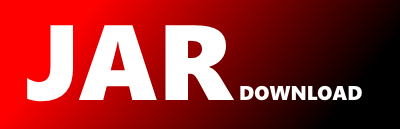
com.vaadin.polymer.app.AppPouchdbDocumentElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-pouchdb project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* app-pouchdb-document
is an implementation of Polymer.AppStorageBehavior
for reading and writing to individual PouchDB documents.
* In order to refer to a PouchDB document, provide the name of the database
(both local and remote databases are supported) and the ID of the document.
* For example:
* <app-pouchdb-document
* db-name="cats"
* doc-id="parsnip">
* </app-pouchdb-document>
*
* In the above example, a PouchDB instance will be created to connect to the
local database named “cats”. Then it will check to see if a document with the
ID “parsnip” exists. If it does, the data
property of the document will be
set to the value of the document. If it does not, then any subsequent
assignments to the data
property will cause a document with ID “parsnip” to
be created.
* Here is an example of a simple form that can be used to read and write to a
PouchDB document:
* <app-pouchdb-document
* db-name="cats"
* doc-id="parsnip"
* data="{{cat}}">
* </app-pouchdb-document>
* <input
* is="iron-input"
* bind-value="{{cat.name}}">
* </input>
*
*/
@JsType(isNative=true)
public interface AppPouchdbDocumentElement extends HTMLElement {
@JsOverlay public static final String TAG = "app-pouchdb-document";
@JsOverlay public static final String SRC = "app-pouchdb/app-pouchdb-document.html";
/**
* If true, all attempts to “put” or “post” values into the database
will be automatically structured as an “upsert”, where documents are
updated if already available, or created if not.
*
* JavaScript Info:
* @property upsert
* @type Boolean
*
*/
@JsProperty boolean getUpsert();
/**
* If true, all attempts to “put” or “post” values into the database
will be automatically structured as an “upsert”, where documents are
updated if already available, or created if not.
*
* JavaScript Info:
* @property upsert
* @type Boolean
*
*/
@JsProperty void setUpsert(boolean value);
/**
* The number of document revisions to keep track of. The default value
(0) indicates no limit.
*
* JavaScript Info:
* @property revsLimit
* @type Number
* @behavior AppPouchdbQuery
*/
@JsProperty double getRevsLimit();
/**
* The number of document revisions to keep track of. The default value
(0) indicates no limit.
*
* JavaScript Info:
* @property revsLimit
* @type Number
* @behavior AppPouchdbQuery
*/
@JsProperty void setRevsLimit(double value);
/**
* A reference to the PouchDB database instance that has been created.
*
* JavaScript Info:
* @property db
* @type Object
* @behavior AppPouchdbQuery
*/
@JsProperty JavaScriptObject getDb();
/**
* A reference to the PouchDB database instance that has been created.
*
* JavaScript Info:
* @property db
* @type Object
* @behavior AppPouchdbQuery
*/
@JsProperty void setDb(JavaScriptObject value);
/**
* Override this getter to define the default value to use when
there’s no data stored.
*
* JavaScript Info:
* @property zeroValue
* @type *
* @behavior AppPouchdbDocument
*/
@JsProperty JavaScriptObject getZeroValue();
/**
* Override this getter to define the default value to use when
there’s no data stored.
*
* JavaScript Info:
* @property zeroValue
* @type *
* @behavior AppPouchdbDocument
*/
@JsProperty void setZeroValue(JavaScriptObject value);
/**
* The data to synchronize.
*
* JavaScript Info:
* @property data
* @type Object
* @behavior AppPouchdbDocument
*/
@JsProperty JavaScriptObject getData();
/**
* The data to synchronize.
*
* JavaScript Info:
* @property data
* @type Object
* @behavior AppPouchdbDocument
*/
@JsProperty void setData(JavaScriptObject value);
/**
* A changes event emitter. Notifies of changes to the PouchDB document
referred to by docId
, if a docId
has been provided.
*
* JavaScript Info:
* @property changes
* @type Object
*
*/
@JsProperty JavaScriptObject getChanges();
/**
* A changes event emitter. Notifies of changes to the PouchDB document
referred to by docId
, if a docId
has been provided.
