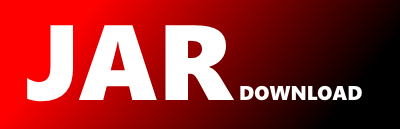
com.vaadin.polymer.app.widget.AppDrawer Maven / Gradle / Ivy
/*
* This code was generated with Vaadin Web Component GWT API Generator,
* from app-layout project by The Polymer Authors
* that is licensed with https://polymer.github.io/LICENSE.txt license.
*/
package com.vaadin.polymer.app.widget;
import com.vaadin.polymer.app.*;
import com.vaadin.polymer.app.widget.event.AppDrawerAttachedEvent;
import com.vaadin.polymer.app.widget.event.AppDrawerAttachedEventHandler;
import com.vaadin.polymer.app.widget.event.AppDrawerResetLayoutEvent;
import com.vaadin.polymer.app.widget.event.AppDrawerResetLayoutEventHandler;
import com.vaadin.polymer.app.widget.event.AppDrawerTransitionedEvent;
import com.vaadin.polymer.app.widget.event.AppDrawerTransitionedEventHandler;
import com.vaadin.polymer.*;
import com.vaadin.polymer.elemental.*;
import com.vaadin.polymer.PolymerWidget;
import com.google.gwt.core.client.JsArray;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.core.client.JavaScriptObject;
/**
* app-drawer is a navigation drawer that can slide in from the left or right.
* Example:
* Align the drawer at the start, which is left in LTR layouts (default):
* <app-drawer opened></app-drawer>
*
* Align the drawer at the end:
* <app-drawer align="end" opened></app-drawer>
*
* To make the contents of the drawer scrollable, create a wrapper for the scroll
content, and apply height and overflow styles to it.
* <app-drawer>
* <div style="height: 100%; overflow: auto;"></div>
* </app-drawer>
*
* Styling
*
*
*
* Custom property
* Description
* Default
*
*
*
*
* --app-drawer-width
* Width of the drawer
* 256px
*
*
* --app-drawer-content-container
* Mixin for the drawer content container
* {}
*
*
* --app-drawer-scrim-background
* Background for the scrim
* rgba(0, 0, 0, 0.5)
*
*
*
*/
public class AppDrawer extends PolymerWidget {
/**
* Default Constructor.
*/
public AppDrawer() {
this("");
}
/**
* Constructor used by UIBinder to create widgets with content.
*/
public AppDrawer(String html) {
super(AppDrawerElement.TAG, AppDrawerElement.SRC, html);
}
/**
* Gets a handle to the Polymer object's underlying DOM element.
*/
public AppDrawerElement getPolymerElement() {
return (AppDrawerElement) getElement();
}
/**
* The transition duration of the drawer in milliseconds.
*
* JavaScript Info:
* @property transitionDuration
* @type Number
*
*/
public double getTransitionDuration() {
return getPolymerElement().getTransitionDuration();
}
/**
* The transition duration of the drawer in milliseconds.
*
* JavaScript Info:
* @property transitionDuration
* @type Number
*
*/
public void setTransitionDuration(double value) {
getPolymerElement().setTransitionDuration(value);
}
/**
* Trap keyboard focus when the drawer is opened and not persistent.
*
* JavaScript Info:
* @property noFocusTrap
* @type Boolean
*
*/
public boolean getNoFocusTrap() {
return getPolymerElement().getNoFocusTrap();
}
/**
* Trap keyboard focus when the drawer is opened and not persistent.
*
* JavaScript Info:
* @property noFocusTrap
* @type Boolean
*
*/
public void setNoFocusTrap(boolean value) {
getPolymerElement().setNoFocusTrap(value);
}
/**
* Disables swiping on the drawer.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
public boolean getDisableSwipe() {
return getPolymerElement().getDisableSwipe();
}
/**
* Disables swiping on the drawer.
*
* JavaScript Info:
* @property disableSwipe
* @type Boolean
*
*/
public void setDisableSwipe(boolean value) {
getPolymerElement().setDisableSwipe(value);
}
/**
* Create an area at the edge of the screen to swipe open the drawer.
*
* JavaScript Info:
* @property swipeOpen
* @type Boolean
*
*/
public boolean getSwipeOpen() {
return getPolymerElement().getSwipeOpen();
}
/**
* Create an area at the edge of the screen to swipe open the drawer.
