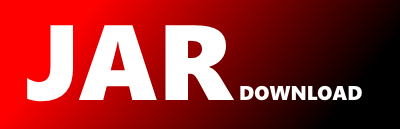
com.vaadin.polymer.app.AppHeaderElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-layout project by The Polymer Authors
* that is licensed with https://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* app-header is container element for app-toolbars at the top of the screen that can have scroll
effects. By default, an app-header moves away from the viewport when scrolling down and
if using reveals
, the header slides back when scrolling back up. For example:
* <app-header reveals>
* <app-toolbar>
* <div main-title>App name</div>
* </app-toolbar>
* </app-header>
*
* app-header can also condense when scrolling down. To achieve this behavior, the header
must have a larger height than the sticky
element in the light DOM. For example:
* <app-header style="height: 96px;" condenses fixed>
* <app-toolbar style="height: 64px;">
* <div main-title>App name</div>
* </app-toolbar>
* </app-header>
*
* In this case the header is initially 96px
tall, and it shrinks to 64px
when scrolling down.
That is what is meant by “condensing”.
* Sticky element
* The element that is positioned fixed to top of the header’s scrollTarget
when a threshold
is reached, similar to position: sticky
in CSS. This element must be an immediate
child of app-header. By default, the sticky
element is the first `app-toolbar that
is an immediate child of app-header.
* <app-header condenses>
* <app-toolbar> Sticky element </app-toolbar>
* </app-header>
*
* Customizing the sticky element
* <app-header condenses>
* <app-toolbar></app-toolbar>
* <app-toolbar sticky> Sticky element </app-toolbar>
* </app-header>
*
* Scroll target
* The app-header’s scrollTarget
property allows to customize the scrollable element to which
the header responds when the user scrolls. By default, app-header uses the document as
the scroll target, but you can customize this property by setting the id of the element, e.g.
* <div id="scrollingRegion" style="overflow-y: auto;">
* <app-header scroll-target="scrollingRegion">
* </app-header>
* </div>
*
* In this case, the scrollTarget
property points to the outer div element. Alternatively,
you can set this property programmatically:
* appHeader.scrollTarget = document.querySelector("#scrollingRegion");
*
* Backgrounds
* app-header has two background layers that can be used for styling when the header is condensed
or when the scrollable element is scrolled to the top.
* Scroll effects
* Scroll effects are optional visual effects applied in app-header based on scroll position. For example,
The Material Design scrolling techniques
recommends effects that can be installed via the effects
property. e.g.
* <app-header effects="waterfall">
* <app-toolbar>App name</app-toolbar>
* </app-header>
*
* Importing the effects
* To use the scroll effects, you must explicitly import them in addition to app-header
:
* <link rel="import" href="/bower_components/app-layout/app-scroll-effects/app-scroll-effects.html">
*
* List of effects
* blend-background
Fades in/out two background elements by applying CSS opacity based on scroll position.
You can use this effect to smoothly change the background color or image of the header.
For example, using the mixin --app-header-background-rear-layer
lets you assign a different
background when the header is condensed:
* app-header {
* background-color: red;
* --app-header-background-rear-layer: {
* /* The header is blue when condensed * /
* background-color: blue;
* };
* }
*
* fade-background
Upon scrolling past a threshold, this effect will trigger an opacity transition to
fade in/out the backgrounds. Compared to the blend-background
effect,
this effect doesn’t interpolate the opacity based on scroll position.
* parallax-background
A simple parallax effect that vertically translates the backgrounds based on a fraction
of the scroll position. For example:
* app-header {
* --app-header-background-front-layer: {
* background-image: url(...);
* };
* }
*
* <app-header style="height: 300px;" effects="parallax-background">
* <app-toolbar>App name</app-toolbar>
* </app-header>
*
* The fraction determines how far the background moves relative to the scroll position.
This value can be assigned via the scalar
config value and it is typically a value
between 0 and 1 inclusive. If scalar=0
, the background doesn’t move away from the header.
* resize-title
Progressively interpolates the size of the title from the element with the main-title
attribute
to the element with the condensed-title
attribute as the header condenses. For example:
* <app-header condenses reveals effects="resize-title">
* <app-toolbar>
* <h4 condensed-title>App name</h4>
* </app-toolbar>
* <app-toolbar>
* <h1 main-title>App name</h1>
* </app-toolbar>
* </app-header>
*
* resize-snapped-title
Upon scrolling past a threshold, this effect fades in/out the titles using opacity transitions.
Similarly to resize-title
, the main-title
and condensed-title
elements must be placed in the
light DOM.
