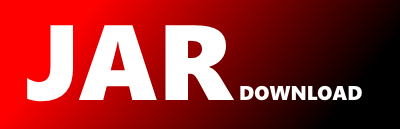
com.vaadin.polymer.app.widget.AppBox Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-layout project by The Polymer Authors
* that is licensed with https://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app.widget;
import com.vaadin.polymer.app.*;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* app-box is a container element that can have scroll effects - visual effects based on
scroll position. For example, the parallax effect can be used to move an image at a slower
rate than the foreground.
* <app-box style="height: 100px;" effects="parallax-background">
* <img background src="picture.png" style="width: 100%; height: 600px;">
* </app-box>
*
* Notice the background
attribute in the img
element; this attribute specifies that that
image is used as the background. By adding the background to the light dom, you can compose
backgrounds that can change dynamically. Alternatively, the mixin --app-box-background-front-layer
allows to style the background. For example:
* .parallaxAppBox {
* --app-box-background-front-layer: {
* background-image: url(picture.png);
* };
* }
*
* Finally, app-box can have content inside. For example:
* <app-box effects="parallax-background">
* <h2>Sub title</h2>
* </app-box>
*
* Importing the effects
* To use the scroll effects, you must explicitly import them in addition to app-box
:
* <link rel="import" href="/bower_components/app-layout/app-scroll-effects/app-scroll-effects.html">
*
* List of effects
* parallax-background
A simple parallax effect that vertically translates the backgrounds based on a fraction
of the scroll position. For example:
* app-header {
* --app-header-background-front-layer: {
* background-image: url(...);
* };
* }
*
* <app-header style="height: 300px;" effects="parallax-background">
* <app-toolbar>App name</app-toolbar>
* </app-header>
*
* The fraction determines how far the background moves relative to the scroll position.
This value can be assigned via the scalar
config value and it is typically a value
between 0 and 1 inclusive. If scalar=0
, the background doesn’t move away from the header.
* Styling
*
*
*
* Mixin
* Description
* Default
*
*
*
*
* --app-box-background-front-layer
* Applies to the front layer of the background
* {}
*
*
*
*/
public class AppBox extends PolymerWidget {
/**
* Default Constructor.
*/
public AppBox() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public AppBox(String html) {
super(AppBoxElement.TAG, AppBoxElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public AppBoxElement getPolymerElement() {
return (AppBoxElement) getElement();
}
/**
* Disables CSS transitions and scroll effects on the element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior AppBox
*/
public boolean getDisabled() {
return getPolymerElement().getDisabled();
}
/**
* Disables CSS transitions and scroll effects on the element.
*
* JavaScript Info:
* @property disabled
* @type Boolean
* @behavior AppBox
*/
public void setDisabled(boolean value) {
getPolymerElement().setDisabled(value);
}
/**
* Allows to set a scrollTop
threshold. When greater than 0, thresholdTriggered
is true only when the scroll target’s scrollTop
has reached this value.
* For example, if threshold = 100
, thresholdTriggered
is true when the scrollTop
is at least 100
.
*
* JavaScript Info:
* @property threshold
* @type Number
* @behavior AppBox
*/
public double getThreshold() {
return getPolymerElement().getThreshold();
}
/**
* Allows to set a scrollTop
threshold. When greater than 0, thresholdTriggered
is true only when the scroll target’s scrollTop
has reached this value.
* For example, if threshold = 100
, thresholdTriggered
is true when the scrollTop
is at least 100
.
*
* JavaScript Info:
* @property threshold
* @type Number
* @behavior AppBox
*/
public void setThreshold(double value) {
getPolymerElement().setThreshold(value);
}
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
public Element getScrollTarget() {
return getPolymerElement().getScrollTarget();
}
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @property scrollTarget
* @type Element
* @behavior IronScrollThreshold
*/
public void setScrollTarget(Element value) {
getPolymerElement().setScrollTarget(value);
}
/**
* An object that configurates the effects installed via the effects
property. e.g.
