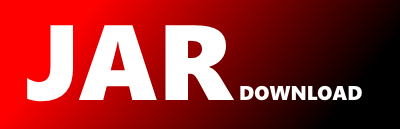
com.vaadin.polymer.app.AppLocationElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-route project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* app-location
is an element that provides synchronization between the
browser location bar and the state of an app. When created, app-location
elements will automatically watch the global location for changes. As changes
occur, app-location
produces and updates an object called route
. This
route
object is suitable for passing into a app-route
, and other similar
elements.
* An example of the public API of a route object that describes the URL
https://elements.polymer-project.org/elements/app-location
:
* {
* prefix: '',
* path: '/elements/app-location'
* }
*
*
*
Example Usage:
* <app-location route="{{route}}"></app-location>
* <app-route route="{{route}}" pattern="/:page" data="{{data}}"></app-route>
*
*
*
As you can see above, the app-location
element produces a route
and that
property is then bound into the app-route
element. The bindings are two-
directional, so when changes to the route
object occur within app-route
,
they automatically reflect back to the global location.
* Hashes vs Paths
* By default app-location
routes using the pathname portion of the URL. This has
broad browser support but it does require cooperation of the backend server. An
app-location
can be configured to use the hash part of a URL instead using
the use-hash-as-path
attribute, like so:
* <app-location route="{{route}}" use-hash-as-path></app-location>
*
*
*
Integrating with other routing code
* There is no standard event that is fired when window.location is modified.
app-location
fires a location-changed
event on window
when it updates the
location. It also listens for that same event, and re-reads the URL when it’s
fired. This makes it very easy to interop with other routing code.
* So for example if you want to navigate to /new_path
imperatively you could
call window.location.pushState
or window.location.replaceState
followed by
firing a location-changed
event on window
. i.e.
* window.history.pushState({}, null, '/new_path');
* window.dispatchEvent(new CustomEvent('location-changed'));
*
*
*
*/
@JsType(isNative=true)
public interface AppLocationElement extends HTMLElement {
@JsOverlay public static final String TAG = "app-location";
@JsOverlay public static final String SRC = "app-route/app-location.html";
/**
* A set of key/value pairs that are universally accessible to branches of
the route tree.
*
* JavaScript Info:
* @property queryParams
* @type ?Object
*
*/
@JsProperty JavaScriptObject getQueryParams();
/**
* A set of key/value pairs that are universally accessible to branches of
the route tree.
*
* JavaScript Info:
* @property queryParams
* @type ?Object
*
*/
@JsProperty void setQueryParams(JavaScriptObject value);
/**
* A model representing the deserialized path through the route tree, as
well as the current queryParams.
*
* JavaScript Info:
* @property route
* @type Object
*
*/
@JsProperty JavaScriptObject getRoute();
/**
* A model representing the deserialized path through the route tree, as
well as the current queryParams.
*
* JavaScript Info:
* @property route
* @type Object
*
*/
@JsProperty void setRoute(JavaScriptObject value);
/**
* A regexp that defines the set of URLs that should be considered part
of this web app.
* Clicking on a link that matches this regex won’t result in a full page
navigation, but will instead just update the URL state in place.
* This regexp is given everything after the origin in an absolute
URL. So to match just URLs that start with /search/ do:
url-space-regex=”^/search/“
*
* JavaScript Info:
* @property urlSpaceRegex
* @type (string|RegExp)
*
*/
@JsProperty Object getUrlSpaceRegex();
/**
* A regexp that defines the set of URLs that should be considered part
of this web app.
* Clicking on a link that matches this regex won’t result in a full page
navigation, but will instead just update the URL state in place.
* This regexp is given everything after the origin in an absolute
URL. So to match just URLs that start with /search/ do:
url-space-regex=”^/search/“
*
* JavaScript Info:
* @property urlSpaceRegex
* @type (string|RegExp)
*
*/
@JsProperty void setUrlSpaceRegex(Object value);
/**
* In many scenarios, it is convenient to treat the hash
as a stand-in
alternative to the path
. For example, if deploying an app to a static
web server (e.g., Github Pages) - where one does not have control over
server-side routing - it is usually a better experience to use the hash
to represent paths through one’s app.
* When this property is set to true, the hash
will be used in place of
* the path
for generating a route
.
*
* JavaScript Info:
* @property useHashAsPath
* @type Boolean
*
*/
@JsProperty boolean getUseHashAsPath();
/**
* In many scenarios, it is convenient to treat the hash
as a stand-in
alternative to the path
. For example, if deploying an app to a static
web server (e.g., Github Pages) - where one does not have control over
server-side routing - it is usually a better experience to use the hash
to represent paths through one’s app.
* When this property is set to true, the hash
will be used in place of
* the path
for generating a route
.
*
* JavaScript Info:
* @property useHashAsPath
* @type Boolean
*
*/
@JsProperty void setUseHashAsPath(boolean value);
/**
* The route path, which will be either the hash or the path, depending
on useHashAsPath.
*
* JavaScript Info:
* @property path
* @type String
*
*/
@JsProperty String getPath();
/**
* The route path, which will be either the hash or the path, depending
on useHashAsPath.
*
* JavaScript Info:
* @property path
* @type String
*
*/
@JsProperty void setPath(String value);
}