* waterfall
Toggles the shadow property in app-header to create a sense of depth (as recommended in the
MD spec) between the header and the underneath content. You can change the shadow by
customizing the --app-header-shadow
mixin. For example:
* app-header {
* --app-header-shadow: {
* box-shadow: inset 0px 5px 2px -3px rgba(0, 0, 0, 0.2);
* };
* }
*
* <app-header condenses reveals effects="waterfall">
* <app-toolbar>
* <h1 main-title>App name</h1>
* </app-toolbar>
* </app-header>
*
* material
Installs the waterfall, resize-title, blend-background and parallax-background effects.
* Content attributes
*
*
*
* Attribute
* Description
* Default
*
*
*
*
* sticky
* Element that remains at the top when the header condenses.
* The first app-toolbar in the light DOM.
*
*
*
* Styling
*
*
*
* Mixin
* Description
* Default
*
*
*
*
* --app-header-background-front-layer
* Applies to the front layer of the background.
* {}
*
*
* --app-header-background-rear-layer
* Applies to the rear layer of the background.
* {}
*
*
* --app-header-shadow
* Applies to the shadow.
* {}
*
*
*
*/
@JsType(isNative=true)
public interface AppHeaderElement extends HTMLElement {
@JsOverlay public static final String TAG = "app-header";
@JsOverlay public static final String SRC = "app-layout/app-layout.html";
/**
* If true, the header will automatically collapse when scrolling down.
That is, the sticky
element remains visible when the header is fully condensed
whereas the rest of the elements will collapse below sticky
element.
* By default, the sticky
element is the first toolbar in the light DOM:
* <app-header condenses>
* <app-toolbar>This toolbar remains on top</app-toolbar>
* <app-toolbar></app-toolbar>
* <app-toolbar></app-toolbar>
* </app-header>
*
* Additionally, you can specify which toolbar or element remains visible in condensed mode
by adding the sticky
attribute to that element. For example: if we want the last
toolbar to remain visible, we can add the sticky
attribute to it.
* <app-header condenses>
* <app-toolbar></app-toolbar>
* <app-toolbar></app-toolbar>
* <app-toolbar sticky>This toolbar remains on top</app-toolbar>
* </app-header>
*
* Note the sticky
element must be a direct child of app-header
.
*
* JavaScript Info:
* @property condenses
* @type Boolean
*
*/
@JsProperty boolean getCondenses();
/**
* If true, the header will automatically collapse when scrolling down.
That is, the sticky
element remains visible when the header is fully condensed
whereas the rest of the elements will collapse below sticky
element.
* By default, the sticky
element is the first toolbar in the light DOM:
* <app-header condenses>
* <app-toolbar>This toolbar remains on top</app-toolbar>
* <app-toolbar></app-toolbar>
* <app-toolbar></app-toolbar>
* </app-header>
*
* Additionally, you can specify which toolbar or element remains visible in condensed mode
by adding the sticky
attribute to that element. For example: if we want the last
toolbar to remain visible, we can add the sticky
attribute to it.
* <app-header condenses>
* <app-toolbar></app-toolbar>
* <app-toolbar></app-toolbar>
* <app-toolbar sticky>This toolbar remains on top</app-toolbar>
* </app-header>
*
* Note the sticky
element must be a direct child of app-header
.
*
* JavaScript Info:
* @property condenses
* @type Boolean
*
*/
@JsProperty void setCondenses(boolean value);
/**
* Slides back the header when scrolling back up.
*
* JavaScript Info:
* @property reveals
* @type Boolean
*
*/
@JsProperty boolean getReveals();
/**
* Slides back the header when scrolling back up.
*
* JavaScript Info:
* @property reveals
* @type Boolean
*
*/
@JsProperty void setReveals(boolean value);
/**
* Displays a shadow below the header.
*
* JavaScript Info:
* @property shadow
* @type Boolean
*
*/
@JsProperty boolean getShadow();
/**
* Displays a shadow below the header.
*
* JavaScript Info:
* @property shadow
* @type Boolean
*
*/
@JsProperty void setShadow(boolean value);
/**
* Disables CSS transitions and scroll effects on the element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior AppBox
*/
@JsProperty boolean getDisabled();
/**
* Disables CSS transitions and scroll effects on the element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior AppBox
*/
@JsProperty void setDisabled(boolean value);
/**
* Mantains the header fixed at the top so it never moves away.
*
* JavaScript Info:
* @property fixed
* @type Boolean
*
*/
@JsProperty boolean getFixed();
/**
* Mantains the header fixed at the top so it never moves away.
*
* JavaScript Info:
* @property fixed
* @type Boolean
*
*/
@JsProperty void setFixed(boolean value);
/**
* Allows to set a scrollTop
threshold. When greater than 0, thresholdTriggered
is true only when the scroll target’s scrollTop
has reached this value.
* For example, if threshold = 100
, thresholdTriggered
is true when the scrollTop
is at least 100
.
*
* JavaScript Info:
* @property threshold
* @type Number
* @behavior AppBox
*/
@JsProperty double getThreshold();
/**
* Allows to set a scrollTop
threshold. When greater than 0, thresholdTriggered
is true only when the scroll target’s scrollTop
has reached this value.