*
* JavaScript Info:
* @property changes
* @type Object
*
*/
@JsProperty void setChanges(JavaScriptObject value);
/**
* Override this getter to return true if the value has never been
persisted to storage.
*
* JavaScript Info:
* @property isNew
* @type boolean
* @behavior AppPouchdbDocument
*/
@JsProperty boolean getIsNew();
/**
* Override this getter to return true if the value has never been
persisted to storage.
*
* JavaScript Info:
* @property isNew
* @type boolean
* @behavior AppPouchdbDocument
*/
@JsProperty void setIsNew(boolean value);
/**
* When true, will perform detailed logging.
*
* JavaScript Info:
* @property log
* @type Boolean
* @behavior AppPouchdbDocument
*/
@JsProperty boolean getLog();
/**
* When true, will perform detailed logging.
*
* JavaScript Info:
* @property log
* @type Boolean
* @behavior AppPouchdbDocument
*/
@JsProperty void setLog(boolean value);
/**
* If this is true transactions will happen one after the other,
never in parallel.
* Specifically, no transaction will begin until every previously
enqueued transaction by this element has completed.
* If it is false, new transactions will be executed as they are
received.
*
* JavaScript Info:
* @property sequentialTransactions
* @type Boolean
* @behavior AppPouchdbDocument
*/
@JsProperty boolean getSequentialTransactions();
/**
* If this is true transactions will happen one after the other,
never in parallel.
* Specifically, no transaction will begin until every previously
enqueued transaction by this element has completed.
* If it is false, new transactions will be executed as they are
received.
*
* JavaScript Info:
* @property sequentialTransactions
* @type Boolean
* @behavior AppPouchdbDocument
*/
@JsProperty void setSequentialTransactions(boolean value);
/**
* A promise that will resolve once all queued transactions
have completed.
* This field is updated as new transactions are enqueued, so it will
only wait for transactions which were enqueued when the field
was accessed.
* This promise never rejects.
*
* JavaScript Info:
* @property transactionsComplete
* @type Promise
* @behavior AppPouchdbDocument
*/
@JsProperty JavaScriptObject getTransactionsComplete();
/**
* A promise that will resolve once all queued transactions
have completed.
* This field is updated as new transactions are enqueued, so it will
only wait for transactions which were enqueued when the field
was accessed.
* This promise never rejects.
*
* JavaScript Info:
* @property transactionsComplete
* @type Promise
* @behavior AppPouchdbDocument
*/
@JsProperty void setTransactionsComplete(JavaScriptObject value);
/**
* If desired, assign a function that implements a conflict resolution
strategy. This conflict resolution strategy will take precedence when
a conflict occurs, and will prevent conflict notification events from
being fired.
* Consider using an app-pouchdb-conflict-resolver
element instead for
a more declarative experience!
*
* JavaScript Info:
* @property resolveConflict
* @type Function
*
*/
@JsProperty Function getResolveConflict();
/**
* If desired, assign a function that implements a conflict resolution
strategy. This conflict resolution strategy will take precedence when
a conflict occurs, and will prevent conflict notification events from
being fired.
* Consider using an app-pouchdb-conflict-resolver
element instead for
a more declarative experience!
*
* JavaScript Info:
* @property resolveConflict
* @type Function
*
*/
@JsProperty void setResolveConflict(Function value);
/**
* The current _rev (revision) of the PouchDB document that this
element’s data refers to, if the document is not new.
*
* JavaScript Info:
* @property rev
* @type String
*
*/
@JsProperty String getRev();
/**
* The current _rev (revision) of the PouchDB document that this
element’s data refers to, if the document is not new.
*
* JavaScript Info:
* @property rev
* @type String
*
*/
@JsProperty void setRev(String value);
/**
* The PouchDB adapter to use. For more information on PouchDB adapters,
please refer to the PouchDB documentation
here.
*
* JavaScript Info:
* @property adapter
* @type String
* @behavior AppPouchdbQuery
*/
@JsProperty String getAdapter();
/**
* The PouchDB adapter to use. For more information on PouchDB adapters,
please refer to the PouchDB documentation
here.
