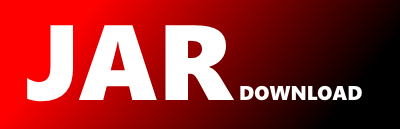
com.vaadin.polymer.app.AppDrawerElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-layout project by The Polymer Authors
* that is licensed with https://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* app-drawer is a navigation drawer that can slide in from the left or right.
* Example:
* Align the drawer at the start, which is left in LTR layouts (default):
* <app-drawer opened></app-drawer>
*
* Align the drawer at the end:
* <app-drawer align="end" opened></app-drawer>
*
* To make the contents of the drawer scrollable, create a wrapper for the scroll
content, and apply height and overflow styles to it.
* <app-drawer>
* <div style="height: 100%; overflow: auto;"></div>
* </app-drawer>
*
* Styling
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --app-drawer-width
* Width of the drawer
* 256px
*
*
* --app-drawer-content-container
* Mixin for the drawer content container
* {}
*
*
* --app-drawer-scrim-background
* Background for the scrim
* rgba(0, 0, 0, 0.5)
*
*
*
*/
@JsType(isNative=true)
public interface AppDrawerElement extends HTMLElement {
@JsOverlay public static final String TAG = "app-drawer";
@JsOverlay public static final String SRC = "app-layout/app-layout.html";
/**
* The transition duration of the drawer in milliseconds.
*
* JavaScript Info:
* @property transitionDuration
* @type Number
*
*/
@JsProperty double getTransitionDuration();
/**
* The transition duration of the drawer in milliseconds.
*
* JavaScript Info:
* @property transitionDuration
* @type Number
*
*/
@JsProperty void setTransitionDuration(double value);
/**
* Trap keyboard focus when the drawer is opened and not persistent.
*
* JavaScript Info:
* @property noFocusTrap
* @type Boolean
*
*/
@JsProperty boolean getNoFocusTrap();
/**
* Trap keyboard focus when the drawer is opened and not persistent.
*
* JavaScript Info:
* @property noFocusTrap
* @type Boolean
*
*/
@JsProperty void setNoFocusTrap(boolean value);
/**
* Disables swiping on the drawer.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
@JsProperty boolean getDisableSwipe();
/**
* Disables swiping on the drawer.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
@JsProperty void setDisableSwipe(boolean value);
/**
* Create an area at the edge of the screen to swipe open the drawer.
*
* JavaScript Info:
* @property swipeOpen
* @type Boolean
*
*/
@JsProperty boolean getSwipeOpen();
/**
* Create an area at the edge of the screen to swipe open the drawer.
*
* JavaScript Info:
* @property swipeOpen
* @type Boolean
*
*/
@JsProperty void setSwipeOpen(boolean value);
/**
* The opened state of the drawer.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty boolean getOpened();
/**
* The opened state of the drawer.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
@JsProperty void setOpened(boolean value);
/**
* The drawer does not have a scrim and cannot be swiped close.
*
* JavaScript Info:
* @property persistent
* @type Boolean
*
*/
@JsProperty boolean getPersistent();
/**
* The drawer does not have a scrim and cannot be swiped close.
*
* JavaScript Info:
* @property persistent
* @type Boolean
*
*/
@JsProperty void setPersistent(boolean value);
/**
* The computed, read-only position of the drawer on the screen (‘left’ or ‘right’).
*
* JavaScript Info:
* @property position
* @type String
*
*/
@JsProperty String getPosition();
/**
* The computed, read-only position of the drawer on the screen (‘left’ or ‘right’).
*
* JavaScript Info:
* @property position
* @type String
*
*/
@JsProperty void setPosition(String value);
/**
* The alignment of the drawer on the screen (‘left’, ‘right’, ‘start’ or ‘end’).
‘start’ computes to left and ‘end’ to right in LTR layout and vice versa in RTL
layout.
*
* JavaScript Info:
* @property align
* @type String
*
*/
@JsProperty String getAlign();
/**
* The alignment of the drawer on the screen (‘left’, ‘right’, ‘start’ or ‘end’).
‘start’ computes to left and ‘end’ to right in LTR layout and vice versa in RTL
layout.
*
* JavaScript Info:
* @property align
* @type String
*
*/
@JsProperty void setAlign(String value);
/**
* Opens the drawer.
*
* JavaScript Info:
* @method open
*
*
*/
void open();
/**
* Closes the drawer.
*
* JavaScript Info:
* @method close
*
*
*/
void close();
/**
* Resets the layout. The event fired is used by app-drawer-layout to position the
content.
*
* JavaScript Info:
* @method resetLayout
*
*
*/
void resetLayout();
/**
* Toggles the drawer open and close.
*
* JavaScript Info:
* @method toggle
*
*
*/
void toggle();
/**
* Gets the width of the drawer.
*
* JavaScript Info:
* @method getWidth
*
* @return {double}
*/
double getWidth();
}