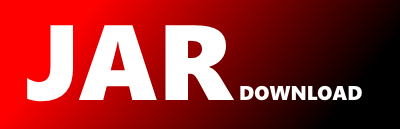
com.vaadin.polymer.app.AppRouteConverterElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-route project by The Polymer Authors
* that is licensed with http://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* app-route-converter
provides a means to convert a path and query
parameters into a route object and vice versa. This produced route object
is to be fed into route-consuming elements such as app-route
.
*
* n.b. This element is intended to be a primitive of the routing system and for
creating bespoke routing solutions from scratch. To simply include routing in
an app, please refer to app-location
and app-route.
*
* An example of a route object that describes
https://elements.polymer-project.org/elements/app-route-converter?foo=bar&baz=qux
and should be passed to other app-route
elements:
* {
* prefix: '',
* path: '/elements/app-route-converter',
* __queryParams: {
* foo: 'bar',
* baz: 'qux'
* }
* }
*
*
*
__queryParams
is private to discourage directly data-binding to it. This is so
that routing elements like app-route
can intermediate changes to the query
params and choose whether to propagate them upstream or not. app-route
for
example will not propagate changes to its queryParams
property if it is not
currently active. A public queryParams object will also be produced in which you
should perform data-binding operations.
* Example Usage:
* <iron-location path="{{path}}" query="{{query}}"></iron-location>
* <iron-query-params
* params-string="{{query}}"
* params-object="{{queryParams}}">
* </iron-query-params>
* <app-route-converter
* path="{{path}}"
* query-params="{{queryParams}}"
* route="{{route}}">
* </app-route-converter>
* <app-route route='{{route}}' pattern='/:page' data='{{data}}'>
* </app-route>
*
*
*
This is a simplified implementation of the app-location
element. Here the
iron-location
produces a path and a query, the iron-query-params
consumes
the query and produces a queryParams object, and the app-route-converter
consumes the path and the query params and converts it into a route which is in
turn is consumed by the app-route
.
*/
@JsType(isNative=true)
public interface AppRouteConverterElement extends HTMLElement {
@JsOverlay public static final String TAG = "app-route-converter";
@JsOverlay public static final String SRC = "app-route/app-route-converter.html";
/**
* A set of key/value pairs that are universally accessible to branches of
the route tree.
*
* JavaScript Info:
* @property queryParams
* @type ?Object
*
*/
@JsProperty JavaScriptObject getQueryParams();
/**
* A set of key/value pairs that are universally accessible to branches of
the route tree.
*
* JavaScript Info:
* @property queryParams
* @type ?Object
*
*/
@JsProperty void setQueryParams(JavaScriptObject value);
/**
* A model representing the deserialized path through the route tree, as
well as the current queryParams.
* A route object is the kernel of the routing system. It is intended to
be fed into consuming elements such as app-route
.
*
* JavaScript Info:
* @property route
* @type ?Object
*
*/
@JsProperty JavaScriptObject getRoute();
/**
* A model representing the deserialized path through the route tree, as
well as the current queryParams.
* A route object is the kernel of the routing system. It is intended to
be fed into consuming elements such as app-route
.
*
* JavaScript Info:
* @property route
* @type ?Object
*
*/
@JsProperty void setRoute(JavaScriptObject value);
/**
* The serialized path through the route tree. This corresponds to the
window.location.pathname
value, and will update to reflect changes
to that value.
*
* JavaScript Info:
* @property path
* @type String
*
*/
@JsProperty String getPath();
/**
* The serialized path through the route tree. This corresponds to the
window.location.pathname
value, and will update to reflect changes
to that value.
*
* JavaScript Info:
* @property path
* @type String
*
*/
@JsProperty void setPath(String value);
}