* For example, if threshold = 100
, thresholdTriggered
is true when the scrollTop
is at least 100
.
*
* JavaScript Info:
* @property threshold
* @type Number
* @behavior AppBox
*/
@JsProperty void setThreshold(double value);
/**
* True if the scrollTop
threshold (set in scrollTopThreshold
) has
been reached.
*
* JavaScript Info:
* @property thresholdTriggered
* @type Boolean
* @behavior AppBox
*/
@JsProperty boolean getThresholdTriggered();
/**
* True if the scrollTop
threshold (set in scrollTopThreshold
) has
been reached.
*
* JavaScript Info:
* @property thresholdTriggered
* @type Boolean
* @behavior AppBox
*/
@JsProperty void setThresholdTriggered(boolean value);
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
@JsProperty Element getScrollTarget();
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
@JsProperty void setScrollTarget(Element value);
/**
* An object that configurates the effects installed via the effects
property. e.g.
* element.effectsConfig = {
* "blend-background": {
* "startsAt": 0.5
* }
* };
*
* Every effect has at least two config properties: startsAt
and endsAt
.
These properties indicate when the event should start and end respectively
and relative to the overall element progress. So for example, if blend-background
starts at 0.5
, the effect will only start once the current element reaches 0.5
of its progress. In this context, the progress is a value in the range of [0, 1]
that indicates where this element is on the screen relative to the viewport.
*
* JavaScript Info:
* @property effectsConfig
* @type Object
* @behavior AppBox
*/
@JsProperty JavaScriptObject getEffectsConfig();
/**
* An object that configurates the effects installed via the effects
property. e.g.
* element.effectsConfig = {
* "blend-background": {
* "startsAt": 0.5
* }
* };
*
* Every effect has at least two config properties: startsAt
and endsAt
.
These properties indicate when the event should start and end respectively
and relative to the overall element progress. So for example, if blend-background
starts at 0.5
, the effect will only start once the current element reaches 0.5
of its progress. In this context, the progress is a value in the range of [0, 1]
that indicates where this element is on the screen relative to the viewport.
*
* JavaScript Info:
* @property effectsConfig
* @type Object
* @behavior AppBox
*/
@JsProperty void setEffectsConfig(JavaScriptObject value);
/**
* A space-separated list of the effects names that will be triggered when the user scrolls.
e.g. waterfall parallax-background
installs the waterfall
and parallax-background
.
*
* JavaScript Info:
* @property effects
* @type String
* @behavior AppBox
*/
@JsProperty String getEffects();
/**
* A space-separated list of the effects names that will be triggered when the user scrolls.
e.g. waterfall parallax-background
installs the waterfall
and parallax-background
.
*
* JavaScript Info:
* @property effects
* @type String
* @behavior AppBox
*/
@JsProperty void setEffects(String value);
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
void assignParentResizable(Object parentResizable);
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
void stopResizeNotificationsFor(Object target);
/**
* Returns true if there’s content below the current element.
*
* JavaScript Info:
* @method isContentBelow
*
* @return {boolean}
*/
boolean isContentBelow();
/**
* Returns true if the current element is on the screen.
That is, visible in the current viewport.
*
* JavaScript Info:
* @method isOnScreen
*
* @return {boolean}
*/
boolean isOnScreen();
/**
* Resets the layout. If you changed the size of app-header via CSS
you can notify the changes by either firing the iron-resize
event
or calling resetLayout
directly.
*
* JavaScript Info:
* @method resetLayout
*
*
*/
void resetLayout();
/**
* Returns an object containing the progress value of the scroll effects
and the top position of the header.
*
* JavaScript Info:
* @method getScrollState
*
* @return {JavaScriptObject}
*/
JavaScriptObject getScrollState();
/**
* Returns true if the current header will condense based on the size of the header
and the consenses
property.
*
* JavaScript Info:
* @method willCondense
*
* @return {boolean}
*/
boolean willCondense();
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
void notifyResize();
/**
* Creates an effect object from an effect’s name that can be used to run
effects programmatically.
*
* JavaScript Info:
* @method createEffect
* @param {string} effectName
* @param {Object=} effectConfig
* @behavior AppBox
* @return {JavaScriptObject}
*/
JavaScriptObject createEffect(String effectName, JavaScriptObject effectConfig);
/**
* Scrolls the content to a particular place.
*
* JavaScript Info:
* @method scroll
* @param {number} left
* @param {number} top
* @behavior IronScrollThreshold
*
*/
void scroll(double left, double top);
/**
* Enables or disables the scroll event listener.
*
* JavaScript Info:
* @method toggleScrollListener
* @param {boolean} yes
* @behavior IronScrollThreshold
*
*/
void toggleScrollListener(boolean yes);
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
boolean resizerShouldNotify(JavaScriptObject element);
}