*
* JavaScript Info:
* @property swipeOpen
* @type Boolean
*
*/
public void setSwipeOpen(boolean value) {
getPolymerElement().setSwipeOpen(value);
}
/**
* The opened state of the drawer.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
public boolean getOpened() {
return getPolymerElement().getOpened();
}
/**
* The opened state of the drawer.
*
* JavaScript Info:
* @property opened
* @type Boolean
*
*/
public void setOpened(boolean value) {
getPolymerElement().setOpened(value);
}
/**
* The drawer does not have a scrim and cannot be swiped close.
*
* JavaScript Info:
* @property persistent
* @type Boolean
*
*/
public boolean getPersistent() {
return getPolymerElement().getPersistent();
}
/**
* The drawer does not have a scrim and cannot be swiped close.
*
* JavaScript Info:
* @property persistent
* @type Boolean
*
*/
public void setPersistent(boolean value) {
getPolymerElement().setPersistent(value);
}
/**
* The computed, read-only position of the drawer on the screen (‘left’ or ‘right’).
*
* JavaScript Info:
* @property position
* @type String
*
*/
public String getPosition() {
return getPolymerElement().getPosition();
}
/**
* The computed, read-only position of the drawer on the screen (‘left’ or ‘right’).
*
* JavaScript Info:
* @property position
* @type String
*
*/
public void setPosition(String value) {
getPolymerElement().setPosition(value);
}
/**
* The alignment of the drawer on the screen (‘left’, ‘right’, ‘start’ or ‘end’).
‘start’ computes to left and ‘end’ to right in LTR layout and vice versa in RTL
layout.
*
* JavaScript Info:
* @property align
* @type String
*
*/
public String getAlign() {
return getPolymerElement().getAlign();
}
/**
* The alignment of the drawer on the screen (‘left’, ‘right’, ‘start’ or ‘end’).
‘start’ computes to left and ‘end’ to right in LTR layout and vice versa in RTL
layout.
*
* JavaScript Info:
* @property align
* @type String
*
*/
public void setAlign(String value) {
getPolymerElement().setAlign(value);
}
// Needed in UIBinder
/**
* The transition duration of the drawer in milliseconds.
*
* JavaScript Info:
* @attribute transition-duration
*
*/
public void setTransitionDuration(String value) {
Polymer.property(this.getPolymerElement(), "transitionDuration", value);
}
/**
* Gets the width of the drawer.
*
* JavaScript Info:
* @method getWidth
*
* @return {double}
*/
public double getWidth() {
return getPolymerElement().getWidth();
}
/**
* Toggles the drawer open and close.
*
* JavaScript Info:
* @method toggle
*
*
*/
public void toggle() {
getPolymerElement().toggle();
}
/**
* Opens the drawer.
*
* JavaScript Info:
* @method open
*
*
*/
public void open() {
getPolymerElement().open();
}
/**
* Closes the drawer.
*
* JavaScript Info:
* @method close
*
*
*/
public void close() {
getPolymerElement().close();
}
/**
* Resets the layout. The event fired is used by app-drawer-layout to position the
content.
*
* JavaScript Info:
* @method resetLayout
*
*
*/
public void resetLayout() {
getPolymerElement().resetLayout();
}
/**
* Fired when the layout of app-drawer is attached.
*
* JavaScript Info:
* @event app-drawer-attached
*/
public HandlerRegistration addAppDrawerAttachedHandler(AppDrawerAttachedEventHandler handler) {
return addDomHandler(handler, AppDrawerAttachedEvent.TYPE);
}
/**
* Fired when the layout of app-drawer has changed.
*
* JavaScript Info:
* @event app-drawer-reset-layout
*/
public HandlerRegistration addAppDrawerResetLayoutHandler(AppDrawerResetLayoutEventHandler handler) {
return addDomHandler(handler, AppDrawerResetLayoutEvent.TYPE);
}
/**
* Fired when app-drawer has finished transitioning.
*
* JavaScript Info:
* @event app-drawer-transitioned
*/
public HandlerRegistration addAppDrawerTransitionedHandler(AppDrawerTransitionedEventHandler handler) {
return addDomHandler(handler, AppDrawerTransitionedEvent.TYPE);
}
}