* element.effectsConfig = {
* "blend-background": {
* "startsAt": 0.5
* }
* };
*
* Every effect has at least two config properties: startsAt
and endsAt
.
These properties indicate when the event should start and end respectively
and relative to the overall element progress. So for example, if blend-background
starts at 0.5
, the effect will only start once the current element reaches 0.5
of its progress. In this context, the progress is a value in the range of [0, 1]
that indicates where this element is on the screen relative to the viewport.
*
* JavaScript Info:
* @property effectsConfig
* @type Object
* @behavior AppBox
*/
public JavaScriptObject getEffectsConfig() {
return getPolymerElement().getEffectsConfig();
}
/**
* An object that configurates the effects installed via the effects
property. e.g.
* element.effectsConfig = {
* "blend-background": {
* "startsAt": 0.5
* }
* };
*
* Every effect has at least two config properties: startsAt
and endsAt
.
These properties indicate when the event should start and end respectively
and relative to the overall element progress. So for example, if blend-background
starts at 0.5
, the effect will only start once the current element reaches 0.5
of its progress. In this context, the progress is a value in the range of [0, 1]
that indicates where this element is on the screen relative to the viewport.
*
* JavaScript Info:
* @property effectsConfig
* @type Object
* @behavior AppBox
*/
public void setEffectsConfig(JavaScriptObject value) {
getPolymerElement().setEffectsConfig(value);
}
/**
* True if the scrollTop
threshold (set in scrollTopThreshold
) has
been reached.
*
* JavaScript Info:
* @property thresholdTriggered
* @type Boolean
* @behavior AppBox
*/
public boolean getThresholdTriggered() {
return getPolymerElement().getThresholdTriggered();
}
/**
* True if the scrollTop
threshold (set in scrollTopThreshold
) has
been reached.
*
* JavaScript Info:
* @property thresholdTriggered
* @type Boolean
* @behavior AppBox
*/
public void setThresholdTriggered(boolean value) {
getPolymerElement().setThresholdTriggered(value);
}
/**
* A space-separated list of the effects names that will be triggered when the user scrolls.
e.g. waterfall parallax-background
installs the waterfall
and parallax-background
.
*
* JavaScript Info:
* @property effects
* @type String
* @behavior AppBox
*/
public String getEffects() {
return getPolymerElement().getEffects();
}
/**
* A space-separated list of the effects names that will be triggered when the user scrolls.
e.g. waterfall parallax-background
installs the waterfall
and parallax-background
.
*
* JavaScript Info:
* @property effects
* @type String
* @behavior AppBox
*/
public void setEffects(String value) {
getPolymerElement().setEffects(value);
}
// Needed in UIBinder
/**
* Allows to set a scrollTop
threshold. When greater than 0, thresholdTriggered
is true only when the scroll target’s scrollTop
has reached this value.
* For example, if threshold = 100
, thresholdTriggered
is true when the scrollTop
is at least 100
.
*
* JavaScript Info:
* @attribute threshold
* @behavior AppBox
*/
public void setThreshold(String value) {
Polymer.property(this.getPolymerElement(), "threshold", value);
}
// Needed in UIBinder
/**
* Specifies the element that will handle the scroll event
on the behalf of the current element. This is typically a reference to an element,
but there are a few more posibilities:
* Elements id
* <div id="scrollable-element" style="overflow: auto;">
* <x-element scroll-target="scrollable-element">
* <!-- Content-->
* </x-element>
* </div>
*
* In this case, the scrollTarget
will point to the outer div element.
* Document scrolling
* For document scrolling, you can use the reserved word document
:
* <x-element scroll-target="document">
* <!-- Content -->
* </x-element>
*
* Elements reference
* appHeader.scrollTarget = document.querySelector('#scrollable-element');
*
*
* JavaScript Info:
* @attribute scroll-target
* @behavior IronScrollThreshold
*/
public void setScrollTarget(String value) {
Polymer.property(this.getPolymerElement(), "scrollTarget", value);
}
// Needed in UIBinder
/**
* An object that configurates the effects installed via the effects
property. e.g.
