vaadin-upload-file-progress-indeterminate
* A mixin that is applied to the progress bar when indeterminate
* {}
*
*
* --vaadin-upload-file-progress-uploading-indeterminate
* A mixin that is applied to the progress bar when uploading and indeterminate
* {}
*
*
* --vaadin-upload-file-progress-complete
* A mixin that is applied to the progress when file is complete
* {}
*
*
* --vaadin-upload-file-canceled
* A mixin that is applied to the upload cancel animation
* {}
*
*
*
*/
public class VaadinUploadFile extends PolymerWidget {
/**
* Default Constructor.
*/
public VaadinUploadFile() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public VaadinUploadFile(String html) {
super(VaadinUploadFileElement.TAG, VaadinUploadFileElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public VaadinUploadFileElement getPolymerElement() {
return (VaadinUploadFileElement) getElement();
}
/**
* File metadata, upload status and progress information.
*
* JavaScript Info:
* @property file
* @type Object
*
*/
public JavaScriptObject getFile() {
return getPolymerElement().getFile();
}
/**
* File metadata, upload status and progress information.
*
* JavaScript Info:
* @property file
* @type Object
*
*/
public void setFile(JavaScriptObject value) {
getPolymerElement().setFile(value);
}
// Needed in UIBinder
/**
* File metadata, upload status and progress information.
*
* JavaScript Info:
* @attribute file
*
*/
public void setFile(String value) {
Polymer.property(this.getPolymerElement(), "file", value);
}
/**
* Fired when abort button is pressed. It is listened by vaadin-upload
which
will abort the upload in progress, but will not remove the file from the list
to allow the animation to hide the element to be run.
*
* JavaScript Info:
* @event file-abort
*/
public HandlerRegistration addFileAbortHandler(FileAbortEventHandler handler) {
return addDomHandler(handler, FileAbortEvent.TYPE);
}
/**
* Fired after the animation to hide the element has finished. It is listened
by vaadin-upload
which will actually remove the file from the upload
file list.
*
* JavaScript Info:
* @event file-remove
*/
public HandlerRegistration addFileRemoveHandler(FileRemoveEventHandler handler) {
return addDomHandler(handler, FileRemoveEvent.TYPE);
}
/**
* Fired when the retry button is pressed. It is listened by vaadin-upload
which will start a new upload process of this file.
*
* JavaScript Info:
* @event file-retry
*/
public HandlerRegistration addFileRetryHandler(FileRetryEventHandler handler) {
return addDomHandler(handler, FileRetryEvent.TYPE);
}
}