*
* JavaScript Info:
* @property adapter
* @type String
* @behavior AppPouchdbQuery
*/
@JsProperty void setAdapter(String value);
/**
* The name of the database. This can be either a local database (such
as “cats”), or a remote one (e.g., “https://example.com:5678/cats“).
*
* JavaScript Info:
* @property dbName
* @type String
* @behavior AppPouchdbQuery
*/
@JsProperty String getDbName();
/**
* The name of the database. This can be either a local database (such
as “cats”), or a remote one (e.g., “https://example.com:5678/cats“).
*
* JavaScript Info:
* @property dbName
* @type String
* @behavior AppPouchdbQuery
*/
@JsProperty void setDbName(String value);
/**
* The value of the _id (Pouch/Couch unique identifier) of the PouchDB
document that this element’s data should refer to.
*
* JavaScript Info:
* @property docId
* @type String
*
*/
@JsProperty String getDocId();
/**
* The value of the _id (Pouch/Couch unique identifier) of the PouchDB
document that this element’s data should refer to.
*
* JavaScript Info:
* @property docId
* @type String
*
*/
@JsProperty void setDocId(String value);
/**
*
*
* JavaScript Info:
* @method setStoredValue
* @param {} storagePath
* @param {} value
*
*
*/
void setStoredValue(Object storagePath, Object value);
/**
*
*
* JavaScript Info:
* @method getStoredValue
* @param {} storagePath
*
*
*/
void getStoredValue(Object storagePath);
/**
* A convenience method. Returns true iff value is null, undefined,
an empty array, or an object with no keys.
*
* JavaScript Info:
* @method valueIsEmpty
* @param {} value
* @behavior AppPouchdbDocument
*
*/
void valueIsEmpty(Object value);
/**
*
*
* JavaScript Info:
* @method reset
*
*
*/
void reset();
/**
* Perform the initial sync between storage and memory. This method
is called automatically while the element is being initialized.
Implementations may override it.
* If an implementation intends to call this method, it should instead
call _initializeStoredValue, which provides reentrancy protection.
*
* JavaScript Info:
* @method initializeStoredValue
* @behavior AppPouchdbDocument
* @return {JavaScriptObject}
*/
JavaScriptObject initializeStoredValue();
/**
* Remove the data from storage.
*
* JavaScript Info:
* @method destroy
* @behavior AppPouchdbDocument
* @return {JavaScriptObject}
*/
JavaScriptObject destroy();
/**
*
*
* JavaScript Info:
* @method save
*
*
*/
void save();
/**
* Override this method.
* If the data value represented by this storage instance is new, this
method generates an attempt to write the value to storage.
*
* JavaScript Info:
* @method saveValue
* @param {*} args
* @behavior AppPouchdbDocument
* @return {JavaScriptObject}
*/
JavaScriptObject saveValue(JavaScriptObject args);
/**
* Override this method to implement creating and updating
stored values.
*
* JavaScript Info:
* @method setStoredValue
* @param {string} storagePath
* @param {*} value
* @behavior AppPouchdbDocument
* @return {JavaScriptObject}
*/
JavaScriptObject setStoredValue(String storagePath, JavaScriptObject value);
/**
* Maps a storage path to the corresponding Polymer databinding path.
Override to define a custom mapping.
* The inverse of memoryPathToStoragePath.
*
* JavaScript Info:
* @method storagePathToMemoryPath
* @param {string} path
* @behavior AppPouchdbDocument
* @return {String}
*/
String storagePathToMemoryPath(String path);
/**
* Maps a Polymer databinding path to the corresponding path in the
storage system. Override to define a custom mapping.
* The inverse of storagePathToMemoryPath.
*
* JavaScript Info:
* @method memoryPathToStoragePath
* @param {string} path
* @behavior AppPouchdbDocument
* @return {String}
*/
String memoryPathToStoragePath(String path);
/**
* Enables performing transformations on the in-memory representation of
storage without activating observers that will cause those
transformations to be re-applied to the storage backend. This is useful
for preventing redundant (or cyclical) application of transformations.
*
* JavaScript Info:
* @method syncToMemory
* @param {Function} operation
* @behavior AppPouchdbDocument
*
*/
void syncToMemory(Function operation);
}