* element.effectsConfig = {
* "blend-background": {
* "startsAt": 0.5
* }
* };
*
* Every effect has at least two config properties: startsAt
and endsAt
.
These properties indicate when the event should start and end respectively
and relative to the overall element progress. So for example, if blend-background
starts at 0.5
, the effect will only start once the current element reaches 0.5
of its progress. In this context, the progress is a value in the range of [0, 1]
that indicates where this element is on the screen relative to the viewport.
*
* JavaScript Info:
* @attribute effects-config
* @behavior AppBox
*/
public void setEffectsConfig(String value) {
Polymer.property(this.getPolymerElement(), "effectsConfig", value);
}
/**
* Used to assign the closest resizable ancestor to this resizable
if the ancestor detects a request for notifications.
*
* JavaScript Info:
* @method assignParentResizable
* @param {} parentResizable
* @behavior VaadinSplitLayout
*
*/
public void assignParentResizable(Object parentResizable) {
getPolymerElement().assignParentResizable(parentResizable);
}
/**
* Used to remove a resizable descendant from the list of descendants
that should be notified of a resize change.
*
* JavaScript Info:
* @method stopResizeNotificationsFor
* @param {} target
* @behavior VaadinSplitLayout
*
*/
public void stopResizeNotificationsFor(Object target) {
getPolymerElement().stopResizeNotificationsFor(target);
}
/**
* Returns true if this app-box is on the screen.
That is, visible in the current viewport.
*
* JavaScript Info:
* @method isOnScreen
*
* @return {boolean}
*/
public boolean isOnScreen() {
return getPolymerElement().isOnScreen();
}
/**
* Resets the layout. This method is automatically called when the element is attached to the DOM.
*
* JavaScript Info:
* @method resetLayout
*
*
*/
public void resetLayout() {
getPolymerElement().resetLayout();
}
/**
* Returns true if there’s content below the current element. This method
should be overridden by the consumer of this behavior.
*
* JavaScript Info:
* @method isContentBelow
* @behavior AppBox
* @return {boolean}
*/
public boolean isContentBelow() {
return getPolymerElement().isContentBelow();
}
/**
* Can be called to manually notify a resizable and its descendant
resizables of a resize change.
*
* JavaScript Info:
* @method notifyResize
* @behavior VaadinSplitLayout
*
*/
public void notifyResize() {
getPolymerElement().notifyResize();
}
/**
* Returns an object containing the progress value of the scroll effects.
*
* JavaScript Info:
* @method getScrollState
*
* @return {JavaScriptObject}
*/
public JavaScriptObject getScrollState() {
return getPolymerElement().getScrollState();
}
/**
* Creates an effect object from an effect’s name that can be used to run
effects programmatically.
*
* JavaScript Info:
* @method createEffect
* @param {string} effectName
* @param {Object=} effectConfig
* @behavior AppBox
* @return {JavaScriptObject}
*/
public JavaScriptObject createEffect(String effectName, JavaScriptObject effectConfig) {
return getPolymerElement().createEffect(effectName, effectConfig);
}
/**
* Scrolls the content to a particular place.
*
* JavaScript Info:
* @method scroll
* @param {number} left
* @param {number} top
* @behavior IronScrollThreshold
*
*/
public void scroll(double left, double top) {
getPolymerElement().scroll(left, top);
}
/**
* Enables or disables the scroll event listener.
*
* JavaScript Info:
* @method toggleScrollListener
* @param {boolean} yes
* @behavior IronScrollThreshold
*
*/
public void toggleScrollListener(boolean yes) {
getPolymerElement().toggleScrollListener(yes);
}
/**
* This method can be overridden to filter nested elements that should or
should not be notified by the current element. Return true if an element
should be notified, or false if it should not be notified.
*
* JavaScript Info:
* @method resizerShouldNotify
* @param {HTMLElement} element
* @behavior VaadinSplitLayout
* @return {boolean}
*/
public boolean resizerShouldNotify(JavaScriptObject element) {
return getPolymerElement().resizerShouldNotify(element);
}
}