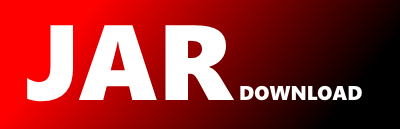
com.vaadin.polymer.app.AppScrollposControlElement Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-layout project by The Polymer Authors
* that is licensed with https://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app;
import com.vaadin.polymer.elemental.*;
import com.google.gwt.core.client.JavaScriptObject;
import com.google.gwt.core.client.JsArray;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsProperty;
import jsinterop.annotations.JsType;
/**
* app-scrollpos-control is a manager for saving and restoring the scroll position when multiple
pages are sharing the same document scroller.
* Example:
* <app-scrollpos-control selected="{{page}}"></app-scrollpos-control>
*
* <app-drawer-layout>
*
* <app-drawer>
* <paper-menu selected="{{page}}">
* <paper-item>Home</paper-item>
* <paper-item>About</paper-item>
* <paper-item>Schedule</paper-item>
* <paper-item>Account</paper-item>
* </paper-menu>
* </app-drawer>
*
* <div>
* <app-toolbar>
* <paper-icon-button icon="menu" drawer-toggle></paper-icon-button>
* </app-toolbar>
* <iron-pages selected="{{page}}">
* <sample-content size="100"></sample-content>
* <sample-content size="100"></sample-content>
* <sample-content size="100"></sample-content>
* <sample-content size="100"></sample-content>
* </iron-pages>
* </div>
*
* </app-drawer-layout>
*
*/
@JsType(isNative=true)
public interface AppScrollposControlElement extends HTMLElement {
@JsOverlay public static final String TAG = "app-scrollpos-control";
@JsOverlay public static final String SRC = "app-layout/app-layout.html";
/**
* Reset the scroll position to 0.
*
* JavaScript Info:
* @property reset
* @type Boolean
*
*/
@JsProperty boolean getReset();
/**
* Reset the scroll position to 0.
*
* JavaScript Info:
* @property reset
* @type Boolean
*
*/
@JsProperty void setReset(boolean value);
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
@JsProperty Element getScrollTarget();
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
@JsProperty void setScrollTarget(Element value);
/**
* The selected page.
*
* JavaScript Info:
* @property selected
* @type String
*
*/
@JsProperty String getSelected();
/**
* The selected page.
*
* JavaScript Info:
* @property selected
* @type String
*
*/
@JsProperty void setSelected(String value);
/**
* Enables or disables the scroll event listener.
*
* JavaScript Info:
* @method toggleScrollListener
* @param {boolean} yes
* @behavior IronScrollThreshold
*
*/
void toggleScrollListener(boolean yes);
/**
* Scrolls the content to a particular place.
*
* JavaScript Info:
* @method scroll
* @param {number} left
* @param {number} top
* @behavior IronScrollThreshold
*
*/
void scroll(double left, double top);
}