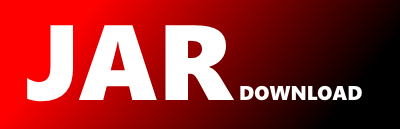
com.vaadin.polymer.app.AppScrollEffectsBehavior Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-layout project by The Polymer Authors
* that is licensed with https://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* Polymer.AppScrollEffectsBehavior
provides an interface that allows an element to use scrolls effects.
* Importing the app-layout effects
* app-layout provides a set of scroll effects that can be used by explicitly importing
app-scroll-effects.html
:
* <link rel="import" href="/bower_components/app-layout/app-scroll-effects/app-scroll-effects.html">
*
* The scroll effects can also be used by individually importing
app-layout/app-scroll-effects/effects/[effectName].html
. For example:
* <link rel="import" href="/bower_components/app-layout/app-scroll-effects/effects/waterfall.html">
*
* Consuming effects
* Effects can be consumed via the effects
property. For example:
* <app-header effects="waterfall"></app-header>
*
* Creating scroll effects
* You may want to create a custom scroll effect if you need to modify the CSS of an element
based on the scroll position.
* A scroll effect definition is an object with setUp()
, tearDown()
and run()
functions.
* To register the effect, you can use Polymer.AppLayout.registerEffect(effectName, effectDef)
For example, let’s define an effect that resizes the header’s logo:
* Polymer.AppLayout.registerEffect('resizable-logo', {
* setUp: function(config) {
* // the effect's config is passed to the setUp.
* this._fxResizeLogo = { logo: Polymer.dom(this).querySelector('[logo]') };
* },
*
* run: function(progress) {
* // the progress of the effect
* this.transform('scale3d(' + progress + ', '+ progress +', 1)', this._fxResizeLogo.logo);
* },
*
* tearDown: function() {
* // clean up and reset of states
* delete this._fxResizeLogo;
* }
* });
*
* Now, you can consume the effect:
* <app-header id="appHeader" effects="resizable-logo">
* <img logo src="logo.svg">
* </app-header>
*
* Imperative API
* var logoEffect = appHeader.createEffect('resizable-logo', effectConfig);
* // run the effect: logoEffect.run(progress);
* // tear down the effect: logoEffect.tearDown();
*
* Configuring effects
* For effects installed via the effects
property, their configuration can be set
via the effectsConfig
property. For example:
* <app-header effects="waterfall"
* effects-config='{"waterfall": {"startsAt": 0, "endsAt": 0.5}}'>
* </app-header>
*
* All effects have a startsAt
and endsAt
config property. They specify at what
point the effect should start and end. This value goes from 0 to 1 inclusive.
*/
@JsType(isNative=true)
public interface AppScrollEffectsBehavior {
@JsOverlay public static final String NAME = "Polymer.AppScrollEffectsBehavior";
@JsOverlay public static final String SRC = "app-layout/app-layout.html";
/**
* Disables CSS transitions and scroll effects on the element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior AppBox
*/
@JsProperty boolean getDisabled();
/**
* Disables CSS transitions and scroll effects on the element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior AppBox
*/
@JsProperty void setDisabled(boolean value);
/**
* An object that configurates the effects installed via the effects
property. e.g.
* element.effectsConfig = {
* "blend-background": {
* "startsAt": 0.5
* }
* };
*
* Every effect has at least two config properties: startsAt
and endsAt
.
These properties indicate when the event should start and end respectively
and relative to the overall element progress. So for example, if blend-background
starts at 0.5
, the effect will only start once the current element reaches 0.5
of its progress. In this context, the progress is a value in the range of [0, 1]
that indicates where this element is on the screen relative to the viewport.
*
* JavaScript Info:
* @property effectsConfig
* @type Object
* @behavior AppBox
*/
@JsProperty JavaScriptObject getEffectsConfig();
/**
* An object that configurates the effects installed via the effects
property. e.g.
* element.effectsConfig = {
* "blend-background": {
* "startsAt": 0.5
* }
* };
*
* Every effect has at least two config properties: startsAt
and endsAt
.
These properties indicate when the event should start and end respectively
and relative to the overall element progress. So for example, if blend-background
starts at 0.5
, the effect will only start once the current element reaches 0.5
of its progress. In this context, the progress is a value in the range of [0, 1]
that indicates where this element is on the screen relative to the viewport.
*
* JavaScript Info:
* @property effectsConfig
* @type Object
* @behavior AppBox
*/
@JsProperty void setEffectsConfig(JavaScriptObject value);
/**
* Allows to set a scrollTop
threshold. When greater than 0, thresholdTriggered
is true only when the scroll target’s scrollTop
has reached this value.
* For example, if threshold = 100
, thresholdTriggered
is true when the scrollTop
is at least 100
.
*
* JavaScript Info:
* @property threshold
* @type Number
* @behavior AppBox
*/
@JsProperty double getThreshold();
/**
* Allows to set a scrollTop
threshold. When greater than 0, thresholdTriggered
is true only when the scroll target’s scrollTop
has reached this value.
* For example, if threshold = 100
, thresholdTriggered
is true when the scrollTop
is at least 100
.
*
* JavaScript Info:
* @property threshold
* @type Number
* @behavior AppBox
*/
@JsProperty void setThreshold(double value);
/**
* True if the scrollTop
threshold (set in scrollTopThreshold
) has
been reached.
*
* JavaScript Info:
* @property thresholdTriggered
* @type Boolean
* @behavior AppBox
*/
@JsProperty boolean getThresholdTriggered();
/**
* True if the scrollTop
threshold (set in scrollTopThreshold
) has
been reached.
*
* JavaScript Info:
* @property thresholdTriggered
* @type Boolean
* @behavior AppBox
*/
@JsProperty void setThresholdTriggered(boolean value);
/**
* A space-separated list of the effects names that will be triggered when the user scrolls.
e.g. waterfall parallax-background
installs the waterfall
and parallax-background
.
*
* JavaScript Info:
* @property effects
* @type String
* @behavior AppBox
*/
@JsProperty String getEffects();
/**
* A space-separated list of the effects names that will be triggered when the user scrolls.
e.g. waterfall parallax-background
installs the waterfall
and parallax-background
.
*
* JavaScript Info:
* @property effects
* @type String
* @behavior AppBox
*/
@JsProperty void setEffects(String value);
/**
* Returns true if there’s content below the current element. This method
should be overridden by the consumer of this behavior.
*
* JavaScript Info:
* @method isContentBelow
* @behavior AppBox
* @return {boolean}
*/
boolean isContentBelow();
/**
* Returns true if the current element is on the screen.
That is, visible in the current viewport. This method should be
overridden by the consumer of this behavior.
*
* JavaScript Info:
* @method isOnScreen
* @behavior AppBox
* @return {boolean}
*/
boolean isOnScreen();
/**
* Creates an effect object from an effect’s name that can be used to run
effects programmatically.
*
* JavaScript Info:
* @method createEffect
* @param {string} effectName
* @param {Object=} effectConfig
* @behavior AppBox
* @return {JavaScriptObject}
*/
JavaScriptObject createEffect(String effectName, JavaScriptObject effectConfig);
}